Question
I was given a Class vector: #include #ifndef VECTOR_H #define VECTOR_H class Vector { public: Vector(); //default constructor Vector(int s); //makes size = s //allocate
I was given a Class vector:
#include
#ifndef VECTOR_H #define VECTOR_H
class Vector {
public: Vector(); //default constructor Vector(int s); //makes size = s //allocate s space //makes all entries 0 Vector(const Vector & other); //copy constructor //makes a deep copy ~Vector(); //default destructor void print(); //Prints out the vector
void get(int val, int pos); //Prints out the vector Vector operator+ (const Vector &other) const;
Vector operator= (const Vector & other);
private: int size; //sets the # of elements used int *entries; //point to array of integers with size entries //e.g. entries = new int[size] };
#endif
I was also given a main function or drover function:
#include "Vector.h"
#include
using namespace std;
int main() { Vector a, b(3), c(3); b.get(); // user enters 1 2 3 c.get(); // user enters -2 1 0
a = b + c; cout << "The sum of "; b.print();
cout << " and "; c.print();
cout << " is "; a.print();
return 0; }
I was able to implement the class. I have the implemnation below, but I cant seem to implement some of the the member functions (such as get and the operators) and need help. This is what I have so far:
#include "Vector.h"
#include
using namespace std;
Vector::Vector() { size = 0; entries = new int[size]; for (int i = 0; i < size; i++) { entries[i] = 0; }
}
Vector::Vector(int s) { size = s; entries = new int[size]; for (int i = 0; i < size; i++) { entries[i] = 0; } }
Vector::Vector(const Vector & other) { this->size = other.size; this->entries = new int[size]; for (int i = 0; i < size; i++) { this->entries[i] = other.entries[i]; } }
Vector::~Vector() { this->entries = NULL; }
void Vector::print() { cout << endl << "["; for (int i = 0; i < size; i++) { cout << entries[i] << ""; }
cout << "]" << endl; }
void Vector::get() { }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
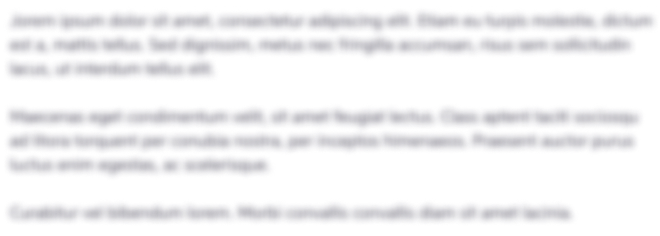
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started