Question
I need help creating a flowchart that outlines the method calls of my project. The flowchart should show the logic of the text menu and
I need help creating a flowchart that outlines the method calls of my project. The flowchart should show the logic of the text menu and what methods are called if a given option is selected. The logic in the methods IS NOT NEEDED.
CODE BELOW:
==================================================================================================
import java.util.Arrays;
import java.util.Scanner;
public class Project1 {
public static void main(String[] args) {
getAnswer();
} // end main
public static void getAnswer()
{
Scanner in = new Scanner(System.in);
boolean quit = false;
int menuItem;
System.out.println("Please enter a sentence of words seperated by spaces: ");
String sentence = in.nextLine();
String[] words = sentence.split(" ");
// handle user commands
do {
System.out.println(" " + "1. Display Word List Ordered A-Z");
System.out.println("2. Display Length of each Word in the List");
System.out.println("3. Display Word List Statistics");
System.out.println("4. Count Number of Words with Even/Odd Length");
System.out.println("5. Count Number of Words that have a Prime Length");
System.out.println("6. Enter New Word List");
System.out.println("7. Quit" + " ");
System.out.println(" " + "Choose a menu item or 7 to quit: " + " ");
menuItem = in.nextInt();
System.out.println();
switch (menuItem) {
case 1:
orderedWordList(words);
break;
case 2:
wordLength(sentence);
break;
case 3:
minMaxWordLength(sentence);
charCount(sentence);
avgWordLength(sentence);
nounCount(sentence);
mostFreqWord(sentence);
break;
case 4:
evenOddLength(sentence);
break;
case 5:
primeLength(sentence);
break;
case 6:
newWordlist();
break;
case 7:
quit = true;
}
} while (!quit);
System.out.println("Thank you for using this program, goodbye!");
in.close();
} // end getAnswers
// order each word in list from A-Z
public static void orderedWordList(String[] orderedWords)
{
Arrays.sort(orderedWords);
System.out.println("The sorted array is " + Arrays.toString(orderedWords));
} // end orderedWordList method
// get lengths of every word in string
public static void wordLength(String wordLengths)
{
// for each word, splitting at space, print word and length
for(String word : wordLengths.split(" "))
{
System.out.println(word + " length is " + word.length());
}
} // end orderedWordLength
// get min/max values in the string
public static void minMaxWordLength(String evalWord)
{
String word = "", small = "", large = "";
String[] words = new String[1000];
int length = 0;
evalWord = evalWord + " ";
for(int i = 0; i < evalWord.length(); i++)
{
if(evalWord.charAt(i) != ' ')
{
word = word + evalWord.charAt(i);
}
else {
words[length] = word;
length++;
word = "";
} // end if
} // end for
small = large = words[0];
//Determine smallest and largest word in the string
for(int k = 0; k < length; k++)
{
if(small.length() > words[k].length())
{
small = words[k];
} // end if
if(large.length() < words[k].length())
{
large = words[k];
} // end if
} // end for
System.out.println("Min word length: " + small.length());
System.out.println("Max word length: " + large.length());
} // end minMaxWordLength
// display total # of characters in string (no spaces)
public static void charCount(String charsInWords)
{
int countChar = 0;
for(int i = 0; i < charsInWords.length(); i++)
{
if(charsInWords.charAt(i) != ' ')
{
countChar++;
}
} // end for
System.out.println("Number of characters: " + countChar);
} // end charCount
// display average word length in string
public static void avgWordLength(String avgLength)
{
String[] words = avgLength.split(" ");
int count = words.length;
int sum = 0;
for(String word : words)
{
sum += word.length();
}
double average = (double)sum / count;
System.out.println("Average word length: " + String.format("%.2f", average));
} // end avgLength
// display number of nouns in string
public static void nounCount(String nounsWords)
{
int nounCount = 0;
for (String word : nounsWords.split(" ")) {
if (Character.isUpperCase(word.charAt(0)))
{
nounCount++;
}
}
System.out.println("Number of nouns: " + nounCount);
} // end nounCount
// display most occurring word in string
public static void mostFreqWord(String freqWord)
{
int max = 0;
int count = 0;
String[] splited = freqWord.split(" ");
Arrays.sort(splited);
String curr = splited[0];
String word = splited[0];
for(int i = 0; i < splited.length; i++)
{
if(splited[i].equalsIgnoreCase(curr))
{
count++;
}
else {
count = 1;
curr = splited[i];
}
if(max < count)
{
max = count;
word = splited[i];
}
} // end for
if(max == 1)
{
System.out.println("No Mode");
} else {
System.out.println("Most frequent word: " + word);
}
} // end mostFreqWord
// display even/odd word counts in string
public static void evenOddLength(String evenOddWords)
{
int posWordCount = 0;
int negWordCount = 0;
for (String word : evenOddWords.split(" "))
{
if(word.length() % 2 == 0)
{
posWordCount++;
}
else
{
negWordCount++;
} // end if else
} // end for loop
System.out.println("Number even: " + posWordCount);
System.out.println("Number odd: " + negWordCount);
} // end evenOddLength
static boolean isPrime(int n)
{
if (n <= 1)
{
return false;
}
for (int i = 2; i < n; i++)
{
if (n % i == 0)
{
return false;
}
} // end for
return true;
} // end boolean
// show count of prime words
public static void primeLength(String primeWord)
{
int primeCount = 0;
for (String word : primeWord.split(" "))
{
int length = word.length();
if (isPrime(length))
primeCount++;
} // end for
System.out.println("Number of Prime in list: " + primeCount);
} // end primeLength
// enter another sentence of strings to evaluate
public static String newWordlist()
{
Scanner in = new Scanner(System.in);
System.out.println("Please enter another sentence of words seperated by spaces: ");
String sentence = in.nextLine();
return sentence;
} // end newWordList
} // end class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
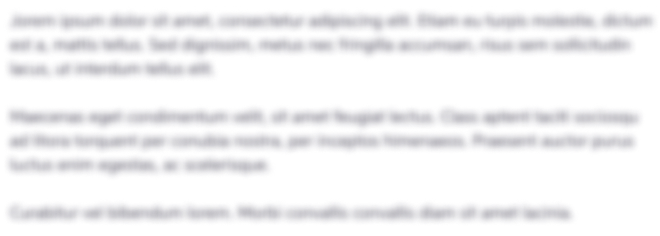
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started