Question
I would like to know if my C# program I have written conforms to the following requirements; Now, you will add error handling into this
I would like to know if my C# program I have written conforms to the following requirements;
"Now, you will add error handling into this project. Recall that you are using the int.TryParse() function to parse out the values entered by the user.
Using the Try/Catch statement, you want to handle any possible exceptions that may occur as a result of performing the operation on the two numbers. Use at least one specific Exception in your catch statement and handle any general exceptions."
\\Program
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Windows.Forms;
namespace Error_Handling { public partial class Form1 : Form { //Set up public variables int num1, num2; bool check1, check2; String text1, text2;
public Form1() { InitializeComponent(); }
//Add Button private void btnAdd_Click(object sender, EventArgs e) { //Set up variables and convert string to integer text1 = txtNum1.Text; check1 = int.TryParse(text1, out num1); text2 = txtNum2.Text; check2 = int.TryParse(text2, out num2);
//Check for valid inputs and dispaly message if error if (check1 == false || check2 == false || (num1 < 0 || num2 < 0)) { MessageBox.Show("Please enter a valid number"); } else //calculate { int res = num1 + num2; txtResult.Text = Convert.ToString(res);
} }
//Subtract Button private void btnSubtract_Click(object sender, EventArgs e) { //Set up variables and convert string to integer text1 = txtNum1.Text; check1 = int.TryParse(text1, out num1); text2 = txtNum2.Text; check2 = int.TryParse(text2, out num2);
//Check for valid inputs and dispaly message if error if (check1 == false || check2 == false || (num1 < 0 || num2 < 0)) { MessageBox.Show("Please enter a valid number"); } else //calculate { int res = num1 - num2; txtResult.Text = Convert.ToString(res);
} } //Multiply Button private void btnMultiply_Click(object sender, EventArgs e) { //Set up variables and convert string to integer text1 = txtNum1.Text; check1 = int.TryParse(text1, out num1); text2 = txtNum2.Text; check2 = int.TryParse(text2, out num2);
//Check for valid inputs and dispaly message if error if (check1 == false || check2 == false || (num1 < 0 || num2 < 0)) { MessageBox.Show("Please enter a valid number"); } else //calculate { int res = num1 * num2; txtResult.Text = Convert.ToString(res);
} }
//Divide Button private void btnDivde_Click(object sender, EventArgs e) { //Set up variables and convert string to integer text1 = txtNum1.Text; check1 = int.TryParse(text1, out num1); text2 = txtNum2.Text; check2 = int.TryParse(text2, out num2);
//Check for valid inputs and dispaly message if error if (check1 == false || check2 == false || (num1 < 0 || num2 < 0)) { MessageBox.Show("Please enter a valid number"); } else //calculate try { int res = num1 / num2; txtResult.Text = Convert.ToString(res);
} //Divide by zero exception catch(Exception divZeroEx) { MessageBox.Show("Cannot divide by zero."); } }
//Clear form private void btnClear_Click(object sender, EventArgs e) { txtNum1.Text = ""; txtNum2.Text = ""; txtResult.Text = ""; }
//Close app private void btnExit_Click(object sender, EventArgs e) { this.Close(); }
} }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
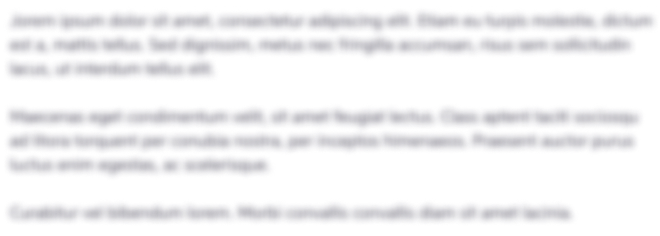
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started