Question
I need help with this assignment/. The instructions are: Create a class called BankAccount. The BankAccount class should contain a String to store the customer
I need help with this assignment/. The instructions are: Create a class called BankAccount. The BankAccount class should contain a String to store the customer name and a double to store the account balance. The BankAccount class should have two constructors, as follows: public BankAccount(String name, double balance) throws NegativeAmountException { // set name and balance // make sure balance is not negative // throw exception if balance is negative } public BankAccount(String name) throws NegativeAmountException { // set name and use 0 balance } As can be seen, the first constructor throws a NegativeAmountException if the balance being used to create the bank account is negative. You will have to create this exception class yourself. The BankAccount class should also contain methods to make a deposit, make a withdrawal, get the current balance, and print a bank account statement. The interfaces for these methods should appear as follows: // update balance by adding deposit amount // make sure deposit amount is not negative // throw exception if deposit is negative public void deposit(double amount) throws NegativeAmountException // update balance by subtracting withdrawal amount // throw exception if funds are not sufficient // make sure withdrawal amount is not negative // throw NegativeAmountException if amount is negative // throw InsufficientFundsException if balance
Here is the code I have so far:
1. BankAccount Code
import java.io.*;
class NegativeAmountException extends Exception //creates NegativeAmountException { public NegativeAmountException() { } public NegativeAmountException(String message) { super(message); } public String toString() { return "NegativeAmountException"; } } class InsufficientFundsException extends Exception //creates InsufficientFundsException { public InsufficientFundsException() { } public InsufficientFundsException(String message) { super(message); } public String toString() { return "InsufficientFundsException"; } } class BankAcct2FINAL { public String name; public double balance;
public BankAcct2FINAL(String name, double balance) throws NegativeAmountException { this.name = name; this.balance = balance; // set name and balance // throw exception if balance is negative if (balance
public BankAcct2FINAL(String name) throws NegativeAmountException { this(name, 0); // set name and use 0 balance }
// update balance by adding deposit amount // make sure deposit amount is not negative // throw exception if deposit is negative public void deposit(double amount) throws NegativeAmountException { if (amount > 0) { this.balance += amount; } else { throw new NegativeAmountException("Deposit amount must not be negative"); } }
// update balance by subtracting withdrawal amount // throw exception if funds are not sufficient // make sure withdrawal amount is not negative // throw NegativeAmountException if amount is negative // throw InsufficientFundsException if balance getBalance()) { throw new InsufficientFundsException( "You do not have sufficient funds for this operation."); } else if (amount
// return current balance public double getBalance() { return this.balance; }
// print bank statement including customer name // and current account balance public void printStatement() { System.out.println("Balance for " + this.name + ": " + getBalance()); } }
2. SavingsAccount Code
import java.util.Scanner; // Needed for the Scanner class import java.text.DecimalFormat; // Needed for 2 decimal place amounts import java.util.logging.Level; import java.util.logging.Logger;
class SavingsAccountFINAL extends BankAcct2FINAL { BankAcct2FINAL account; // To reference a BankAccount object double balance; // The account's starting balance double interestRate; // The annual interest rate // Create a Scanner object for keyboard input. Scanner keyboard = new Scanner(System.in); // Create an object for dollars and cents DecimalFormat formatter = new DecimalFormat("#0.00"); // Get the starting balance. // Get the annual interest rate. // post monthly interest by multiplying current balance // by current interest rate divided by 12 and then adding // result to balance by making deposit public void postInterest() { double Interest; interestRate=keyboard.nextDouble(); Interest = balance*(interestRate*.001)/12; try { this.deposit(Interest); // and current account balance (use printStatement from // the BankAccount superclass) // following this also print current interest rate } catch (Exception error) { Logger.getLogger(SavingsAccountFINAL.class.getName()).log(Level.SEVERE, null, error); } } }
The error I get for SavingsAccount file above is:
SavingsAccountFINAL.java:6: error: no suitable constructor found for BankAcct2FINAL(no arguments) class SavingsAccountFINAL extends BankAcct2FINAL ^ constructor BankAcct2FINAL.BankAcct2FINAL(String,double) is not applicable (actual and formal argument lists differ in length) constructor BankAcct2FINAL.BankAcct2FINAL(String) is not applicable (actual and formal argument lists differ in length) 1 error
3. FinalExam Code
import java.util.*;
public class FinalExam2 { public static void main (String[] args) { double amount = 0; SavingsAccountFINAL penny = null; try { penny = new SavingsAccountFINAL("Penny Saved", 500.00, .05); Scanner input = new Scanner(System.in); System.out.print("Enter your deposit amount: "); amount = input.nextDouble(); penny.deposit(amount); } catch(NegativeAmountException error) { System.out.println("NegativeAmountException: " + error.getMessage()); System.exit(1); } System.out.print("Enter your withdraw amount: "); try { amount = input.nextDouble(); penny.withdraw(amount); } catch(NegativeAmountException error) { System.out.println("NegativeAmountException: " + error.getMessage()); System.exit(1); }
I am totally lost and not sure what I am doing. Can you help?
CsD Eile Edit yew Build Project Settings lootsndow eip All Files Sort By Name import java.1 publie elass FinalExan public static voidmaan [String [] &rgs) thro#3 IOException SavingAccount savings-null ry FinalExam.class // in5tantiate a saving account savinga-nex Saving ecoune ("Penny Saved, 2000.00, 0.05) catch (NegativeimouncException nael) Browse Find Compile Messages GRASP Messages Run VO Interactions -3GRASP exee Javs FinalExam Clear ecoune or Penny Saved 51,000-00 balance 0.05 current ratef intere,t Help Enter deposit amount! 500 Account for Fenny Saved 52.500.00 balanee Enter withdraval anouat: 50 Account for Fenny Saved .450.00 balance 0.05t eurrent zate of intezest Posting monthiy interest ccount tor Penny Saved 1,456.04 balance 0.05t eurrent zate of interest Line:3 Cot1 Code:112
Step by Step Solution
There are 3 Steps involved in it
Step: 1
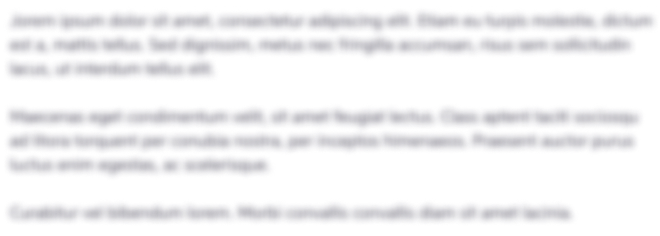
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started