Question
I need help making the print statements to display the answer. Define 7 functions. Each function will return a structured variable. V1= (5,-4, -3) V2=
I need help making the print statements to display the answer.
Define 7 functions. Each function will return a structured variable.
V1= (5,-4, -3)
V2= (1,2,6)
1) Add two vectors
2) Subtract two vectors
3) Multiply a vector by a scalar
4) Negate a vector (invert each value)
5) Take cross product of two vectors
6) Take dot project of two vectors (results in a scalar) (not a structured variable- just a scalar)
7) Normalize a vector (V2)
Submit both your code, and a screen capture of the results of each function.
#include
typedef struct{ int x; int y; int z; } value;
value V1;
V1.x = 5; V1.y = -4; V1.z = 3;
value V2;
V2.x = 1; V2.y = 2; V2.z = 6;
value add(value V1, value V2) { value V3; V3.x = V1.x+V2.x; V3.y = V1.y+V2.y; V3.z = V1.z+V2.z; return V3; } printf("Add two vectors: %f ",add);
value subtract(value V1, value V2) { value V3; V3.x = V1.x-V2.x; V3.y = V1.y-V2.y; V3.z = V1.z-V2.z; return V3; } value multiply(value V1, int V2) { V1.x = V1.x*V2; V1.y = V1.y*V2; V1.z = V1.z*V2; return V1; } value invert(value V1, int V2) { V1.x = V1.x*-1; V1.y = V1.y*-1; V1.z = V1.z*-1; return V1; } value cross(value V1, value V2) { value V3; V3.x = (V1.y*V2.z)-(V1.z*V2.y); V3.y = (V1.z*V2.x)-(V1.x*V2.z); V3.z = (V1.x*V2.y)-(V1.y*V2.x); return V3; } int dot(value V1, value V2) { int V3; V3=((V1.x*V2.x)+(V1.y*V2.y)+(V1.z*V2.z)); return V3; } double normalize(value V2) { double V3; V3=(V2.x*V2.x)+(V2.y*V2.y)+(V2.z*V2.z); return sqrt(V3); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
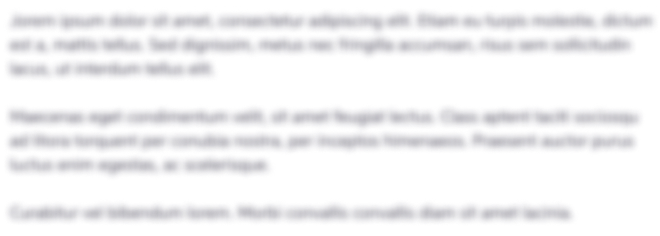
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started