Question
I need this is Java * 1. Implement interfaces: SimpleFractionInterface and Comparable (i.e. compareTo()) * 2. Implement methods equals() and toString() from class Object *
I need this is Java * 1. Implement interfaces: SimpleFractionInterface and Comparable (i.e. compareTo()) * 2. Implement methods equals() and toString() from class Object * 3. Must work for both positive and negative fractions * 4. Must not reduce fraction to lowest term unless simplifySimpleFraction() is invoked * 5. For input 3/-10 & -3/-10, convert them to -3/10 & 3/10 respectively (see Hint 2. below) * 6. Must display negative fraction as -x/y, * example: (-3)/10 or 3/(-10), must display as -3/10 * 7. Must throw only SimpleFractionException in case of errors * 8. Must not add new or modify existing data fields * 9. Must not add new public methods * 10.May add private methods * * Hints: * * 1. To reduce a fraction such as 4/8 to lowest terms, you need to divide both * the numerator and the denominator by their greatest common denominator. * The greatest common denominator of 4 and 8 is 4, so when you divide * the numerator and denominator of 4/8 by 4, you get the fraction 1/2. * The recursive algorithm which finds the greatest common denominator of * two positive integers is implemented (see code) * * 2. It will be easier to determine the correct sign of a fraction if you force * the fraction's denominator to be positive. However, your implementation must * handle negative denominators that the client might provide. * * 3. You need to downcast reference parameter SimpleFractionInterface to SimpleFraction if * you want to use it as SimpleFraction. See add, subtract, multiply and divide methods * * 4. Use "this" to access this object if it is needed
Using:
-
/* This file specifies methods for SimpleFractionInterface */ /* Do not modify this file!! */
package PJ1;
public interface SimpleFractionInterface { /** Task: Sets "this" fraction to a given value. * @param num is the integer numerator * @param den is the integer denominator * @throw SimpleFractionException if denominator is 0 */ public void setSimpleFraction(int num, int den);
/** Task: Reduce "this" fraction to lowest term, i.e divide the numerator and denominator by their Greatest Common Divisor */ public void simplifySimpleFraction();
/** Task: compute floating value of "this" fraction * @return the double floating point value of a fraction */ public double toDouble();
/** Task: Adds two fractions. * @param secondFraction is a fraction that is the second operand of the addition * @return a new reduced fraction which is the sum of "this" fraction and the secondFraction */ public SimpleFractionInterface add(SimpleFractionInterface secondFraction);
/** Task: Subtracts two fractions. * @param secondFraction a fraction that is the second operand of the subtraction * @return a new reduced fraction which is the difference of "this" fraction and the second operand */ public SimpleFractionInterface subtract(SimpleFractionInterface secondFraction);
/** Task: Multiplies two fractions. * @param secondFraction a fraction that is the second operand of the multiplication * @return a new reduced fraction which is the product of "this" fraction and the secondFraction*/ public SimpleFractionInterface multiply(SimpleFractionInterface secondFraction);
/** Task: Divides two fractions. * @param secondFraction a fraction that is the second operand of the division * @return a new reduced fraction which the quotient of "this" fraction and the secondFraction * @throw SimpleFractionException if secondFraction is 0 */ public SimpleFractionInterface divide(SimpleFractionInterface secondFraction);
}
And
-
/* This program is used to test PJ1.Fracition class * More info are given in Readme file */
import java.util.*; import PJ1.*;
class PJ1_Test { static private Scanner scanner;
