Question
I need your help:Using Java You are to write a program to simulate the counter activity at a deli. The actions that can be taken
I need your help:Using Java
You are to write a program to simulate the counter activity at a deli. The actions that can be taken are given by the String[] in the DeliCounter class provided. Only the major components of the classes you are to write will be provided; other requirements will have to be derived and provided based on implied program behavior.
Write the following classes
MenuItem
Write an abstract class MenuItem. The delis menu is, simply, a collection of MenuItem objects. All MenuItems have a name and a price. Well assume that all names are a single word (no embedded spaces) and all prices can be represented as a double value. MenuItem has an abstract method, boolean isInventory(). More on inventory below.
PackagedItem
Some items on the delis menu are pre-packaged and considered inventory; bottles of water, cans of soft drinks, bags of chips are examples. These items, in addition to a name and unit price, have a quantity. For example, the deli may have 50 bags of chips on hand. The quantity must be adjusted as items are sold. (We wont worry about restocking for this program.) The owner of the shop wants to know the total value of his packaged inventory items.
PreparedItem
Some items on the delis menu must be prepared for each order. Such items are not considered to be inventory. In addition to a name and price, PreparedItems have a preparation time that is kept in minutes.
Additional Program Capability
Initially, the deli program starts with an empty menu. It should be able to save and restore the menu on demand. It should be able to offer customers the list of items that may be ordered, sell an item, add new items to the menu, and list the current value of the packaged items.
Grading Elements
MenuItem is an abstract class representing any menu item, it contains the isInventory method.
PackagedItem, PreparedItem are classes that represent specific types of menu items, minimize their local class members, and exploit their superclass as much as possible.
DeliCounter program supports all user operations.
All class members are marked public or private, as appropriate. Code follows the Java naming conventions, is in the default package, submission is a single zip file with no directories.
<<<<<
The following is a sample execution.
[0] Restore Inventory
[1] Save Inventory
[2] List Total Packaged Item Value
[3] List Menu
[4] Order Item
[5] Add New Package
[6] Add New Cooked Item
[7] Exit
3
[0] Restore Inventory
[1] Save Inventory
[2] List Total Packaged Item Value
[3] List Menu
[4] Order Item
[5] Add New Package
[6] Add New Cooked Item
[7] Exit
5
Add a new packaged item:
Item name:
chips
Item price:
1.50
Item quantity:
10
[0] Restore Inventory
[1] Save Inventory
[2] List Total Packaged Item Value
[3] List Menu
[4] Order Item
[5] Add New Package
[6] Add New Cooked Item
[7] Exit
6
Add a new cooked item:
Item name:
Ham&Swiss
Item price:
3.50
Item cooking time:
11
[0] Restore Inventory
[1] Save Inventory
[2] List Total Packaged Item Value
[3] List Menu
[4] Order Item
[5] Add New Package
[6] Add New Cooked Item
[7] Exit
5
Add a new packaged item:
Item name:
Coke
Item price:
2.00
Item quantity:
20
[0] Restore Inventory
[1] Save Inventory
[2] List Total Packaged Item Value
[3] List Menu
[4] Order Item
[5] Add New Package
[6] Add New Cooked Item
[7] Exit
6
Add a new cooked item:
Item name:
HotDog
Item price:
2.50
Item cooking time:
3
[0] Restore Inventory
[1] Save Inventory
[2] List Total Packaged Item Value
[3] List Menu
[4] Order Item
[5] Add New Package
[6] Add New Cooked Item
[7] Exit
2
Total value of packaged items is 55.0
[0] Restore Inventory
[1] Save Inventory
[2] List Total Packaged Item Value
[3] List Menu
[4] Order Item
[5] Add New Package
[6] Add New Cooked Item
[7] Exit
3
[0] chips
[1] Ham&Swiss
[2] Coke
[3] HotDog
[0] Restore Inventory
[1] Save Inventory
[2] List Total Packaged Item Value
[3] List Menu
[4] Order Item
[5] Add New Package
[6] Add New Cooked Item
[7] Exit
4
Which item number do you want?
