Question
I need help with creating UML Hierarchy Diagram and UML Class Diagrams for each class. Please, use the UML Documents Required instruction below. -------------------------------------------------------------------------- UML
I need help with creating UML Hierarchy Diagram and UML Class Diagrams for each class. Please, use the UML Documents Required instruction below.
--------------------------------------------------------------------------
UML Documents Required:
UML Hierarchy Diagram
UML Class Diagrams for each Class (Auto, Bicycle, Brand, Color, Parkable, ParkingLot, ParkingSpace, ValidParkingObject, Vehicle)
----------------------------------------------------------------------------
Auto
public class Auto extends Vehicle implements Parkable {
private String plate; private Color color; private boolean hasPermit;
/** * Default Auto */ public Auto() { super(); plate = "N/A"; color = Color.Unknown; hasPermit = false; }
/** * Full parameter Constructor * @param inPlate String with Plate Data * @param inColor String with Color Data * @param inHasPermit boolean: True for has permit, False for not having permit */ public Auto(String inPlate, String inColor, boolean inHasPermit, int inSpeed, int inPass) { super(inSpeed, inPass); setPlate(inPlate); setColor(inColor); hasPermit = inHasPermit; }
@Override public boolean park() { if (isHasPermit()) { return true; } else { return false; } }
/** * Describes the Auto * @return a String with the Auto's data */ @Override public String toString() { return "Auto{" + "plate=" + plate + ", color=" + color + ", hasPermit=" + hasPermit + super.toString() + '}'; } /** * Get the Auto's license plate data * @return a String with License Plate data */ public String getPlate() { return plate; }
/** * Set the Auto's license plate data * @param inPlate a String to use for the Auto's License Plate data */ public void setPlate(String inPlate) { if (inPlate.length() > 0 && inPlate.length() < 9) plate = inPlate; else System.out.println("Attempted to set plate to a String too long or too short!"); }
/** * Get the color of the Auto * @return a String containing the color information for the Auto */ public Color getColor() { return color; }
/** * Set the color of the Auto * @param inColor a String denoting the color of the Auto */ public void setColor(String inColor) { Color[] validColors = Color.values(); boolean foundMatch = false; //Check the elements/values in the array for (int index = 0; index < validColors.length; index++) { if (inColor.equals( validColors[index].toString() )) { foundMatch = true; //We found a match!!! } } inColor = inColor.replaceAll(" ", "");//Removing Spaces! //foundMatch is true ONLY IF we found a matching enum value if (foundMatch) { color = Color.valueOf(inColor); } else { color = Color.Unknown; }
}
/** * Check if the Auto has a permit * @return a true if Auto has a permit and a false if Auto does NOT have a permit */ public boolean isHasPermit() { return hasPermit; }
/** * Sets the Auto's Permit status * @param inPermit a boolean denoting if the Auto should (true) or should not (false) have a permit */ public void setHasPermit(boolean inPermit) { hasPermit = inPermit; } public boolean equals(Object otherObject) {
//Check if the other object is even an Auto if (!(otherObject instanceof Auto)) { return false; //Since it's not even a Auto! } else { // Your logic for figuring out if the objects should match goes here! if (((Auto) otherObject).getPlate().equals(getPlate()) && ((Auto) otherObject).getColor().equals(getColor()) && ((Auto) otherObject).isHasPermit() == (isHasPermit())) { return true; } else { return false; // At least 1 attribute is different } } }
@Override public boolean unpark() { return true; } }
Bicycle
public class Bicycle extends Vehicle implements Parkable { private String serialNumber; private Brand brand; private boolean locked;
/** * Default Bicycle */ public Bicycle() { super(); serialNumber = "N/A"; brand = Brand.Unknown; locked = false; }
/** * Full parameter Bicycle constructor * @param inSerialNumber String with Serial Number Data * @param inBrand String with Brand Data * @param inLocked boolean: True if bike is locked, False if bike is not locked */ public Bicycle(String inSerialNumber, String inBrand, boolean inLocked, int inSpeed, int inPass) { super(inSpeed,inPass); setSerialNumber(inSerialNumber); setBrand(inBrand); locked = inLocked; }
@Override public boolean park() { setLocked(true); return true; }
/** * Describes the Bicycle * @return a String with the Bicycle's data */ @Override public String toString() { return "Bicycle{" + "serialNumber=" + serialNumber + ", brand=" + brand + ", locked=" + locked + super.toString() + '}'; }
/** * The Bicycle's serial number * @return a String containing the Bicycle's serial number */ public String getSerialNumber() { return serialNumber; }
/** * Change the Bicycle's serial number * @param inSerialNumber a String to set as the Bicycle's serial number */ public void setSerialNumber(String inSerialNumber) { if ( inSerialNumber.length() > 2 && inSerialNumber.length() < 14) serialNumber = inSerialNumber; else System.out.println("Attempted to set serial number to something too small or too large"); }
