Question
I need to create a running total class, any suggestions on how to create one? I have been trying many different ways but I cant
I need to create a running total class, any suggestions on how to create one? I have been trying many different ways but I cant seem to create a class to work. These are the classes I have so far.
import javax.swing.*; // Needed for Swing classes import java.awt.event.*; // Needed for ActionListener Interface import javax.swing.event.*; import java.util.Random; import java.awt.*; import javax.swing.JOptionPane;
public class Final extends JFrame { private double total; private double total2; private double total3;
private final int sides = 3; private int value; private int value2; private int value3; private JPanel panel; private JLabel endLabel; private JLabel AmountLabel; private JLabel totalAmount; private JLabel imageLabel1; private JLabel imageLabel2; private JLabel imageLabel3; private JTextField EnteredMoney; private JTextField endMoney; private JTextField totalMoney; private JButton Amount; private final int WINDOW_WIDTH = 5000; private final int WINDOW_HEIGHT = 300;
public NatoliFinal() { setTitle("Dice Simulator");
setSize(WINDOW_WIDTH, WINDOW_HEIGHT);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
buildPanel();
setVisible(true); }
public void buildPanel() { AmountLabel = new JLabel("Amount Inserted: $");
EnteredMoney = new JTextField(10);
endLabel = new JLabel("You have won: $");
endMoney = new JTextField(10);
endMoney.setEditable(false);
totalAmount = new JLabel("Total amount won is");
totalMoney = new JTextField(10);
totalMoney.setEditable(false);
imageLabel1 = new JLabel();
imageLabel2 = new JLabel();
imageLabel3 = new JLabel();
Amount = new JButton("Spin");
Amount.addActionListener(new AmountListener());
JPanel panel = new JPanel(); JPanel panel2 = new JPanel(); JPanel panel3 = new JPanel(); JPanel panel4 = new JPanel();
panel = new JPanel(); panel2 = new JPanel(); panel3 = new JPanel(); panel4 = new JPanel();
panel.add(AmountLabel); panel.add(EnteredMoney); panel.add(endLabel); panel.add(endMoney); panel.add(totalAmount); panel.add(totalMoney); panel2.add(imageLabel1); panel2.add(imageLabel2); panel4.add(imageLabel3); panel3.add(Amount);
add(panel,BorderLayout.WEST); add(panel2,BorderLayout.NORTH); add(panel3,BorderLayout.SOUTH); add(panel4,BorderLayout.EAST);
}
private class AmountListener implements ActionListener { public void actionPerformed(ActionEvent e) {
NatoliSlot Roll = new NatoliSlot();
value = Roll.getValue(); value2 = Roll.getValue2(); value3 = Roll.getValue3();
String Emoney =""; double eMoney = 0.0;
double Doubel =0.0;
Emoney = EnteredMoney.getText();
eMoney = Double.parseDouble(Emoney);
switch(value) { case 1 : ImageIcon set = new ImageIcon("cherry.jpg"); imageLabel1.setIcon(set); break;
case 2: ImageIcon set2 = new ImageIcon("lemon.png"); imageLabel1.setIcon(set2); break;
case 3: ImageIcon set3 = new ImageIcon("watermelon.jpg"); imageLabel1.setIcon(set3); break;
}//end of vlaue switch
switch(value2) { case 1 : ImageIcon set = new ImageIcon("cherry.jpg"); imageLabel2.setIcon(set); break;
case 2: ImageIcon set2 = new ImageIcon("lemon.png"); imageLabel2.setIcon(set2); break;
case 3: ImageIcon set3 = new ImageIcon("watermelon.jpg"); imageLabel2.setIcon(set3); break;
}// end of value2 switch
switch(value3) { case 1 : ImageIcon set = new ImageIcon("cherry.jpg"); imageLabel3.setIcon(set); break;
case 2: ImageIcon set2 = new ImageIcon("lemon.png"); imageLabel3.setIcon(set2); break;
case 3: ImageIcon set3 = new ImageIcon("watermelon.jpg"); imageLabel3.setIcon(set3); break; }// end of value3 switch
DoubleScore D = new DoubleScore(); total3 = D.setNum(eMoney,value,value2,value3); endMoney.setText(String.format("%f",(total3)));
totalMoney.setText(String.format("%f",(total3)));
}
}
public static void main(String [] args) { new Final(); }
}
import java.util.Random; public class Slot { private int sides =3; private int value; private int value2; private int value3;
public Slot() {
roll();
} // Storing sides into value public void roll( ) { Random rand = new Random(); value = rand.nextInt(sides)+1; value2 = rand.nextInt(sides)+1; value3 = rand.nextInt(sides)+1;
}
public void setvalue(int Value) { value = Value; }
public void setvalue2(int Value2) { value2 = Value2; }
public void setvalue3(int Value3) { value3 = Value3; }
public int getValue() { return value; }
public int getValue2() { return value2; }
public int getValue3() { return value3; }
}//End of class
public class DoubleScore { private double num; private int value; private int value2; private int value3;
public DoubleScore() {
}
public double setNum(double total, int value, int value2, int value3) {
if (value == 1 && value2 ==1 && value3==1) { num = Math.pow(total, 3); }
else if(value == 2 && value2 ==2 && value3 == 2) { num = Math.pow(total, 3); }
else if(value ==3 && value2 ==3 && value3 ==3) { num = Math.pow(total, 3); } if(value ==1 && value2 ==1 && value3 == 2) { num = Math.pow(total, 2); } else if(value ==1 && value2 ==1 && value3 == 3) { num = Math.pow(total, 2); } if(value ==1 && value3 ==1 && value2 == 2) { num = Math.pow(total, 2); } else if(value ==1 && value3 ==1 && value2 == 3) { num = Math.pow(total, 2); } if(value2 ==1 && value3 ==1 && value == 2) { num = Math.pow(total, 2); } else if(value2 ==1 && value3 ==1 && value == 3) { num = Math.pow(total, 2); } if(value ==2 && value2 ==2 && value3 ==1) { num = Math.pow(total, 2); } else if(value ==2 && value2 ==2 && value3 == 3) { num = Math.pow(total, 2); } if(value ==2 && value3 ==2 && value2 == 1) { num = Math.pow(total, 2); } else if(value ==2 && value3 ==2 && value2 == 3) { num = Math.pow(total, 2); } if(value2 ==2 && value3 ==2 && value == 3) { num = Math.pow(total, 2); } else if(value2 ==2 && value3 ==2 && value ==1) { num = Math.pow(total, 2); } if(value ==3 && value2 ==3 && value3 == 1) { num = Math.pow(total, 2); } else if(value ==3 && value2 ==3 && value3 == 2) { num = Math.pow(total, 2); } if(value ==3 && value3 ==3 && value2 == 1) { num = Math.pow(total, 2); } else if(value ==3 && value3 ==3 && value2 == 2) { num = Math.pow(total, 2); } if(value2 ==3 && value3 ==3 && value == 1) { num = Math.pow(total, 2); } if(value2 ==3 && value3 ==3 && value == 2) { num = Math.pow(total, 2); }
return num;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
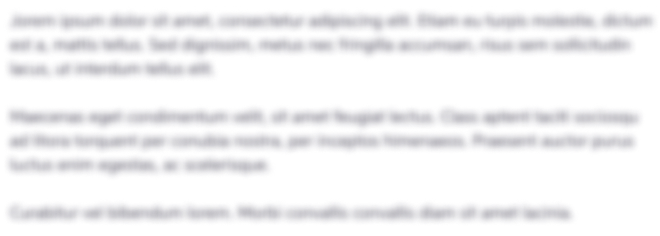
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started