Answered step by step
Verified Expert Solution
Question
1 Approved Answer
I need help to modify this money class using the instruction in the picture in java please! /** * A Money object models money as
I need help to modify this money class using the instruction in the picture in java please!
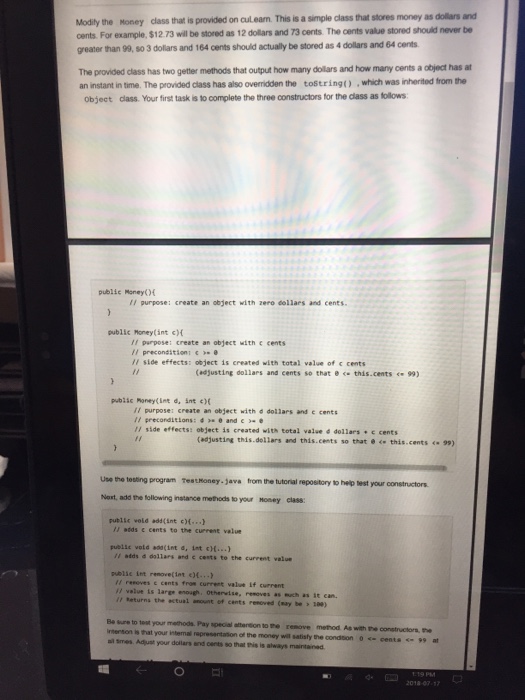
/**
* A Money object models money as dollars and cents
**/
public class Money{
/* instance attributes */
private int dollars = -1;
private byte cents = -1; // Once created we must ensure that 0
public Money(){
// purpose: create an object with zero dollars and cents.
}
public Money(int c){
// purpose: create an object with c cents
// precondition: c >= 0
// side effects: object is created with total value of c cents
// (adjusting dollars and cents so that 0
Modily the Money class that is provided on cu.eam This is a single class that stores monay as dollars and oents. For example, $12.73 will be stored as 12 dollars and 73 cents The cents value stored should never be greater than 99, so 3 dollars and 164 cents should actually be stored as 4 dolars and 64 cents The provided class has two getter methods that output how many dolars and how many cents a obiject has at an instant in time. The provided class has also overridden the tostring) ,which was inherited from the object class. Your first task is to complete the three constructors for the dlass as follows public Money) II purpose: create an object with zero dollars and cents public Money(int c) purpose: create an object with c cents /I preconestionc I/ side effects: object is created with total value of c cents adjusting dollars and cents so that e this.cents99) pubiic Money(it d, int c) If purpose: create an object with d dollars and c cents l/ preconditions: 4 and e I side effects: object is created with total value dollars.c cents (edjusting this.dellars and this.cents so that this.cents 99) Use the testing program TestRoney-java from the tutorial repository to help test your constructors Naxt, add the tollowing instance methods to your Honey class: public vold add(int c...) //.dds c cents to t? current value public vold alint , int cH...) 1 adds d allars and c cests to the current value nublic int renove(iat en...) i/ value is large enough Otherise, renoves as much as it e / teturns the sctusl anount of cents renoved (aay be > 109) ure to test your methods. Pay special attention to te remove method As with the constructors rtenton is that your internal representation of the money wil satisty the conditsion ocents al Smes. Adjust your dolars and cents so that Phis is always maintained 99 at 2018 07-17 }
public Money(int d, int c){
// purpose: create an object with d dollars and c cents
// preconditions: d >= 0 and c >= 0
// side effects: object is created with total value d dollars + c cents
// (adjusting this.dollars and this.cents so that 0
}
/** getter for dollars
*
* @return the current number of dollars this has
*/
public int getDollars(){ return this.dollars; }
/** getter for cents
*
* @return the current number of cents this has
*/
public int getCents(){ return this.cents; }
// Task #2 is to finish these three instance methods
// public void add(int c){...}
// adds c cents to the current value
// public void add(int d, int c){...}
// adds d dollars and c cents to the current value
// public int remove(int c){...}
// removes c cents from current value if current
// value is large enough. Otherwise, removes as much as it can.
// Returns the actual amount of cents removed (may be > 100)
/**
* Returns a String representation of the value of the current object.
*
* @return The value of the current object is returned as the
String
"$D.cc" * where D is the number of dollars and cc is the cents of the value. Uses the
format()
* method from the
String
class to ensure that the cents are displayed properly (2 spaces * with leading zeros if needed).
**/
@Override
public String toString(){
return "$" + String.format("%01d", dollars) + "." + String.format("%02d", cents);
}
}
Starting from here is the testMoney.java mentioned in the question
/** basic tester for Money class */
public class TestMoney{
public static void main(String[] args){
Money money;
String actual,expected;
System.out.print(" Money() test : ");
money = new Money();
actual = money.toString();
expected = "$0.00";
System.out.print("expected \"" + expected + "\" actual output \"" + actual + "\"");
if( actual.equals(expected) ){
System.out.println(" pass");
}else{
System.out.println(" fail");
}
System.out.print("Money(int, int) test : ");
money = new Money(0,0);
actual = money.toString();
expected = "$0.00";
System.out.print("expected \"" + expected + "\" actual output \"" + actual + "\"");
if( actual.equals(expected) ){
System.out.println(" pass");
}else{
System.out.println(" fail");
}
System.out.print("Money(int, int) test : ");
money = new Money(12,7);
actual = money.toString();
expected = "$12.07";
System.out.print("expected \"" + expected + "\" actual output \"" + actual + "\"");
if( actual.equals(expected) ){
System.out.println(" pass");
}else{
System.out.println(" fail");
}
System.out.print("Money(int, int) test : ");
money = new Money(3,4023);
actual = money.toString();
expected = "$43.23";
System.out.print("expected \"" + expected + "\" actual output \"" + actual + "\"");
if( actual.equals(expected) ){
System.out.println(" pass");
}else{
System.out.println(" fail");
}
System.out.print(" Money(int) test : ");
money = new Money(0);
actual = money.toString();
expected = "$0.00";
System.out.print("expected \"" + expected + "\" actual output \"" + actual + "\"");
if( actual.equals(expected) ){
System.out.println(" pass");
}else{
System.out.println(" fail");
}
System.out.print(" Money(int) test : ");
money = new Money(63);
actual = money.toString();
expected = "$0.63";
System.out.print("expected \"" + expected + "\" actual output \"" + actual + "\"");
if( actual.equals(expected) ){
System.out.println(" pass");
}else{
System.out.println(" fail");
}
System.out.print(" Money(int) test : ");
money = new Money(21387);
actual = money.toString();
expected = "$213.87";
System.out.print("expected \"" + expected + "\" actual output \"" + actual + "\"");
if( actual.equals(expected) ){
System.out.println(" pass");
}else{
System.out.println(" fail");
}
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
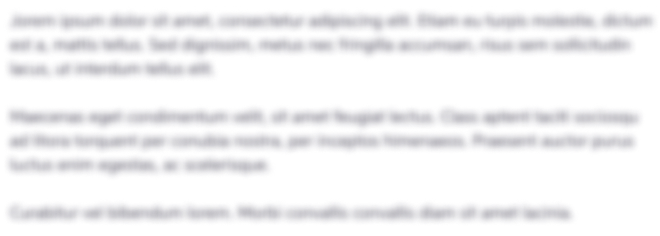
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started