Question
I need help with this assignment, can someone HELP ? This is the assignment: Online shopping cart (continued) (C++) This program extends the earlier Online
I need help with this assignment, can someone HELP ?
This is the assignment:
Online shopping cart (continued) (C++)
This program extends the earlier "Online shopping cart" program. (Consider first saving your earlier program).
(1) Extend the ItemToPurchase class per the following specifications:
- Parameterized constructor to assign item name, item description, item price, and item quantity (default values of 0). (1 pt)
- Public member functions
- SetDescription() mutator & GetDescription() accessor (2 pts)
- PrintItemCost() - Outputs the item name followed by the quantity, price, and subtotal
- PrintItemDescription() - Outputs the item name and description
- Private data members
- string itemDescription - Initialized in default constructor to "none"
Ex. of PrintItemCost() output:
Bottled Water 10 @ $1 = $10
Ex. of PrintItemDescription() output:
Bottled Water: Deer Park, 12 oz.
(2) Create three new files:
- ShoppingCart.h - Class declaration
- ShoppingCart.cpp - Class definition
- main.cpp - main() function (Note: main()'s functionality differs from the warm up)
Build the ShoppingCart class with the following specifications. Note: Some can be function stubs (empty functions) initially, to be completed in later steps.
- Default constructor
- Parameterized constructor which takes the customer name and date as parameters (1 pt)
- Private data members
- string customerName - Initialized in default constructor to "none"
- string currentDate - Initialized in default constructor to "January 1, 2016"
- vector < ItemToPurchase > cartItems
- Public member functions
- GetCustomerName() accessor (1 pt)
- GetDate() accessor (1 pt)
- AddItem()
- Adds an item to cartItems vector. Has parameter ItemToPurchase. Does not return anything.
- RemoveItem()
- Removes item from cartItems vector. Has a string (an item's name) parameter. Does not return anything.
- If item name cannot be found, output this message: Item not found in cart. Nothing removed.
- ModifyItem()
- Modifies an item's description, price, and/or quantity. Has parameter ItemToPurchase. Does not return anything.
- If item can be found (by name) in cart, check if parameter has default values for description, price, and quantity. If not, modify item in cart.
- If item cannot be found (by name) in cart, output this message: Item not found in cart. Nothing modified.
- GetNumItemsInCart() (2 pts)
- Returns quantity of all items in cart. Has no parameters.
- GetCostOfCart() (2 pts)
- Determines and returns the total cost of items in cart. Has no parameters.
- PrintTotal()
- Outputs total of objects in cart.
- If cart is empty, output this message: SHOPPING CART IS EMPTY
- PrintDescriptions()
- Outputs each item's description.
Ex. of PrintTotal() output:
John Doe's Shopping Cart - February 1, 2016 Number of Items: 8 Nike Romaleos 2 @ $189 = $378 Chocolate Chips 5 @ $3 = $15 Powerbeats 2 Headphones 1 @ $128 = $128 Total: $521
Ex. of PrintDescriptions() output:
John Doe's Shopping Cart - February 1, 2016 Item Descriptions Nike Romaleos: Volt color, Weightlifting shoes Chocolate Chips: Semi-sweet Powerbeats 2 Headphones: Bluetooth headphones
(3) In main(), prompt the user for a customer's name and today's date. Output the name and date. Create an object of type ShoppingCart. (1 pt) Ex.
Enter customer's name: John Doe Enter today's date: February 1, 2016 Customer name: John Doe Today's date: February 1, 2016
(4) Implement the PrintMenu() function. PrintMenu() has a ShoppingCart parameter, and outputs a menu of options to manipulate the shopping cart. Each option is represented by a single character. Build and output the menu within the function.
If the an invalid character is entered, continue to prompt for a valid choice. Hint: Implement Quit before implementing other options. Call PrintMenu() in the main() function. Continue to execute the menu until the user enters q to Quit. (3 pts) Ex:
MENU a - Add item to cart d - Remove item from cart c - Change item quantity i - Output items' descriptions o - Output shopping cart q - Quit Choose an option:
(5) Implement Output shopping cart menu option. (3 pts) Ex:
OUTPUT SHOPPING CART John Doe's Shopping Cart - February 1, 2016 Number of Items: 8 Nike Romaleos 2 @ $189 = $378 Chocolate Chips 5 @ $3 = $15 Powerbeats 2 Headphones 1 @ $128 = $128 Total: $521
(6) Implement Output item's description menu option. (2 pts) Ex.