private static SimpleFraction readFraction() { System.out.print( " Try to read a fraction x/y, please enter x y : " ); int numerator = scanner.nextInt(); int denominator = scanner.nextInt(); SimpleFraction f = new SimpleFraction(numerator, denominator); System.out.println( "\t\tRead OK:"+f); return f; }
private static void printOperations() { System.out.println("=============================================="); System.out.println(" Operations:"); System.out.println(" 0) exit \t1) add \t\t2) subtract \t3) multiply \t4) divide"); System.out.println(" 5) compareTo \t6) equals \t7) simplifySimpleFraction t8) toDouble \t9) setSimpleFraction (x/y) "); System.out.print( " Enter an operation number: "); }
public static void main( String args[] ) { scanner = new Scanner( System.in ); // scanner for input boolean continueLoop = true; // determines if more input is needed SimpleFraction n1=null; SimpleFraction n2=null; int op,x,y; do { try // read two numbers and calculate quotient { printOperations(); op= scanner.nextInt();
if (op == 0) { break; } else if ((op >0) && (op <7)) { n1 = readFraction(); n2 = readFraction(); } else if ((op > 6) && (op < 9)) { n1 = readFraction(); } else if (op == 9) { n1 = new SimpleFraction(); } else { System.out.print( " Invalid input... try again " ); continue; }
System.out.println(" Tests: "); switch (op) { case 1: System.out.println("\t" + n1 + " + " + n1 + " = " + n1.add(n1)); System.out.println("\t" + n2 + " + " + n2 + " = " + n2.add(n2)); System.out.println("\t" + n1 + " + " + n2 + " = " + n1.add(n2)); System.out.println("\t" + n2 + " + " + n1 + " = " + n2.add(n1)); break; case 2: System.out.println("\t" + n1 + " - " + n1 + " = " + n1.subtract(n1)); System.out.println("\t" + n2 + " - " + n2 + " = " + n2.subtract(n2)); System.out.println("\t" + n1 + " - " + n2 + " = " + n1.subtract(n2)); System.out.println("\t" + n2 + " - " + n1 + " = " + n2.subtract(n1)); break; case 3: System.out.println("\t" + n1 + " * " + n1 + " = " + n1.multiply(n1)); System.out.println("\t" + n2 + " * " + n2 + " = " + n2.multiply(n2)); System.out.println("\t" + n1 + " * " + n2 + " = " + n1.multiply(n2)); System.out.println("\t" + n2 + " * " + n1 + " = " + n2.multiply(n1)); break; case 4: System.out.println("\t" + n1 + " / " + n1 + " = " + n1.divide(n1)); System.out.println("\t" + n2 + " / " + n2 + " = " + n2.divide(n2)); System.out.println("\t" + n1 + " / " + n2 + " = " + n1.divide(n2)); System.out.println("\t" + n2 + " / " + n1 + " = " + n2.divide(n1)); break; case 5: System.out.println("\t" + n1 + " ct " + n1 + " = " + n1.compareTo(n1)); System.out.println("\t" + n2 + " ct " + n2 + " = " + n2.compareTo(n2)); System.out.println("\t" + n1 + " ct " + n2 + " = " + n1.compareTo(n2)); System.out.println("\t" + n2 + " ct " + n1 + " = " + n2.compareTo(n1)); break; case 6: System.out.println("\t" + n1 + " eq "+ n1 + " = " + n1.equals(n1)); System.out.println("\t" + n2 + " eq "+ n2 + " = " + n2.equals(n2)); System.out.println("\t" + n1 + " eq "+ n2 + " = " + n1.equals(n2)); System.out.println("\t" + n2 + " eq "+ n1 + " = " + n2.equals(n1)); break; case 7: System.out.print("\t" + n1 ); n1.simplifySimpleFraction(); System.out.println(" simplifySimpleFraction " + n1); break; case 8: System.out.println("\t" + n1 + " toDouble = " + n1.toDouble()); break; case 9: System.out.print( "read a fraction x/y, please enter x y : " ); x = scanner.nextInt(); y = scanner.nextInt(); System.out.print("\t" + x +"/"+ y + " setSimpleFraction = "); n1.setSimpleFraction(x,y); System.out.println(n1); break; }
} // end try catch ( SimpleFractionException e) { System.err.printf( " Fraction Exception: %s ", e); } // end catch } while ( continueLoop ); // end do...while } // end main } // end class
Every time I write the code I can't get it to compile. This is my code. Please tell me what I'm doing wrong