0
You purchased chips
The price is 1.5
[0] Restore Inventory
[1] Save Inventory
[2] List Total Packaged Item Value
[3] List Menu
[4] Order Item
[5] Add New Package
[6] Add New Cooked Item
[7] Exit
2
Total value of packaged items is 53.5
[0] Restore Inventory
[1] Save Inventory
[2] List Total Packaged Item Value
[3] List Menu
[4] Order Item
[5] Add New Package
[6] Add New Cooked Item
[7] Exit
1
[0] Restore Inventory
[1] Save Inventory
[2] List Total Packaged Item Value
[3] List Menu
[4] Order Item
[5] Add New Package
[6] Add New Cooked Item
[7] Exit
3
[0] chips
[1] Ham&Swiss
[2] Coke
[3] HotDog
[0] Restore Inventory
[1] Save Inventory
[2] List Total Packaged Item Value
[3] List Menu
[4] Order Item
[5] Add New Package
[6] Add New Cooked Item
[7] Exit
4
Which item number do you want?
2
You purchased Coke
The price is 2.0
[0] Restore Inventory
[1] Save Inventory
[2] List Total Packaged Item Value
[3] List Menu
[4] Order Item
[5] Add New Package
[6] Add New Cooked Item
[7] Exit
2
Total value of packaged items is 51.5
[0] Restore Inventory
[1] Save Inventory
[2] List Total Packaged Item Value
[3] List Menu
[4] Order Item
[5] Add New Package
[6] Add New Cooked Item
[7] Exit
0
[0] Restore Inventory
[1] Save Inventory
[2] List Total Packaged Item Value
[3] List Menu
[4] Order Item
[5] Add New Package
[6] Add New Cooked Item
[7] Exit
2
Total value of packaged items is 53.5
[0] Restore Inventory
[1] Save Inventory
[2] List Total Packaged Item Value
[3] List Menu
[4] Order Item
[5] Add New Package
[6] Add New Cooked Item
[7] Exit
3
[0] chips
[1] Ham&Swiss
[2] Coke
[3] HotDog
[0] Restore Inventory
[1] Save Inventory
[2] List Total Packaged Item Value
[3] List Menu
[4] Order Item
[5] Add New Package
[6] Add New Cooked Item
[7] Exit
4
Which item number do you want?
3
You purchased HotDog
The price is 2.5
[0] Restore Inventory
[1] Save Inventory
[2] List Total Packaged Item Value
[3] List Menu
[4] Order Item
[5] Add New Package
[6] Add New Cooked Item
[7] Exit
7
----------DeliCounter-----
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.util.ArrayList;
import java.util.Scanner;
public class DeliCounter {
public static final String[] ACTIONS = { "Restore Inventory", "Save Inventory", "List Total Packaged Item Value",
"List Menu", "Order Item", "Add New Package", "Add New Cooked Item", "Exit" };
public static void main(String[] args) {
ArrayList
Scanner kybd = new Scanner(System.in);
DeliCounter deli = new DeliCounter();
displayMenu(ACTIONS);
int activity = kybd.nextInt();
while (!ACTIONS[activity].equalsIgnoreCase("Exit")) {
if (ACTIONS[activity].equalsIgnoreCase("Restore Inventory")) {
menu = restoreInventory();
} else if (ACTIONS[activity].equalsIgnoreCase("Save Inventory")) {
saveInventory(menu);
} else if (ACTIONS[activity].equalsIgnoreCase("List Total Packaged Item Value")) {
displayPackageValue(menu);
} else if (ACTIONS[activity].equalsIgnoreCase("List Menu")) {
displayMenu(menu);
} else if (ACTIONS[activity].equalsIgnoreCase("Order Item")) {
System.out.println("Which item number do you want? ");
int itemNum = kybd.nextInt();
orderItem(itemNum, menu);
} else if (ACTIONS[activity].equalsIgnoreCase("Add New Package")) {
MenuItem newItem = newPackagedItem(kybd);
menu.add(newItem);
} else if (ACTIONS[activity].equalsIgnoreCase("Add New Cooked Item")) {
MenuItem newItem = addCooked(kybd);
menu.add(newItem);
} else {
System.out.println("Invalid input ignored");
}
displayMenu(ACTIONS);
activity = kybd.nextInt();
}
}
private static MenuItem addCooked(Scanner kybd) {