OUTPUT ITEMS' DESCRIPTIONS John Doe's Shopping Cart - February 1, 2016 Item Descriptions Nike Romaleos: Volt color, Weightlifting shoes Chocolate Chips: Semi-sweet Powerbeats 2 Headphones: Bluetooth headphones
(7) Implement Add item to cart menu option. (3 pts) Ex:
ADD ITEM TO CART Enter the item name: Nike Romaleos Enter the item description: Volt color, Weightlifting shoes Enter the item price: 189 Enter the item quantity: 2
(8) Implement remove item menu option. (4 pts) Ex:
REMOVE ITEM FROM CART Enter name of item to remove: Chocolate Chips
(9) Implement Change item quantity menu option. Hint: Make new ItemToPurchase object and use ItemToPurchase modifiers before using ModifyItem() function. (5 pts) Ex:
CHANGE ITEM QUANTITY Enter the item name: Nike Romaleos Enter the new quantity: 3
P.S
I am including all files and the code that I have so far....
the Code is Below:
ShoppingCart.h (header file)
#ifndef SHOPPINGCART_H_
#define SHOPPINGCART_H_
#include
#include
#include "ItemToPurchase.h"
using namespace std;
class ShoppingCart
{
private:
string name;
string date;
vector
public:
ShoppingCart(string name, string date);
virtual ~ShoppingCart();
const string& getDate() const;
void setDate(const string& date);
const string& getName() const;
void setName(const string& name);
void add(ItemToPurchase &item);
void remove(string itemName);
void update(ItemToPurchase &item);
void showDescription();
void showCart();
void showOption();
};
#endif
Shoppingcart.cpp
#include "ShoppingCart.h"
#include
#include
using namespace std;
ShoppingCart::ShoppingCart(string name = "none", string date = "January 1, 2016")
{
this->name=name;
this->date=date;
}
const string& ShoppingCart::getDate() const
{
return date;
}
void ShoppingCart::setDate(const string& date)
{
this->date = date;
}
const string& ShoppingCart::getName() const
{
return name;
}
void ShoppingCart::setName(const string& name)
{
this->name = name;
}
void ShoppingCart::add(ItemToPurchase &newItem)
{
int set = 0;
if(newItem.GetName().compare("none") != 0)
{
//vector
//for (it = item.begin() ; it != item.end(); ++it)
for(int i=0;i { if(item.at(i).GetName() == newItem.GetName()) { cout<<"Item is already in cart. Nothing added."< set = 1; break; } } if(set == 0) item.push_back(newItem); } } void ShoppingCart::remove(string itemName) { //vector int i=0; //for (it = item.begin() ; it != item.end(); ++it) for(int j=0;j { i++; if(item.at(j).GetName() == itemName) { cout<<"found...removing "< item.erase (item.begin()+j); break; } } if(i == 0) { cout<<"Shopping cart is empty."< cout<<"No item found with name "< } } void ShoppingCart::update(ItemToPurchase &updateItem) { ///vector int i=0; //for (it = item.begin() ; it != item.end(); ++it) for(int j=0;j { i++; if(item.at(i).GetName() == updateItem.GetName()) { item.at(i).SetName(updateItem.GetName()); item.at(i).SetDescription(updateItem.GetDescription()); item.at(i).SetPrice(updateItem.GetPrice()); item.at(i).SetQuantity(updateItem.GetQuantity()); break; } } if(i == 0) { cout<<"Shopping cart is empty."< cout<<"No itemfound with name "< } } ShoppingCart::~ShoppingCart() { } void ShoppingCart::showDescription() { vector int i=0; cout<<""< //for (it = item.begin() ; it != item.end(); ++it) for(int j=0;j { cout << item.at(i).GetName() << " : " << item.at(i).GetDescription()<< endl; i++; } if(i == 0) cout<<"Shopping cart is empty."< } void ShoppingCart::showCart() { //vector int i = 0; double tot = 0; cout<<""< //for (it = item.begin() ; it != item.end(); ++it) for(int j=0;j { cout << item.at(i).GetName() << " " << item.at(i).GetQuantity() << " @ $" << item.at(i).GetPrice() << " = $" << item.at(i).GetPrice() * item.at(i).GetQuantity() << endl; tot = tot + (item.at(i).GetPrice() *item.at(i). GetQuantity()); i++; } if(i == 0) { cout<<"Shopping cart is empty."