public class SimpleFraction implements SimpleFractionInterface, Comparable
public SimpleFraction() { //Set fraction to 0/1 num = 0; den = 1; }
public SimpleFraction(int num, int den) { //Set fraction to 0/1. Throw exception if denominator is 0 if(den == 0){ throwException(); } else{ this.num = num; this.den = den; } }
public void setSimpleFraction(int num, int den) { // return SimpleFractionException if initial Denominator is 0 if(den == 0){ throwException(); } else{ this.num = num; this.den = den; } }
public double toDouble() { // return double floating point value double fractionAsDouble = (double)(num) / (double)(den);
return fractionAsDouble; }
public SimpleFractionInterface add(SimpleFractionInterface secondFraction) { //Used when accessing formatted fraction array final int numIndex = 0; final int denIndex = 1;
// Downcast interface object to SimpleFraction object SimpleFraction fractionTwo = (SimpleFraction) secondFraction;
//Convert secondFraction into a string String fractionTwoString = fractionTwo.toString();
//convert current fraction into a string String fractionOneString = this.toString();
//Assign a/b and c/d using fractionOneString and fractionTwoString int a = getIntNumerator(fractionOneString); int b = getIntDenominator(fractionOneString); int c = getIntNumerator(fractionTwoString); int d = getIntDenominator(fractionTwoString);
//Add using formnula: a/b + c/d is (ad + cb)/(bd) int newNum = (a * d) + (c * b); int newDen = b * d;
//Get our formatted fraction int[] formattedFraction = formatProperFraction(newNum, newDen);
//Create new Simple fraction object with our formatted num and den SimpleFraction resultFraction = new SimpleFraction(formattedFraction[numIndex], formattedFraction[denIndex]);
//Reduce fraction resultFraction.reduceSimpleFractionToLowestTerms();
return resultFraction; }
public SimpleFractionInterface subtract(SimpleFractionInterface secondFraction) { //Used when accessing formatted fraction array final int numIndex = 0; final int denIndex = 1;
//Downcast secondFraction to SimpleFraction SimpleFraction fractionTwo = (SimpleFraction) secondFraction;
//Convert fractions to strings String fractionOneString = this.toString(); String fractionTwoString = fractionTwo.toString();
//Assign a/b and c/d using fractionOneString and fractionTwoString int a = getIntNumerator(fractionOneString); int b = getIntDenominator(fractionOneString); int c = getIntNumerator(fractionTwoString); int d = getIntDenominator(fractionTwoString);
//Subtract using formula: a/b - c/d is (ad - cb)/(bd) int newNum = (a * d) - (c * b); int newDen = b * d;
//Get formatted fraction int[] formattedFraction = formatProperFraction(newNum, newDen);
//Create new simple fraction object with formatted num and den SimpleFraction resultFraction = new SimpleFraction(formattedFraction[numIndex], formattedFraction[denIndex]);
//Reduce fraction resultFraction.reduceSimpleFractionToLowestTerms();
return resultFraction; }
public SimpleFractionInterface multiply(SimpleFractionInterface secondFraction) { //Used when accessing formatted fraction array final int numIndex = 0; final int denIndex = 1; //Downcast secondFraction to a SimpleFraction SimpleFraction fractionTwo = (SimpleFraction) secondFraction;
//Convert fractions to strings String fractionOneString = this.toString(); String fractionTwoString = fractionTwo.toString();
//Assign a/b and c/d using fractionOneString and fractionTwoString int a = getIntNumerator(fractionOneString); int b = getIntDenominator(fractionOneString); int c = getIntNumerator(fractionTwoString); int d = getIntDenominator(fractionTwoString);
//Multiply using formula: a/b * c/d is (ac)/(bd) int newNum = a * c; int newDen = b * d;
//Get formatted fraction int[] formattedFraction = formatProperFraction(newNum, newDen);
