Question
I worked my solution but i need assistance in making sure i did the right thing if there is a place where i made a
I worked my solution but i need assistance in making sure i did the right thing if there is a place where i made a mistake please fix it and explain how it was done and where i made the mistake .
You are developing a Fraction structure for Teachers Pet Software. The structure contains three public data fields for whole number, numerator, and denominator. Using the same structure, write the functions described below: An enterFractionValue()function that declares a local Fraction object and prompts the user to enter values for the Fraction. Do not allow the user to enter a value of 0 for the denominator of any Fraction; continue to prompt the user for a denominator value until a valid one is entered. The function returns a data-filled Fraction object to the calling function. A reduceFraction()function that accepts a Fraction object and reduces it to proper form, returning the reduced Fraction. For example, a Fraction entering the function as 0 2/6 would be reduced to 0 1/3, and a Fraction entered as 4 18/4 would be reduced to 8 1/2. A displayFraction()function that displays any Fraction object passed to it. This function displays a fraction with a slash between the numerator and denominator. A main()function that declares a Fraction object and continues to get Fraction values from the user until the user enters a Fraction with value 0 (both the whole number and the numerator are 0). For each Fraction entered, display the Fraction, reduce the Fraction, and display the Fraction again.
My solution:
#include
using namespace std;
// Creating a structure fraction
struct fraction {
// Creating data to store wholenumber, numerrator and denominator
int wholenumber;
int numerator;
int denominator;
};
//Renaming fraction
typedef fraction fraction;
fraction enterfractionValue()
{
fraction fraction;
cout << "Enter the whole number fraction : ";
cin >> fraction.wholenumber;
cout << "Enter the numerator number fraction : ";
cin >> fraction.numerator;
//Loop till the denominator is 0
do
{
cout << "Enter the denominator number fraction : ";
cin >> fraction.denominator;
if (fraction.denominator == 0)
{
cout << "denominator is invalid ";
cout << "Enter the denominator number fraction : ";
}
// if denominator is not 0 come out of loop
else
break;
} while (1);
return fraction;
void displayfraction();
{
cout << fraction.wholenumber << "" << fraction.numerator << "/" << fraction.denominator;
}
//Taking a fraction object and reducing the fraction
void reducedfraction();
//Multiply the whole number with denominator and add the numerator to get the reduced number
int reduce = fraction.wholenumber * fraction.denominator + fraction.numerator;
//Divide the reduce by the denominator and store
fraction.wholenumber = reduce / fraction.denominator;
//Divide the reduced number and store the remainder
fraction.numerator = reduce % fraction.denominator;
if ((fraction.numerator % 2 == 0) &&
(fraction.denominator % 2 == 0))
//Divide both the denominator and numerator by 2
{
fraction.denominator = fraction.denominator / 2;
fraction.numerator = fraction.numerator / 2;
}
}
//Initiataing the main function
int mian()
{
//Creating a fraction object fraction1
fraction fraction1;
do
{
fraction1 = enterfractionValue();
if ((fraction1.wholenumber == 0) && (fraction1.numerator == 0))
break;
displayfraction(fraction1);
displayfraction(fraction1);
reducedfraction(fraction1);
};
system("pause");
return 0;
};
Step by Step Solution
There are 3 Steps involved in it
Step: 1
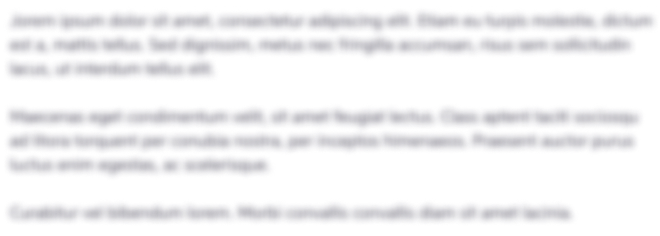
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started