System.out.println("Add a new cooked item: ");
System.out.println("Item name:");
String name= kybd.next();
System.out.println("Item price:");
double pri=kybd.nextDouble();
System.out.println("Item cooking time:");
int min=kybd.nextInt();
return new PreparedItem(name,pri,min);
}
private static MenuItem newPackagedItem(Scanner kybd) {
System.out.println("Add a new packaged item: ");
System.out.println("Item name:");
String name= kybd.next();
System.out.println("Item price:");
double pri=kybd.nextDouble();
System.out.println("Item quantity:");
int quantity=kybd.nextInt();
return new PackagedItem(name,pri,quantity);
}
private static void orderItem(int itemNum, ArrayList
String st="";
double priceItem=0;
for (int i=0;i
if (itemNum==i)
st=st+menu.get(i).toString();
priceItem=menu.get(i).getPrice();
}
System.out.println("Yoy purchaced "+st);
System.out.println("The price is "+priceItem+" ");
}
private static void displayMenu(ArrayList
System.out.println(" ");
String s="";
for (MenuItem item:items) {
s=s+item.toString()+" ";
}
System.out.println(s);
}
private static void displayPackageValue(ArrayList
double totalPrice=0;
for (MenuItem item:menu) {
totalPrice=totalPrice+item.getTotal();
}
System.out.println("Total value of packeged item is "+totalPrice);
}
private static void saveInventory(ArrayList
}
private static ArrayList
return null;
}
public static void displayMenu(String[] items) {
for (int i = 0; i < items.length; i++) {
System.out.println("[" + i + "] " + items[i]);
}}}
-------MenuItem----
// stub to help DeliCounter compile initially
public abstract class MenuItem {
private String name;
private double price;
private double total;
protected MenuItem(String itemName,double itemPrice) {
this.setName(itemName);
this.setPrice(itemPrice);
}
public abstract boolean isInventory();
/**
* @return the price
*/
public double getPrice() {
return price;
}
/**
* @param price the price to set
*/
public void setPrice(double price) {
this.price = price;
}
/**
* @return the name
*/
public String getName() {
return name;
}
/**
* @param name the name to set
*/
public void setName(String name) {
this.name = name;
}
public double getTotal() {
total = total+getPrice();
return total;
}
@Override
public String toString() {
return "MenuItem [ " + name + "]";
}}
-------PackageItem-----
public class PackagedItem extends MenuItem {
private double total;
private int quantity;
protected PackagedItem(String itemName, double itemPrice,int quantity) {
super(itemName, itemPrice);
this.setQuantity(quantity);
}
@Override
public boolean isInventory() {
return false;
}
/**
* @return the quantity
*/
public int getQuantity() {
return quantity;
}
/**
* @param quantity the quantity to set
*/
public void setQuantity(int quantity) {
this.quantity = quantity;
}
public double getTotal() {
total = total+super.getPrice();
return total;
}
}
-------PreparedItem-----
public class PreparedItem extends MenuItem {
private double total;
private int minutes;
protected PreparedItem(String itemName, double itemPrice,int min) {
super(itemName, itemPrice);
this.setMinutes(min);
}
@Override
public boolean isInventory() {
return false;
}
public int getMinutes() {
return minutes;
}
public void setMinutes(int minutes) {
this.minutes = minutes;
}
public double getTotal() {
total = total+super.getPrice();
return total;
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
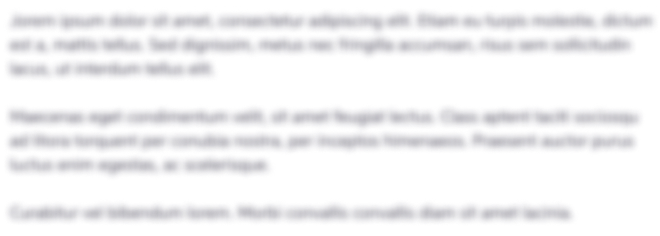
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started