I need help with the javascript so the page works like this: I have 3 external pages and they need to be separate, Below I've
I need help with the javascript so the page works like this: I have 3 external pages and they need to be separate, Below I've provided the html and CSS I just need help beginning the javascript to do the following:
- When the Convert from F to C or Convert from C to F button is clicked, the appropriate textbox above is read, the value there is converted to Celsius or Fahrenheit, and the converted temperature appears in the other textbox with exactly three digits after the decimal point. For example, if you enter -9 in the Celsius box and click the Convert from C to F button, you should see 15.800 in the Fahrenheit box; if you then click the Convert from F to C button, you should see -9.000 in the Celsius box. Use parseFloat to convert the input string to a number. If the input cannot be converted to a finite number, the output string should be Bad input.. A number's toFixed method will help when formatting the output number.
- When the Draw the box button is clicked, the textarea below it is cleared and a hollow square box of asterisks with sides of length equal to the number in the textbox above it is printed to the textarea. For example, if the number entered is 10, the output should look like
********** * * * * * * * * * * * * * * * * **********
If the number entered is 4, the output should look like**** * * * * ****
You may want to use a for loop inside a for loop. If the input is not a nonnegative integer, print only the message I need a number to draw a box. to the textarea. Your program should produce correct output whether the input number is 0, 1 or any higher integer. Use parseInt to convert a string into an integer. - When the Hailstone it button is clicked, the textarea below it is cleared and the hailstone sequence beginning with the number in the textbox above it is printed to the textarea, one number to a line, followed by the length of the sequence. If the input is not a positive integer, print only the message I need a number to start with. to the textarea. You can find the next number in a hailstone sequence by looking at the current number:
- If the current number is even, then the next number is half of the current number.
- If the current number is odd, then the next number is three times the current number plus one.
3 10 5 16 8 4 2 1 Length = 8
Let's make things happen
css below:
@charset "UTF-8";
/* CS 3312, spring 2021 Studio 4 DON'T CHANGE THIS FILE */
/* Fixing the box-sizing attribute will make sizing boxes easier. */ html { box-sizing: border-box; }
/* Normally the * selector is smart to avoid, but for this purpose it's fine. */ *, *:before, *:after { box-sizing: inherit; }
body { background-color: rgb(255, 250, 245); font-family: Georgia, serif; }
h1 { color: rgb(34, 68, 102); text-align: center; }
fieldset { border-color: rgb(68, 136, 204); border-radius: 10px; border-style: solid; border-width: 2px; float: left; }
legend { border-color: rgb(68, 136, 204); border-style: solid; border-width: 2px; padding: 0px 5px; }
input { text-align: center; }
label, textarea { display: block; }
/*jslint browser */
// CS 3312, spring 2021 // Studio 4 // YOUR NAME:
// All the code below will be run once the page content finishes loading. document.addEventListener('DOMContentLoaded', function () { 'use strict';
// Add your JavaScript code here. // Do things when user clicks the Convert from buttons************************************** document.querySelector('#convert-f-to-c').addEventListener('click', function () document.querySelector('#convert-f-to-c').addEventListener('click', function (){ // Empty the output elements. document.querySelector('#convert-f-to-c').value = ''; document.querySelector('#convert-c-to-f').value = ''; document.querySelector('#poem-error').textContent = ''; // Get all input strings. const fahrenheit = document.querySelector('#fahrenheit').value; const celsius = document.querySelector('#celsius').value; });
Step by Step Solution
There are 3 Steps involved in it
Step: 1
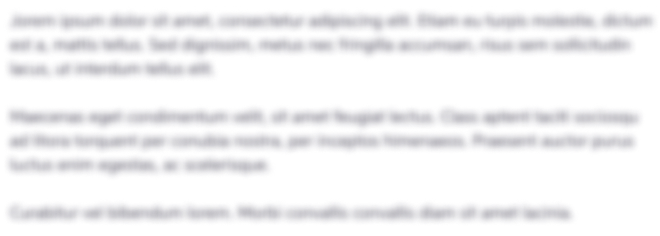
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started