//Create new SimpleFraction object with formatted num and den SimpleFraction resultFraction = new SimpleFraction(formattedFraction[numIndex], formattedFraction[denIndex]);
//Reduce fraction resultFraction.reduceSimpleFractionToLowestTerms();
return resultFraction; }
public SimpleFractionInterface divide(SimpleFractionInterface secondFraction) { //Used when accessing formatted fraction array final int numIndex = 0; final int denIndex = 1;
//Downcase secondFraction to SimpleFraction SimpleFraction fractionTwo = (SimpleFraction) secondFraction;
//Convert fractions to strings String fractionOneString = this.toString(); String fractionTwoString = fractionTwo.toString();
//Assign a/b and c/d using fractionOneString and fractionTwoString int a = getIntNumerator(fractionOneString); int b = getIntDenominator(fractionOneString); int c = getIntNumerator(fractionTwoString); int d = getIntDenominator(fractionTwoString);
//Check to see if denominator is 0. If so, throw exception if(b == 0|| d == 0){ throwException(); }
//Divide using formula: a/b / c/d is (ad)/(bc) int newNum = a * d; int newDen = b * c;
//Get formatted fraction int[] formattedFraction = formatProperFraction(newNum, newDen);
//Create new SimpleFraction object with formatted num and den SimpleFraction resultFraction = new SimpleFraction(formattedFraction[numIndex], formattedFraction[denIndex]);
//Reduce fraction resultFraction.reduceSimpleFractionToLowestTerms();
return resultFraction; }
public SimpleFractionInterface getReciprocal() { //Create ints with switched numerator and denominator int recipNum = this.den; int recipDen = this.num;
//Create new SimpleFraction object using reciprocated values SimpleFraction reciprocal = new SimpleFraction(recipNum, recipDen);
return reciprocal; }
public boolean equals(Object other) { //Cast other to SimpleFraction SimpleFraction otherFraction = (SimpleFraction) other;
//Reduce fractions this.reduceSimpleFractionToLowestTerms(); otherFraction.reduceSimpleFractionToLowestTerms();
//Return the string values of each fraction return this.toString().equals(otherFraction.toString()); }
public int compareTo(SimpleFraction other) { //Convert SimpleFractions to doubles and compare double thisDouble = this.toDouble(); double otherDouble = other.toDouble();
double result = Double.compare(thisDouble, otherDouble);
//Return int value of double (either negative, 0, or positive result) return (int) result; }
public String toString() { return num + "/" + den; } private int getIntDenominator(String fraction){
//Delimit where the denominator starts int delimiter = fraction.indexOf("/");
//Get only the denominator of the string, first param is inclusive, so we add one //To exclude the backslash String stringResult = fraction.substring(delimiter + 1, fraction.length());
//Convert to integer int denominator = Integer.parseInt(stringResult);
return denominator; } private int getIntNumerator(String fraction){
//Set start index of fraction final int fractionStart = 0;
//Delimit where the denominator starts int delimiter = fraction.indexOf("/");
//Get only the numerator of the string String stringResult = fraction.substring(fractionStart, delimiter);
//Convert to integer int numerator = Integer.parseInt(stringResult);
return numerator; }
private int[] formatProperFraction(int num, int den){ //Store fraction as [num, den] int[] fractionArray = new int[2];
if(den < 0){ //Force positive den = Math.abs(den);
//Make num negative instead of den fractionArray[0] = -1 * num; fractionArray[1] = den;
return fractionArray; } else{ fractionArray[0] = num; fractionArray[1] = den;
return fractionArray; }
}
//Throw exception for 0 denominator private void throwException(){ throw new SimpleFractionException("Denominator cannot be 0"); }
private void reduceSimpleFractionToLowestTerms() { int gcd = GCD(Math.abs(num), Math.abs(den));
