Question
I need help with a few of the following functions. They are labled with /********************** Code goes here.**********************/. I have attached SLLNodeForInt.java and RecursionPractice.java package
I need help with a few of the following functions. They are labled with /**********************Code goes here.**********************/. I have attached SLLNodeForInt.java and RecursionPractice.java
package apps;
public class SLLNodeForInt {
int info;
SLLNodeForInt next;
public SLLNodeForInt(int info) {
this.info = info;
next = null;
}
}
_________________________________________________________
package apps;
public class RecursionPractice {
public static void printAlphabetsHelper(char ch)
{
System.out.print(ch);
ch++;
if (ch <= 'Z') {
printAlphabetsHelper(ch);
}
}
public static void printAlphabets()
{
// This is complete. Nothing to do in this function.
// this is a wrapper function that simply rely on recursive function printAlphabetHelper(ch)
// This prints "ABCDEFGHIJKLMNOPQRSTUVWXYZ" one character after another.
printAlphabetsHelper('A');
}
public static boolean isLarger(int a, int b)
{
// Assume both a and b are non-negative integers.
// NOT ALLOWED TO USE '<' or '>' operators, only '==' is allowed. Use recursion
// if a is larger, then returns true
// Or (including equal), returns false
if ((a-b) == -1)
return false;
else
return true; // change this as needed
}
public static void printPrimeFactorsHelper(int n, int divisor)
{
// if n is divided by divisor, then print divisor
// and recursive call with (n/divisor, divisor)
// if n is not divided by divisor, recursive call with (n, divisor+1)
// stop when n is too small
int temp = n/divisor;
if(divisor == temp)
divisor++;
else if(temp != 0)
printPrimeFactorsHelper(temp, divisor);
}
public static void printPrimeFactors(int n)
{
// This is complete. Nothing to do in this function.
// This prints prime factors of n.
// eg) printPrimeFactors(60) will print 2 2 3 5
printPrimeFactorsHelper(n, 2);
System.out.println();
}
public static int sumAll(SLLNodeForInt node)
{
// sum all values in the list
// eg) List 10,-5,10,20 --> sumAll() returns 35.
/**********************
Code goes here.
**********************/
return 0; // change this as needed
}
public static int max(int a, int b)
{
// this is complete. This may be useful for your findMaxhelper()
if (a>b) return a;
return b;
}
public static int findMax(SLLNodeForInt node)
{
// returns the maximum value in the linked list
/**********************
Code goes here.
**********************/
return 1; // change this as needed
}
public static void printLinkedListReverse(SLLNodeForInt node)
{
// print the linked list contents in reverse order.
// For example of a list A-->B-->C
// this prints "C B A"
SLLNodeForInt curNode = node;
while(curNode != null)
System.out.println(curNode.info + " ");
curNode = curNode.next;
return; // change this as needed
}
public static boolean subsetSum(SLLNodeForInt node, int target)
{
// returns true if any of subset of numbers[] sum to target
// For example, The list is {2,3,5,7,11}, target=12
// a subset {5,7} sums to 12.
// Another subset {2,3,7} sums to 12 too.
// If no such subset exist, it returns false.
/**********************
Code goes here.
**********************/
return true; // change this as needed
}
public static SLLNodeForInt remove(SLLNodeForInt node, int val)
{
// remove the first instance of 'val'
// eg) List 10,-5,10,20,10,4 --> -5,10,20,10,4 after remove()
// return if the given list is null
// if node has info equal to val, skip this node and return whatever is returned from its recursive call
// if node has info NOT equal to val, return this node with its connection to whatever is returned from its recursive call
/**********************
Code goes here.
**********************/
return null; // change this as needed
}
public static SLLNodeForInt removeAll(SLLNodeForInt node, int val)
{ // requirement: worst-case time complexity O(N) while N is the number elements in the list
// similar to remove() above.
// remove all instances of 'val'
// eg) List 10,-5,10,20,10,4 --> -5,20,4 after removeAll()
/**********************
Code goes here.
**********************/
return null; // change this as needed
}
// Following functions are here just to help testing
static SLLNodeForInt insertLinkedList(SLLNodeForInt list, int val) {
SLLNodeForInt newNode = new SLLNodeForInt(val);
newNode.next = list;
list = newNode;
return list;
}
static SLLNodeForInt buildLinkedList(int[] nums) {
SLLNodeForInt ls = null;
for(int i=nums.length-1;i>=0;i--) {
ls = insertLinkedList(ls, nums[i]);
}
return ls;
}
static void printLinkedList(SLLNodeForInt node) {
if (node == null) {
System.out.println();
return;
}
System.out.print(node.info+" ");
printLinkedList(node.next);
}
public static void main(String[] args) {
printAlphabets();
System.out.println();
System.out.println(isLarger(10,11));
printPrimeFactors(2*2*3*11*13*17);
SLLNodeForInt ls = buildLinkedList(new int[] {10,-5,10,20,10});
System.out.println("The total sum is "+sumAll(ls));
// System.out.println("The Max number is "+findMax(ls));
// printLinkedListReverse(ls);
// System.out.println(subsetSum(buildLinkedList(new int[]{2,3,5,7,11}), 12));
//
// ls = remove(ls, 10); System.out.print("List is ..."); printLinkedList(ls);
// ls = removeAll(ls, 10); System.out.print("List is ..."); printLinkedList(ls);
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
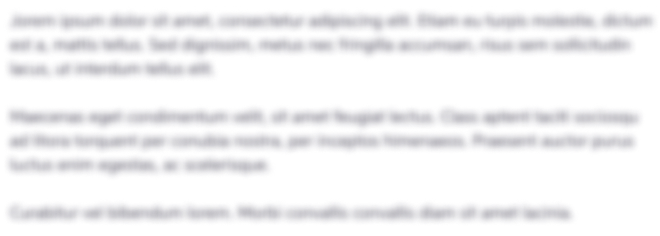
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started