Question
I need to create a C++ program that recreates a car dealership, with 3 different classes: vehicle, showroom, and dealership. I already have main.cpp, I
I need to create a C++ program that recreates a car dealership, with 3 different classes: vehicle, showroom, and dealership. I already have main.cpp, I just need the 3 classes.
The vehicle class will need to store data including the make of the vehicle, the model of the vehicle, the year, the price, and mileage. It needs to have these constructors and the functions defined. If dynamic memory is used, there should also be a destructor to clean up any memory allocated by the class.
The showroom clas should store an array of vehicle objects. Each showroom ceated should have a different number of vehicles, so dynamic memory allocation should be used. The showroom should contain variables for the name of the showroom, a pointer to the array of vehicles, a maximum capacity of the array, and a count of how many vehicles there are. There should also be the following functions, plus the special functios (a copy cunstructor, assignment operator and destructor).
The dealership class should store a dynamic array of showroom objects. It needs a name and ways to keep track of how many Showrooms it can store versus how many it is currently storing. It also needs a way to print everything in its inventory and a way to get the average price of each vehicle. It also needs the special functions - copy constructor, assigment operator, and destructor.
Here's main.cpp:
#include "Vehicle.h" #include "Showroom.h" #include "Dealership.h" #include
void TestOne(Vehicle *v); void TestTwo(Vehicle *v);
int main() { // Initialize some data. It's hard-coded here, but this data could come from a file, database, etc Vehicle vehicles[] = { Vehicle("Ford", "Mustang", 1973, 9500, 113000), Vehicle("Mazda", "CX-5", 2017, 24150, 5900), Vehicle("Dodge", "Charger", 2016, 18955, 9018), Vehicle("Telsa", "Model S", 2018, 74500, 31), Vehicle("Toyota", "Prius", 2015, 17819, 22987), Vehicle("Nissan", "Leaf", 2016, 12999, 16889), Vehicle("Chevrolet", "Volt", 2015, 16994, 12558), };
int testNum; cin >> testNum; if (testNum == 1) TestOne(vehicles); else if (testNum == 2) TestTwo(vehicles);
return 0; }
void TestOne(Vehicle *vehicles) { // Showrooms to store the vehicles Showroom showroom("Primary Showroom", 3); showroom.AddVehicle(&vehicles[0]); showroom.AddVehicle(&vehicles[1]); //showroom.AddVehicle(&vehicles[2]); Showroom secondary("Fuel-Efficient Showroom", 4); secondary.AddVehicle(&vehicles[3]); secondary.AddVehicle(&vehicles[4]); secondary.AddVehicle(&vehicles[5]); secondary.AddVehicle(&vehicles[6]);
// A "parent" object to store the Showrooms Dealership dealership("COP3503 Vehicle Emporium", 2); dealership.AddShowroom(&showroom); dealership.AddShowroom(&secondary);
dealership.ShowInventory(); }
void TestTwo(Vehicle *vehicles) { // Showrooms to store the vehicles Showroom showroom("Primary Showroom", 3); showroom.AddVehicle(&vehicles[0]); showroom.AddVehicle(&vehicles[1]); Showroom secondary("Fuel-Efficient Showroom", 4);
secondary.AddVehicle(&vehicles[4]); secondary.AddVehicle(&vehicles[5]); Showroom third("Fuel-Efficient Showroom", 4); third.AddVehicle(&vehicles[3]); // A "parent" object to store the Showrooms Dealership dealership("COP3503 Vehicle Emporium", 3); dealership.AddShowroom(&showroom); dealership.AddShowroom(&secondary); dealership.AddShowroom(&third);
cout
cout // Print all the information on a single line void Display() const; //Return a string in the form of "1970 Ford Mustang" string GetYearMakeModel() const; //How much to buy this? float GetPrice() const
Step by Step Solution
There are 3 Steps involved in it
Step: 1
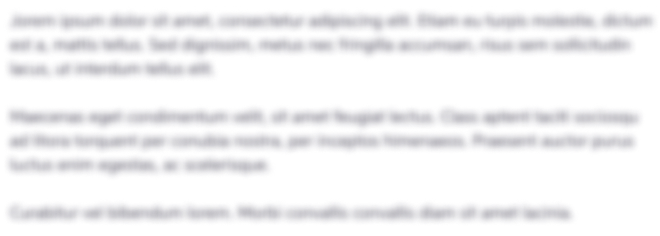
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started