num = num / gcd;
den = den / gcd; }
/** Task: Computes the greatest common divisor of two integers. * @param integerOne an integer * @param integerTwo another integer * @return the greatest common divisor of the two integers */ private int GCD(int integerOne, int integerTwo) { int result;
if (integerOne % integerTwo == 0) result = integerTwo; else result = GCD(integerTwo, integerOne % integerTwo);
return result; } // end GCD
//----------------------------------------------------------------- // Simple test is provided here
public static void main(String[] args) { SimpleFractionInterface firstOperand = null; SimpleFractionInterface secondOperand = null; SimpleFractionInterface result = null; double doubleResult = 0.0;
SimpleFraction nineSixteenths = new SimpleFraction(9, 16); // 9/16 SimpleFraction oneFourth = new SimpleFraction(1, 4); // 1/4
System.out.println(" ========================================= "); // 7/8 + 9/16 firstOperand = new SimpleFraction(7, 8); result = firstOperand.add(nineSixteenths); System.out.println("The sum of " + firstOperand + " and " + nineSixteenths + " is \t\t" + result); System.out.println("\tExpected result :\t\t23/16 ");
// 9/16 - 7/8 firstOperand = nineSixteenths; secondOperand = new SimpleFraction(7, 8); result = firstOperand.subtract(secondOperand); System.out.println("The difference of " + firstOperand + " and " + secondOperand + " is \t" + result); System.out.println("\tExpected result :\t\t-5/16 ");
// 15/-2 * 1/4 firstOperand = new SimpleFraction(15, -2); result = firstOperand.multiply(oneFourth); System.out.println("The product of " + firstOperand + " and " + oneFourth + " is \t" + result); System.out.println("\tExpected result :\t\t-15/8 ");
// (-21/2) / (3/7) firstOperand = new SimpleFraction(-21, 2); secondOperand= new SimpleFraction(3, 7); result = firstOperand.divide(secondOperand); System.out.println("The quotient of " + firstOperand + " and " + secondOperand + " is \t" + result); System.out.println("\tExpected result :\t\t-49/2 ");
// -21/2 + 7/8 firstOperand = new SimpleFraction(-21, 2); secondOperand= new SimpleFraction(7, 8); result = firstOperand.add(secondOperand); System.out.println("The sum of " + firstOperand + " and " + secondOperand + " is \t\t" + result); System.out.println("\tExpected result :\t\t-77/8 ");
// 0/10, 5/(-15), (-22)/7 firstOperand = new SimpleFraction(0, 10); doubleResult = firstOperand.toDouble(); System.out.println("The double floating point value of " + firstOperand + " is \t" + doubleResult); System.out.println("\tExpected result \t\t\t0.0 "); firstOperand = new SimpleFraction(1, -3); doubleResult = firstOperand.toDouble(); System.out.println("The double floating point value of " + firstOperand + " is \t" + doubleResult); System.out.println("\tExpected result \t\t\t-0.333333333... "); firstOperand = new SimpleFraction(-22, 7); doubleResult = firstOperand.toDouble(); System.out.println("The double floating point value of " + firstOperand + " is \t" + doubleResult); System.out.println("\tExpected result \t\t\t-3.142857142857143"); System.out.println(" ========================================= "); firstOperand = new SimpleFraction(-21, 2); System.out.println("First = " + firstOperand); // equality check System.out.println("check First equals First: "); if (firstOperand.equals(firstOperand)) System.out.println("Identity of fractions OK"); else System.out.println("ERROR in identity of fractions");
secondOperand = new SimpleFraction(-42, 4); System.out.println(" Second = " + secondOperand); System.out.println("check First equals Second: "); if (firstOperand.equals(secondOperand)) System.out.println("Equality of fractions OK"); else System.out.println("ERROR in equality of fractions");
