Question
I put the code for part 1, I need help for part 2 Driver.java import publications.PaperPublication; import book.Book; import book.ChildrenBook; import book.EducationBook; import journal.Journal; import
I put the code for part 1, I need help for part 2
Driver.java
import publications.PaperPublication; import book.Book; import book.ChildrenBook; import book.EducationBook; import journal.Journal; import magazine.Magazine;
public class Driver {
public static void main(String[] args) { // TODO Auto-generated method stub //displaying to the user what the program is about to do System.out.println("Will create objects from each" + " of the 6 classes."); //creating objects from the classes PaperPublication p1 = new PaperPublication(), p2 = new PaperPublication(2.0, 5), p3 = new PaperPublication(5.0, 50); Book p4 = new Book(15.0,200,0,1990,"Made up Title","Alan Smithee"), p5 = new Book(15.0, 200, 0, 1990, "Made up Title", "Alan Smithee"), p6 = new Book(1.0, 100, 0, 2000, "1984", "George Orwell"); ChildrenBook p7 = new ChildrenBook(); EducationBook p8 = new EducationBook(299.99,300,9499831,300, "How to Sell Overpriced Textbooks to Poor Students","Greedy McSlimeface",20,"Advanced Juggling"); Journal p9 = new Journal(39.99,40,42,"Hypothetical Cat Juggling"); Magazine p10 = new Magazine(); //display the information of the objects using the toString() method System.out.println("The first PaperPublication: " + p1); System.out.println("The second PaperPublication: " + p2); System.out.println("The third PaperPublication: " + p3); System.out.println("The fourth PaperPublication: " + p4); System.out.println("The fifth PaperPublication: " + p5); System.out.println("The six PaperPublication: " + p6); System.out.println("The seventh PaperPublication: " + p7); System.out.println("The eight PaperPublication: " + p8); System.out.println("The nineth PaperPublication: " + p9); System.out.println("The tenth PaperPublication: " + p10); System.out.println(); //testing of equality of some objects using the equals() method if(p1.equals(p2)) System.out.println("The two PaperPublications p1 & p2 are equal"); else System.out.println("The two PaperPublications p1 & p2 are not equal"); if(p1.equals(p2)) System.out.println("The two Books b1 & b2 are equal"); else System.out.println("The two Books b1 & b2 are not equal"); //creating an object array PaperPublication[] publicationsArray = {p1,p2,p3,p4,p5,p6,p7,p8,p9,p10}; System.out.println(); //finding the cheapest price in the array //Find the cheapest book and display its information double cheapest = publicationsArray[0].getPrice(); int cheapestIndex = 0; for(int i = 0;i
}
}
PaperPublication.java
package publications;
public class PaperPublication {
double price; int number_of_pages; //Default constructor with not parameters initialises attributes to 0 public PaperPublication(){ this(0,0); } //Parametrised constructor takes price as argument public PaperPublication(double price){ this(price, 0); } //Parametrised constructor takes price and number_of_pages public PaperPublication(double price, int number_of_pages){ this.price = price; this.number_of_pages = number_of_pages; } //toString method returns values of attributes public String toString(){ return "This paper publication has " + number_of_pages + " pages and costs $" + price; } //equals method returns true iff all attributes between compared objects are the same //equals must be overridden as it is inherited from the Object class public boolean equals(Object publication){ PaperPublication castPublication = (PaperPublication) publication; //type cast parameter into PaperClass class if(this.price == castPublication.price && this.number_of_pages == castPublication.number_of_pages){ return true; } return false; } //getPrice returns price of object public double getPrice(){ return this.price; } //setPrice sets new price public void setPrice(double price){ this.price = price; } //getNumber_of_pages returns number_of_pages public int getNumber_of_pages(){ return this.number_of_pages; } //setNumber_of_pages sets new number_of_pages public void setNumber_of_pages(int number_of_pages){ this.number_of_pages = number_of_pages; } }
ChildrenBook.java
package book; public class ChildrenBook extends Book { public int min_age; //Default Constructor// public ChildrenBook(){ super(); this.min_age= 0; }
//Parametrized Constructor// public ChildrenBook (double price, int number_of_pages, long isbn, int issueYear, String title, String authorName, int min_age){ super(price,number_of_pages,isbn,issueYear,title, authorName); this.min_age=min_age; } //Accessor Method public int getMin_age(){ return min_age; } //Mutator Method// public void setMin_Age(int min_age){ this.min_age=min_age; } //ToString Method public String toString(){ return super.toString() + " It is suitable for ages "+min_age+" and up."; } //equals method returns true iff all attributes between compared objects are the same //equals must be overridden as it is inherited from the Object class public boolean equals(Object publication){ if(publication == null){ //make sure the parameter is not null return false; } else if(getClass() != publication.getClass()) //make sure the parameter is the same class as the compared object { return false; } else{ ChildrenBook book = (ChildrenBook) publication; //type cast parameter into ChildrenBook class if(this.getPrice() == book.getPrice() && this.getNumber_of_pages() == book.getNumber_of_pages() && this.isbn == book.isbn && this.issueYear == book.issueYear && this.title.equalsIgnoreCase(book.title) && this.authorname == book.authorname && this.min_age == book.min_age){ return true; } } return false; } }