/** * Get the type of Bicycle * @return a String with the brand of the Bicycle */ public Brand getBrand() { return brand; }
/** * Set the type of Bicycle * @param inBrand a String with the brand info for the Bicycle */ public void setBrand(String inBrand) { Brand[] validBrands = Brand.values(); boolean foundMatch = false; //Check the elements/values in the array for (int index = 0; index < validBrands.length; index++) { if (inBrand.equals( validBrands[index].toString() )) { foundMatch = true; //We found a match!!! } } inBrand = inBrand.replaceAll(" ", "");//Removing Spaces! //foundMatch is true ONLY IF we found a matching enum value if (foundMatch) { brand = Brand.valueOf(inBrand); } else { brand = Brand.Unknown; }
}
/** * Check if the Bicycle is locked or not * @return a boolean with data on if the Bicycle is locked (true) or not (false) */ public boolean isLocked() { return locked; }
/** * Set the locked status of the Bicycle * @param lock a boolean which locks (true) or unlocks (false) a Bicycle */ public void setLocked(boolean lock) { locked = lock; } public boolean equals(Object otherObject) { if (!(otherObject instanceof Bicycle)) { return false; //Since it's not even a Bicycle! } else { if (((Bicycle) otherObject).getSerialNumber().equals(getSerialNumber()) && ((Bicycle) otherObject).getBrand().equals(getBrand()) && ((Bicycle) otherObject).isLocked() == (isLocked())) { return true; } else { return false; // At least 1 attribute is different } } }
@Override public boolean unpark() { setLocked(false); return true; } }
public enum Brand { Unknown, Schwinn, Huffy, Aero; public static Brand randomBrand() { Brand[] myBrands = Brand.values(); Random gen = new Random(); int index = gen.nextInt((myBrands.length -1)) + 1; return myBrands[index]; } public String toString() { String base = super.toString(); String output = ""; boolean firstChar = true; for (int index = 0; index < base.length(); index++) { //Check for uppercase if (Character.isUpperCase( base.charAt(index) ) && !firstChar) { output = output + " "; } output = output + base.charAt(index); firstChar = false; } return output; } }
Color
public enum Color { Unknown, Red, Orange, Pink, Black; public static Color randomColor() { Color[] myColors = Color.values(); Random gen = new Random(); int index = gen.nextInt((myColors.length -1)) + 1; return myColors[index]; } public String toString() { String base = super.toString(); String output = ""; boolean firstChar = true; for (int index = 0; index < base.length(); index++) { //Check for uppercase if (Character.isUpperCase( base.charAt(index) ) && !firstChar) { output = output + " "; } output = output + base.charAt(index); firstChar = false; } return output; } }
Parkable
public interface Parkable { /** * Method that parks a Parkable vehicle * @return true if vehicle is correctly parked */ boolean park(); /** * Method that unparks a Parkable vehicle * @return true if vehicle has been correctly unparked */ boolean unpark(); }
ParkingLot
public class ParkingLot { private ParkingSpace[] spaces; private double percentFull; /** * Constructor for a Parking Lot * @param numAuto number of Auto ParkingSpaces * @param numBike number of Bicycle ParkingSpaces */ public ParkingLot(int numAuto, int numBike) { spaces = new ParkingSpace[numAuto + numBike]; for (int i = 0; i < numAuto; i++) { spaces[i] = new ParkingSpace("Auto"); } for (int i = numAuto; i < spaces.length; i++) { spaces[i] = new ParkingSpace("Bicycle"); } percentFull = 0; }
@Override public String toString() { int count = 0; for (int i = 0; i < spaces.length; i++) { if (spaces[i].isOccupied()) { count++; } } percentFull = (100 * count) / spaces.length; return "ParkingLot{" + "spaces=" + spaces.length + ", percentFull=" + percentFull + "%}"; } /** * Try to park a given Vehicle * @param inAuto Auto to park * @return True if we could park, false otherwise */ public boolean park(Auto inAuto) { for(int i = 0; i < spaces.length; i++) { if (!spaces[i].isOccupied() && spaces[i].getType() == ValidParkingObject.Auto) { spaces[i].parkAuto(inAuto); return true; } } return false; }
/** * Try to park a given Vehicle * @param inBike Auto to park * @return True if we could park, false otherwise */ public boolean park(Bicycle inBike) { for(int i = 0; i < spaces.length; i++) { if (!spaces[i].isOccupied() && spaces[i].getType() == ValidParkingObject.Bicycle) { spaces[i].parkBicycle(inBike); return true; } } return false; }
/** * Try to find and return a parked vehicle * @param target Plate or Serial Number to look for * @return The parked vehicle OR a null if no such vehicle could be found */ public Object find(String target) { for(int i = 0; i < spaces.length; i++) { if (spaces[i].isOccupied() && spaces[i].getType() == ValidParkingObject.Auto && ((Auto) spaces[i].getVehicle()).getPlate().equals(target)) { return spaces[i].unpark(); } else if (spaces[i].isOccupied() && spaces[i].getType() == ValidParkingObject.Bicycle && ((Bicycle) spaces[i].getVehicle()).getSerialNumber().equals(target)) { return spaces[i].unpark(); } } return null; } }