// comparison check SimpleFraction first = (SimpleFraction)firstOperand; SimpleFraction second = (SimpleFraction)secondOperand; System.out.println(" check First compareTo Second: "); if (first.compareTo(second) == 0) System.out.println("SimpleFractions == operator OK"); else System.out.println("ERROR in fractions == operator");
second = new SimpleFraction(7, 8); System.out.println(" Second = " + second); System.out.println("check First compareTo Second: "); if (first.compareTo(second) < 0) System.out.println("SimpleFractions < operator OK"); else System.out.println("ERROR in fractions < operator");
System.out.println(" check Second compareTo First: "); if (second.compareTo(first) > 0) System.out.println("SimpleFractions > operator OK"); else System.out.println("ERROR in fractions > operator");
System.out.println(" =========================================");
System.out.println(" check SimpleFractionException: 1/0"); try { SimpleFraction a1 = new SimpleFraction(1, 0); System.out.println("Error! No SimpleFractionException"); } catch ( SimpleFractionException fe ) { System.err.printf( "Exception: %s ", fe ); } // end catch System.out.println("Expected result : SimpleFractionException! ");
System.out.println(" check SimpleFractionException: division"); try { SimpleFraction a2 = new SimpleFraction(); SimpleFraction a3 = new SimpleFraction(1, 2); a3.divide(a2); System.out.println("Error! No SimpleFractionException"); } catch ( SimpleFractionException fe ) { System.err.printf( "Exception: %s ", fe ); } // end catch System.out.println("Expected result : SimpleFractionException! ");
} // end main } // end SimpleFraction
SimpleFractionException.java
public class SimpleFractionException extends RuntimeException { public SimpleFractionException() { this(""); }
public SimpleFractionException(String errorMsg) { super(errorMsg); }
}
SimpleFractionInterface.java /* This file specifies methods for SimpleFractionInterface */ /* Do not modify this file!! */
//package PJ1;
public interface SimpleFractionInterface { /** Task: Sets a fraction to a given value. * @param num is the integer numerator * @param den is the integer denominator * @throws ArithmeticException if denominator is 0 */ public void setSimpleFraction(int num, int den);
/** Task: convert a fraction to double value * @return the double floating point value of a fraction */ public double toDouble();
/** Task: Adds two fractions. * @param secondFraction is a fraction that is the second operand of the addition * @return a fraction which is the sum of the invoking fraction and the secondFraction */ public SimpleFractionInterface add(SimpleFractionInterface secondFraction);
/** Task: Subtracts two fractions. * @param secondFraction a fraction that is the second operand of the subtraction * @return a fraction which is the difference of the invoking fraction and the second operand */ public SimpleFractionInterface subtract(SimpleFractionInterface secondFraction);
/** Task: Multiplies two fractions. * @param secondFraction a fraction that is the second operand of the multiplication * @return a fraction which is the product of the invoking fraction and the secondFraction*/ public SimpleFractionInterface multiply(SimpleFractionInterface secondFraction);
/** Task: Divides two fractions. * @param secondFraction a fraction that is the second operand of the division * @return a fraction which the quotient of the invoking fraction and the secondFraction * @throws FractionException if secondFraction is 0 */ public SimpleFractionInterface divide(SimpleFractionInterface secondFraction);
/** Task: Get's the fraction's reciprocal * @return the reciprocal of the invoking fraction * @throws FractionException if the new number with denominator is 0*/ public SimpleFractionInterface getReciprocal();
}
PJ1_Test.java
import java.util.*; //import PJ1.*;
class PJ1_Test { static private Scanner scanner;
