Question
I need help with the following C++ assignment. Sequential/Binary Search. I have a few helper functions below. Please reach back if you have any questions.
I need help with the following C++ assignment. Sequential/Binary Search. I have a few helper functions below. Please reach back if you have any questions.
void displayArray(int a [], int s)
// if there are 200 elements or fewer, display the entire array
// (ten columns, values right-justified in the columns)
// if there are more than 200 elements,
// display the first 100 elements and the last 100 elements
void swapElement(int [] a, int i, int j){
// exchange elements i and j in array a
// and increment a global swapCount variable
bool compareElement(int [] a, int i, int j){
// return true if a[i]
// and increment a global compareCount variable
void copyArray(int [] source, int [] dest, int s){
// copy the first s elements from source into dest
void reverseArray(int [] a, int s){
// reverse the s elements in array a
The prototypes for the three simple sort algorithms are
void exchangeSort(int a [], int s);
void insertionSort(int a [], int s);
void insertionSort(int a [], int i, int j);
// sort from index i to index j inclusive
void selectionSort(int a [], int s);
Insertion sort. The complete code associated with the first prototype is
void insertionSort(int a [], int s){
insertionSort(a, 0, s-1);
}
The second insertion-sort function does all of the work.
Write a program that performs the following tasks: . Display a friendly greeting to the user Prompt the user for a file name Accept that file name Attempt to open the file for input o See note below for input file format o On failure, display an appropriate error message and exit . Read the data in the file and populate an array| Make a copy of the array Sort the copy, using any sorting algorithm desired The size of the list will not exceed 100,000 entries You now have two lists, one unsorted and one sorted. Prompt the user for a search item Accept that item Search the unsorted list using sequential search o Let the user know if it was found or not found." o Report the number of comparisons made during the search. Search the sorted copy of the list using sequential search o Report "found" or "not found" o Report the number of comparisons made during the search . The input file will contain an integer (the number of data items to follow) and at least that number of data items. Open the file, read the first entry, create your array using that size, and then populate the array with the following data items. The format of the file is: a single integer stating the number of entries to be read, followed by at least that many integers. For example, the file 10 32 65 11 2 5 34 66 22 98 9 contains ten elements: 32, 65, 11, 2.5, 34, 66, 22. 98, and 9. The leading 10 is not one of the data elements. That way you can open the file, read one integer, create your arrays, and then read the data into the arrays. Write a program that performs the following tasks: . Display a friendly greeting to the user Prompt the user for a file name Accept that file name Attempt to open the file for input o See note below for input file format o On failure, display an appropriate error message and exit . Read the data in the file and populate an array| Make a copy of the array Sort the copy, using any sorting algorithm desired The size of the list will not exceed 100,000 entries You now have two lists, one unsorted and one sorted. Prompt the user for a search item Accept that item Search the unsorted list using sequential search o Let the user know if it was found or not found." o Report the number of comparisons made during the search. Search the sorted copy of the list using sequential search o Report "found" or "not found" o Report the number of comparisons made during the search . The input file will contain an integer (the number of data items to follow) and at least that number of data items. Open the file, read the first entry, create your array using that size, and then populate the array with the following data items. The format of the file is: a single integer stating the number of entries to be read, followed by at least that many integers. For example, the file 10 32 65 11 2 5 34 66 22 98 9 contains ten elements: 32, 65, 11, 2.5, 34, 66, 22. 98, and 9. The leading 10 is not one of the data elements. That way you can open the file, read one integer, create your arrays, and then read the data into the arraysStep by Step Solution
There are 3 Steps involved in it
Step: 1
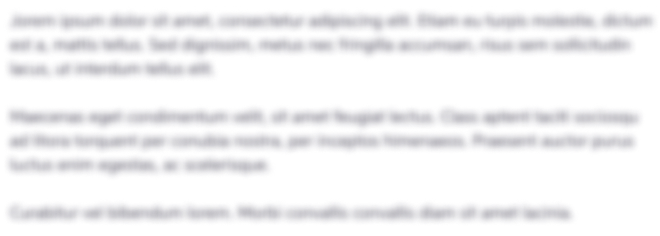
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started