Book.java
package book;
import publications.PaperPublication;
public class Book extends PaperPublication {
protected long isbn; protected int issueYear; protected String title; protected String authorname; //Default Constructor set parameters to null// public Book (){ super(); this.isbn = 0; this.issueYear= 0; this.title = null; this.authorname = null; } // Parametrised Constructor // public Book (double price, int number_of_pages, long isbn, int issueYear, String title, String authorname){
super(price, number_of_pages); this.isbn = isbn; this.issueYear= issueYear; this.title = title; this.authorname = authorname; } //returns value of isbn// public long getisbn(){ return this.isbn; } //Mutator for the isbn// public void setisbn(long isbn){ this.isbn = isbn; } //returns value of the issue year// public int getissueYear (){ return this.issueYear; } //mutator for the Issue Year// public void setissueYear (int issueYear){ this.issueYear = issueYear; } //returns the title// public String gettitle(){ return this.title; } //mutator for the title of book// public void settitle(String title){ this.title = title; } //Returns the name of the author// public String getauthorname (){ return this.authorname; } //mutator for the name of the author// public void setauthorname(String authorname){ this.authorname = authorname; } //considering if a period is entered in original PaperPublication class// public String toString(){ return super.toString() + " This book is titled \" " + title + " \", by " + authorname + ", was issued in " + issueYear + " and its isbn is "+ isbn+"."; } //equals method returns true iff all attributes between compared objects are the same //equals must be overridden as it is inherited from the Object class public boolean equals(Object publication){ Book book = (Book) publication; //type cast parameter into Book class if(this.getPrice() == book.getPrice() && this.getNumber_of_pages() == book.getNumber_of_pages() && this.isbn == book.isbn && this.issueYear == book.issueYear && this.title.equalsIgnoreCase(book.title) && this.authorname == book.authorname){ return true; } return false; } }
EducationBook.java
package book; public class EducationBook extends Book { protected int editionNum; protected String specialty_field;
//Default Constructor public EducationBook(){ super(); this.editionNum = 0; this.specialty_field = null; }
//Parametrized constructor public EducationBook(double price, int number_of_pages, long Isbn, int IssueYear, String Title, String AuthorName,int editionNum, String specialty_field){ super(price, number_of_pages, Isbn, IssueYear, Title, AuthorName); this.editionNum = editionNum; this.specialty_field = specialty_field; }
//Accessors
public int getEditionNum(){ return this.editionNum; }
public String getSpecialtyField(){ return this.specialty_field; }
//Mutators
public void setEditionNum(int editionNum){ editionNum = this.editionNum; }
public void setSpecialtyField(String specialty_field){ specialty_field = this.specialty_field; }
//equals method returns true iff all attributes between compared objects are the same //equals must be overridden as it is inherited from the Object class public boolean equals(Object publication){ EducationBook book = (EducationBook) publication; //type cast parameter into EducationBook class if(this.getPrice() == book.getPrice() && this.getNumber_of_pages() == book.getNumber_of_pages() && this.isbn == book.isbn && this.issueYear == book.issueYear && this.title.equalsIgnoreCase(book.title) && this.authorname == book.authorname && this.editionNum == book.editionNum && this.specialty_field == book.specialty_field){ return true; } return false; } //toString method
public String toString(){ return super.toString() + "The edition number is: " + this.editionNum + " and the specialty field is: " + this.specialty_field + "."; } }
Magazine.java
package magazine;
import publications.PaperPublication;
public class Magazine extends PaperPublication {
enum PaperQuality { LOW, MEDIUM, HIGH; } enum IssuingFrequency { WEEKLY, MONTHLY, YEARLY; }
PaperQuality paperQuality; IssuingFrequency issuingFrequency;
//Default Constructor public Magazine() { super(); this.paperQuality = null; this.issuingFrequency = null; }; //Parametrized Constructor that will take Paper Quality and Issuing Frequency as a parameter public Magazine(double price,int number_of_pages,PaperQuality paperQuality, IssuingFrequency issuingFrequency){ super(price,number_of_pages); this.paperQuality=paperQuality; this.issuingFrequency = issuingFrequency; }; //Accessors public String getPaperQuality(){ return this.paperQuality.toString(); };