private static SimpleFraction readFraction() { System.out.print( " Try to read a fraction x/y, please enter x y : " ); int numerator = scanner.nextInt(); int denominator = scanner.nextInt(); SimpleFraction f = new SimpleFraction(numerator, denominator); System.out.println( "\t\tRead OK:"+f); return f; }
private static void printOperations() { System.out.println("=============================================="); System.out.println(" Operations:"); System.out.println(" 0) exit \t1) add \t\t2) subtract \t3) multiply \t4) divide"); System.out.println(" 5) compareTo \t6) equals \t7) recipocal \t8) toDouble \t9) setSimpleFraction (x/y) "); System.out.print( " Enter an operation number: "); }
public static void main( String args[] ) { scanner = new Scanner( System.in ); // scanner for input boolean continueLoop = true; // determines if more input is needed SimpleFraction n1=null; SimpleFraction n2=null; int op,x,y;
do { try // read two numbers and calculate quotient { printOperations(); op= scanner.nextInt();
if (op == 0) { break; } else if ((op >0) && (op <7)) { n1 = readFraction(); n2 = readFraction(); } else if ((op > 6) && (op < 9)) { n1 = readFraction(); } else if (op == 9) { n1 = new SimpleFraction(); } else { System.out.print( " Invalid input... try again " ); continue; }
System.out.println(" Tests: "); switch (op) { case 1: System.out.println("\t" + n1 + " + " + n1 + " = " + n1.add(n1)); System.out.println("\t" + n2 + " + " + n2 + " = " + n2.add(n2)); System.out.println("\t" + n1 + " + " + n2 + " = " + n1.add(n2)); System.out.println("\t" + n2 + " + " + n1 + " = " + n2.add(n1)); break; case 2: System.out.println("\t" + n1 + " - " + n1 + " = " + n1.subtract(n1)); System.out.println("\t" + n2 + " - " + n2 + " = " + n2.subtract(n2)); System.out.println("\t" + n1 + " - " + n2 + " = " + n1.subtract(n2)); System.out.println("\t" + n2 + " - " + n1 + " = " + n2.subtract(n1)); break; case 3: System.out.println("\t" + n1 + " * " + n1 + " = " + n1.multiply(n1)); System.out.println("\t" + n2 + " * " + n2 + " = " + n2.multiply(n2)); System.out.println("\t" + n1 + " * " + n2 + " = " + n1.multiply(n2)); System.out.println("\t" + n2 + " * " + n1 + " = " + n2.multiply(n1)); break; case 4: System.out.println("\t" + n1 + " / " + n1 + " = " + n1.divide(n1)); System.out.println("\t" + n2 + " / " + n2 + " = " + n2.divide(n2)); System.out.println("\t" + n1 + " / " + n2 + " = " + n1.divide(n2)); System.out.println("\t" + n2 + " / " + n1 + " = " + n2.divide(n1)); break; case 5: System.out.println("\t" + n1 + " ct " + n1 + " = " + n1.compareTo(n1)); System.out.println("\t" + n2 + " ct " + n2 + " = " + n2.compareTo(n2)); System.out.println("\t" + n1 + " ct " + n2 + " = " + n1.compareTo(n2)); System.out.println("\t" + n2 + " ct " + n1 + " = " + n2.compareTo(n1)); break; case 6: System.out.println("\t" + n1 + " eq "+ n1 + " = " + n1.equals(n1)); System.out.println("\t" + n2 + " eq "+ n2 + " = " + n2.equals(n2)); System.out.println("\t" + n1 + " eq "+ n2 + " = " + n1.equals(n2)); System.out.println("\t" + n2 + " eq "+ n1 + " = " + n2.equals(n1)); break; case 7: System.out.println("\t" + n1 + " getReciprocal= " + n1.getReciprocal()); break; case 8: System.out.println("\t" + n1 + " toDouble = " + n1.toDouble()); break; case 9: System.out.print( "read a fraction x/y, please enter x y : " ); x = scanner.nextInt(); y = scanner.nextInt(); System.out.print("\t" + x +"/"+ y + " setSimpleFraction = "); n1.setSimpleFraction(x,y); System.out.println(n1); break; }
} // end try catch ( SimpleFractionException fracitonException ) { System.err.printf( " Fraction Exception: %s ", fracitonException ); } // end catch } while ( continueLoop ); // end do...while } // end main } // end class
Step by Step Solution
There are 3 Steps involved in it
Step: 1
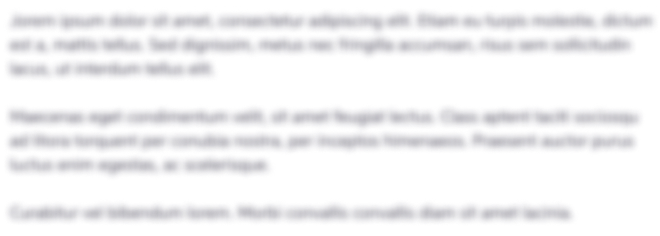
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started