< cout<<"Total: $"< } } void ShoppingCart::showOption() { cout<<"\t\tMenu"< cout<<"add - Add item to cart"< cout<<"remove - Remove item from cart"< cout<<"change - Change item quantity"< cout<<"descriptions - Output items' descriptions"< cout<<"cart - Output shopping cart"< cout<<"options - Print the options menu"< cout<<"quit - Quit"< } itemToPurchase.h #ifndef ItemToPurchase_H_ #define ItemToPurchase_H_ #include using namespace std; class ItemToPurchase { private: string itemName; string itemDescription; double itemPrice; int itemQuantity; public: ItemToPurchase(); ItemToPurchase(string name, string desc, double price, int quantity); void SetName(string name); void SetPrice(double price); void SetQuantity(int quantity); string GetName(); double GetPrice(); int GetQuantity(); const string& GetDescription() const; void SetDescription(const string& itemDescription); void clean(); }; #endif itemToPurchase.cpp #include "ItemToPurchase.h" ItemToPurchase::ItemToPurchase() { itemName = "none"; itemDescription = "none"; itemPrice = 0; itemQuantity = 0; } ItemToPurchase::ItemToPurchase(string name, string desc, double price, int quantity) { if(name == "'") name = "none"; if(desc == "") desc = "none"; itemName = name; itemDescription = desc; itemPrice = price; itemQuantity = quantity; } void ItemToPurchase::SetName(string name) { itemName = name; } void ItemToPurchase::SetPrice(double price) { itemPrice = price; } void ItemToPurchase::SetQuantity(int quantity) { itemQuantity = quantity; } string ItemToPurchase::GetName() { return itemName; } double ItemToPurchase::GetPrice() { return itemPrice; } const string& ItemToPurchase::GetDescription() const { return itemDescription; } void ItemToPurchase::SetDescription(const string& itemDescription) { this->itemDescription = itemDescription; } int ItemToPurchase::GetQuantity() { return itemQuantity; } void ItemToPurchase::clean() { itemName = "none"; itemDescription = "none"; itemPrice = 0; itemQuantity = 0; } main,cpp #include #include "ItemToPurchase.h" #include "ShoppingCart.h" using namespace std; int main() { ItemToPurchase item; string name, desc; double price; int q; string custName,date; string option = ""; cout<<"Enter Customer's Name :"; getline(cin, custName); cout<<"Enter Today's Date : "; getline(cin, date); ShoppingCart sCart(name, date); sCart.setName(custName); sCart.setDate(date); sCart.showOption(); while(option.compare("quit") != 0 ) { cout<<"Enter option : "< getline(cin, option); if(option.compare("options") == 0) sCart.showOption(); else if(option.compare("add") == 0) { cout << "Enter the item name: "; getline(cin, name); cout << "Enter the item description: "; getline(cin, desc); cout << "Enter the item price: "; cin >> price; cout << "Enter the item quantity: "; cin >> q; item.SetName(name); item.SetDescription(desc); item.SetPrice(price); item.SetQuantity(q); sCart.add(item); item.clean(); cin.ignore(); } else if(option.compare("remove") == 0) { cout << "Enter the item name: "; getline(cin, name); sCart.remove(name); } else if(option.compare("change") == 0) { cout << "Enter the item name: "; getline(cin, name); cout << "Enter the item quantity: "; cin >> q; item.SetName(name); item.SetQuantity(q); sCart.update(item); item.clean(); cin.ignore(); } else if(option.compare("descriptions") == 0) sCart.showDescription(); else if(option.compare("cart") == 0) sCart.showCart(); } cout<<"Program..Terminated.."; return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
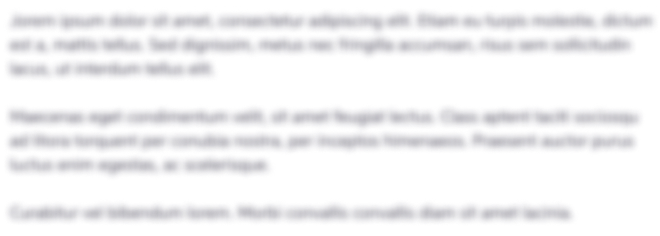
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started