Parking Space
public class ParkingSpace { private boolean occupied; private int number; private ValidParkingObject type; private Object vehicle; private static int numberOfSpaces = 0;
/** * Gets the vehicle parked in the ParkingSpace (DOES NOT REMOVE VEHICLE) * @return the vehicle parked in the ParkingSpace */ public Object getVehicle() { return vehicle; }
/** * Default Parking Space */ public ParkingSpace() { occupied = false; number = numberOfSpaces; numberOfSpaces++; type = ValidParkingObject.Unknown; vehicle = null; }
/** * Full parameter ParkingSpace constructor * @param inType String noting the type of object which can park in this Parking Space */ public ParkingSpace(String inType) { occupied = false; number = numberOfSpaces; numberOfSpaces++; setType(inType); vehicle = null; }
/** * Describes the Parking Space * @return a String with the Parking Space's data */ @Override public String toString() { return "ParkingSpace{" + "occupied=" + occupied + ", number=" + number + ", type=" + type + ", vehicle=" + vehicle + '}'; } /** * Try to park an Auto in this Parking Space * @param inAuto the Auto try to park here * @return a boolean noting if the Auto was able to park (true) or not (false) */ public boolean parkAuto(Auto inAuto) { if (isOccupied()) { return false; } else if (getType().equals(ValidParkingObject.Auto)) { occupied = true; vehicle = inAuto; return true; } else return false; } /** * Try to park a Bicycle in this Parking Space * @param inBike the Bicycle to try parking here * @return a boolean noting if the Bicycle was able to park (true) or not (false) */ public boolean parkBicycle (Bicycle inBike) { if (isOccupied()) { return false; } else if (getType().equals(ValidParkingObject.Bicycle)) { vehicle = inBike; occupied = true; return true; } else return false; }
/** * Unparks a vehicle from the ParkingSpace * @return the Vehicle in the ParkingSpace */ public Object unpark() { occupied = false; return vehicle; } /** * Check if the Parking Space is occupied or not * @return a boolean which is true if the Parking Space is in use and false if the Parking Space is not in use */ public boolean isOccupied() { return occupied; }
/** * Get the ParkingSpace number * @return an int with the ParkingSpace's number */ public int getNumber() { return number; }
/** * Get the type of ParkingSpace * @return a String denoting what type of Object is allowed to park in this ParkingSpace */ public ValidParkingObject getType() { return type; }
/** * Set the type of Parking Space * @param inType a String to note what type of Object is allowed to park in this Parking Space */ public void setType(String inType) { ValidParkingObject[] validTypes = ValidParkingObject.values(); boolean foundMatch = false; //Check the elements/values in the array for (int index = 0; index < validTypes.length; index++) { if (inType.equals( validTypes[index].toString() )) { foundMatch = true; //We found a match!!! } } inType = inType.replaceAll(" ", "");//Removing Spaces! //foundMatch is true ONLY IF we found a matching enum value if (foundMatch) { type = ValidParkingObject.valueOf(inType); } else { type = ValidParkingObject.Unknown; }
}
public boolean equals(Object otherObject) { if (!(otherObject instanceof ParkingSpace)) { return false; //Since it's not even a ParkingSpace! } else { if (((ParkingSpace) otherObject).getNumber() == (getNumber()) && ((ParkingSpace) otherObject).getType().equals(getType()) && ((ParkingSpace) otherObject).isOccupied() == (isOccupied())) { return true; } else { return false; // At least 1 attribute is different } } }
}
Valid parking object
public enum ValidParkingObject { Unknown, Auto, Bicycle; public static ValidParkingObject randomValidParkingObject() { ValidParkingObject[] myValidParkingObjects = ValidParkingObject.values(); Random gen = new Random(); int index = gen.nextInt((myValidParkingObjects.length -1)) + 1; return myValidParkingObjects[index]; } public String toString() { String base = super.toString(); String output = ""; boolean firstChar = true; for (int index = 0; index < base.length(); index++) { //Check for uppercase if (Character.isUpperCase( base.charAt(index) ) && !firstChar) { output = output + " "; } output = output + base.charAt(index); firstChar = false; } return output; } }
Vehicle
public class Vehicle { private int speed; private int pass;
public Vehicle() { speed = 0; pass = 0; }
public Vehicle(int speed, int pass) { setSpeed(speed); setPass(pass); }
@Override public String toString() { return "Vehicle{" + "speed=" + speed + ", pass=" + pass + '}'; }
public int getSpeed() { return speed; }
public void setSpeed(int inSpeed) { if (inSpeed > 0) speed = inSpeed; else speed = 0; }
public int getPass() { return pass; }
public void setPass(int inPass) { if (inPass > 0) pass = inPass; else pass = 0; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
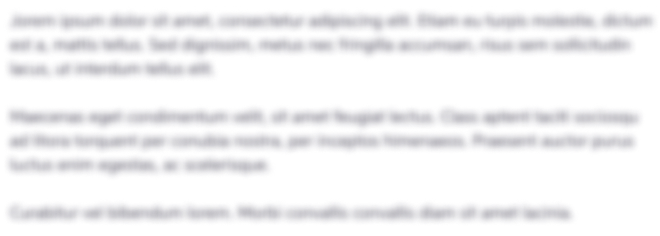
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started