Question
I wrote code in C++ that takes a text file containing information on different football players and their combine results, stores those players as a
I wrote code in C++ that takes a text file containing information on different football players and their combine results, stores those players as a vector of objects of the class, and prints out those objects. Finally, I wrote a function that calculates the average weight of the players.
MAIN.CPP:
#include "Combine.h" #include using namespace std; // main is a global function // main is a global function int main() { // This is a line comment //cout << "Hello, World!" << endl; vector players; getPlayersFromFile("CombinePlayers.txt", players); for (int i = 0; i < players.size(); ++i) { cout << players[i] << endl; //cout << "Hello Jack" << endl; }
avgWeight(players);
return 0; }
COMBINE.H:
#ifndef COMBINE_H_ #define COMBINE_H_ #include #include #include #include #include #include using namespace std; class Combine { private: string name, college, pos; int height, weight; float dash, bench; public: //CONSTRUCTORS Combine() { name = "John Smith"; college = "University"; pos = "player"; height = -1; weight = -1; dash = -1; bench = -1; } Combine(string name, string college, string pos, int height, int weight, float dash, float bench) { this->name = name; this->college = college; this->pos = pos; setHeight(height); setWeight(weight); setDash(dash); setBench(bench); } //GETTERS string getName() const { return name; } string getCollege() const { return college; } string getPos() const { return pos; } int getHeight() const { return height; } int getWeight() const { return weight; } float getDash() const { return dash; } float getBench() const { return bench; } //SETTERS void setName(string name) { this->name = name; } void setCollege(string college) { this->college = college; } void setPos(string pos) { this->pos = pos; } void setHeight(int height) { if (height <= 0) { //Default value this->height = 1; } else { this->height = height; } } void setWeight(int weight) { if (weight <= 0) { //Default value this->weight = 1; } else { this->weight = weight; } } void setDash(float dash) { if (dash <= 0.0) { //Default value this->dash = 1; } else { this->dash = dash; } } void setBench(float bench) { if (bench <= 0.0) { //Default value this->bench = 1; } else { this->bench = bench; } } // Overloaded operators friend ostream& operator<<(ostream &outs, const Combine &com) { outs << left << setw(25) << com.name; outs << left << setw(25) << com.college; outs << left << setw(25) << com.pos; outs << right << setw(5) << com.height; outs << setw(5) << com.weight; outs << setw(5) << com.dash; outs << setw(5) << com.bench; return outs; } }; void getPlayersFromFile(string filename, vector &players) { ifstream inFile; // Use ../ to get out of the cmake-build-debug folder to find the file inFile.open("../" + filename); // inFile.open(filename); string name = "", college = "", pos = "", header = ""; string temp; int height = 0, weight = 0; float dash = 0, bench = 0; char comma = ','; // check that the file is in a good state if (inFile) { // read in the header line getline(inFile, header); // loop through all the data in the file while (inFile && inFile.peek() != EOF) { // read line getline(inFile,temp); // pass it to string strem stringstream ss(temp); // get name getline(ss,name,','); // get college getline(ss,college,','); // get POS getline(ss,pos,','); // get height getline(ss,temp,','); height = atoi(temp.c_str()); // get weight getline(ss,temp,','); weight = atoi(temp.c_str()); if(ss.good()){ // get 40 yard getline(ss,temp,','); dash = atof(temp.c_str()); } else{ dash=1; } if(ss.good()){ // get bench press getline(ss,temp,','); bench = atof(temp.c_str()); } else{ bench=1; } // create a Combine object and put it on the back of the vector players.push_back( Combine(name, college, pos, height, weight, dash, bench)); } } else { cerr << "File not found" << endl; } inFile.close(); }
float avgWeight(vector&players) { float total = 0; getPlayersFromFile("CombinePlayers.txt", players); for (int i = 0; i < players.size(); ++i) { total += players[i].getWeight();//cout << "Hello Jack" << endl; } cout << (total/players.size()); }
#endif /* COMBINE_H_ */
CombinePlayers.txt:
Name, College, POS, Height (in), Weight (lbs), 40 Yard, Bench Press Johnathan Abram, Mississippi State, S, 71, 205, 4.45 Paul Adams, Missouri, OT, 78, 317, 5.18, 16 Nasir Adderley, Delaware, S, 72, 206 Azeez Al-Shaair, Florida Atlantic, LB, 73, 234, 16 Otaro Alaka, Texas A&M, LB, 75, 239, 4.82, 20
OUTPUT:
Johnathan Abram Mississippi State S 71 205 4.45 1 Paul Adams Missouri OT 78 317 5.18 16 Nasir Adderley Delaware S 72 206 1 1 Azeez Al-Shaair Florida Atlantic LB 73 234 16 1 Otaro Alaka Texas A&M LB 75 239 4.82 20
240.2
Now, this only worked because I stored any missing data as a 1 (so, if the player did not have a recorded bench press number, it was stored as 1). Thus, calculations were not impacted. However, I want to be able to print that same data and perform the same calculation, without storing missing data as a 1, but rather leaving it blank. Is this possible?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
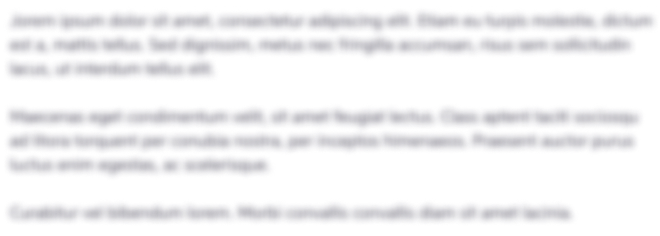
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started