public String getIssueFrequency(){ return this.issuingFrequency.toString(); }; //Mutators public void setPaperQuality(PaperQuality paperQuality){ paperQuality=this.paperQuality; }; public void setIssuingFrequency(IssuingFrequency issuingFrequency){ issuingFrequency=this.issuingFrequency; };
//toString method returns values of Magazine attributes public String toString(){ return super.toString() + "The paper quality of this magazine is " + paperQuality + "." + "It is issued " + issuingFrequency + "."; }
//equals method returns true iff all attributes between compared objects are the same //equals must be overridden as it is inherited from the Object class public boolean equals(Object publication){ Magazine magazine = (Magazine) publication; //type cast parameter into Magazine class if(this.getPrice() == magazine.getPrice() && this.getNumber_of_pages() == magazine.getNumber_of_pages() && this.paperQuality == magazine.paperQuality && this.issuingFrequency == magazine.issuingFrequency){ return true; } return false; }
}
Journal.java
package journal;
import publications.PaperPublication;
public class Journal extends PaperPublication{ private int issue_number; private String specialty_field; //Default constructor public Journal(){ super(); issue_number = 0; specialty_field = "None"; }
//Overloaded constructor takes issue_number as parameter public Journal(double price, int number_of_pages, int issue_number){ super(price, number_of_pages); this.issue_number = issue_number; }
//Overloaded constructor takes issue_number and specialty_field as parameters public Journal(double price, int number_of_pages, int issue_number, String specialty_field){ super(price, number_of_pages); this.issue_number = issue_number; this.specialty_field = specialty_field; } //toString method returns values of attributes public String toString(){ return "This is issue No. " + issue_number + " of the Journal of " + specialty_field + ". " + "It has a price of $" + this.getPrice() + " and " + "the total number of pages is " + this.getNumber_of_pages() +"."; } //equals method returns true iff all attributes between compared objects are the same //equals must be overridden as it is inherited from the Object class public boolean equals(Object publication){ Journal journalPublication = (Journal) publication; //type cast parameter into Journal class if(this.getPrice() == journalPublication.getPrice() && this.getNumber_of_pages() == journalPublication.getNumber_of_pages() && this.issue_number == journalPublication.issue_number && specialty_field.equalsIgnoreCase(journalPublication.specialty_field)){ return true; } return false; }
//accessors and mutators return or set new values for attributes public int getIssue(){ return this.issue_number; } public void setIssue(int issue_number){ this.issue_number = issue_number; } public String getSpecialty(){ return this.specialty_field; } public void setSpecialty(String specialty_field){ this.specialty_field = specialty_field; } }
Various publications can be described as follows: A PaperPublication class with the following: a tle (String type), price (double type) and number of pages (int type) A Book is a PaperPublication that in addition has the following: an ISBN (long type), an issue year (int type), and author(s)name (String type) - A ChildrenBook is a Book that in addition has the following: minimum age (int type), which indicates the minimum age that this book is expected for. An EducationalBook is a Book that in addition it has the following: edition number (int type) and speciality field (String type), such as pharmaceutical, engineering, commerce, etc. A Journal is a PaperPublication that in addition has the following: issue number (int type) and speciality field (String type), such as pharmaceutical, engineering, commerce, etc. A Magazine is a PaperPublication that in addition has the following: paper quality (enumeration type that can be: High, Normal, or Low), and issuing frequency (enumeration type that can be: Weekly, Monthly, or Yearly). Various publications can be described as follows: A PaperPublication class with the following: a tle (String type), price (double type) and number of pages (int type) A Book is a PaperPublication that in addition has the following: an ISBN (long type), an issue year (int type), and author(s)name (String type) - A ChildrenBook is a Book that in addition has the following: minimum age (int type), which indicates the minimum age that this book is expected for. An EducationalBook is a Book that in addition it has the following: edition number (int type) and speciality field (String type), such as pharmaceutical, engineering, commerce, etc. A Journal is a PaperPublication that in addition has the following: issue number (int type) and speciality field (String type), such as pharmaceutical, engineering, commerce, etc. A Magazine is a PaperPublication that in addition has the following: paper quality (enumeration type that can be: High, Normal, or Low), and issuing frequency (enumeration type that can be: Weekly, Monthly, or Yearly)Step by Step Solution
There are 3 Steps involved in it
Step: 1
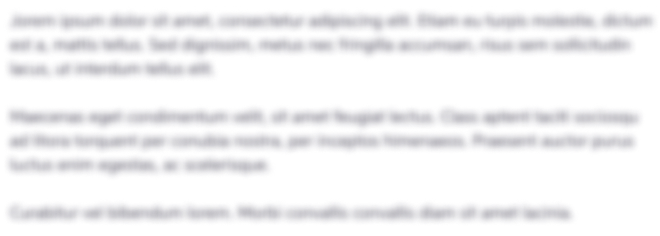
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started