Question
I wrote this program to generate unique random numbers but the output confused me Write a Lottery class that simulates a 6-number lottery (e.g. Lotto).
I wrote this program to generate unique random numbers but the output confused me
Write a Lottery class that simulates a 6-number lottery (e.g. "Lotto"). The class should have an array of six integers named lotteryNumbers, and another array of six integers named userLotteryPicks. The class' constructor should use the Random class to generate a unique random number in the range of 1 to 60 for each of the 6 elements in the lotteryNumbers array. Thus, there should be a loop that keeps generating random numbers until all 6 numbers are unique. The Lottery class should have a method, getUsersPicks(), which prompts the user to enter 6 unique numbers between 1 - 60, which will be stored in the array of integers named usersLotteryPicks. Thus, there should be validation to ensure that each number the user selects is different from all the other numbers that he/she entered previously, and to keep looping until the user enters 6 unique numbers. Finally, the Lottery class should have a method, checkLotteryMatch(), which will compare the 2 arrays - lotteryNumbers & usersLotteryPicks - and return the number of digits that match. The digits do not have to match in the exact order, so a nested loop should be created that goes through each digit in the user's lottery picks array and compares it to each digit in the lotteryNumbers array, counting each of the matches. The checkLotteryMatch() method will return an int containing the number of matches.
Write a Driver class called the LotteryGame, which instantiates the Lottery class. Once a Lottery object has been created, the driver will call the Lottery object's getUsersPicks() method, followed by the checkLotteryMatch() method. Use the results of the checkLotteryMatch() method to determine if the user is a winner by using the following criteria:
For a 3-digit match, display a message to the user that he/she will receive a free Lottery ticket as the prize
For a 4-digit match, display a message to the user that he/she will receive a $2000 prize
For a 5-digit match, display a message to the user that he/she will receive a prize of $50,000.
For a 6-digit match, display a message to the user that he/she will receive a grand prize of $1,000,000.
If there are no matches, display the following message to the user: "Sorry, no matches today. Try again!"
Extra Credit:
Create a method called checkDuplicates which receives any array of ints, and an integer number. The method will return a true or false, depending on whether the integer number passed to the method already exists in the array of ints (also passed to the method).
Call the checkDuplicates method from both the constructor of the Lotto class and the getUserPicks() method also of the Lotto class. This will prevent you from writing duplicate code in both of these places.
This is what I wrote
DOMAIN
package lotterygame; import java.util.Scanner; import java.util.Random; // ///** // * // * @author morty // */ public class Lottery { int[] lotteryNumbers = new int[6]; int[] userLotteryPicks = new int[6];
public static int getRandomNum(int maxNum, int minNum) { return ((int)(Math.random()*(maxNum - minNum))) + minNum; }
public Lottery() { for (int i = 0; i < 6; i++) { int rand = getRandomNum(1, 60); lotteryNumbers[i] = rand; System.out.println(lotteryNumbers[i]); } }
public void getUsersPicks() { Scanner key = new Scanner(System.in); System.out.println("Please enter numbers between 1 & 60 "); for (int j = 0; j < 6; j++) { int userNums = key.nextInt(); while (userNums > 60 || userNums < 1) { userNums = key.nextInt(); } userLotteryPicks[j] = userNums; } }
public int checkDuplicates() { int counter = 0; for (int k = 0; k < lotteryNumbers.length; k++) { if (lotteryNumbers[k] == userLotteryPicks[k]) { counter++; System.out.println(counter); } } return counter; } }
DRIVER
package lotterygame; /** * * @author morty */ public class LotteryGame {
/** * @param args the command line arguments */ public static void main(String[] args) {
Lottery lotto = new Lottery(); lotto.getUsersPicks(); lotto.checkDuplicates(); int counter = lotto.checkDuplicates(); if (counter == 3) { System.out.println("You get a free Lottery ticket as the prize"); } else if (counter == 4) { System.out.println("Bravo, you won $2,000"); } else if (counter == 5) { System.out.println("Fantastic, you won $50,000"); } else if (counter == 5) { System.out.println("Congratulations, you won the grand prize of $1,000,000"); } else if (counter == 0) { System.out.println("Sorry, no matches today. Try again!"); } } }
OUTPUT
These are the lotto numbers 43 41 40 15 15 22 Please enter numbers between 1 & 60 43 50 40 56 15 20 1 2 3 1 2 3 You get a free Lottery ticket as the prize
Step by Step Solution
There are 3 Steps involved in it
Step: 1
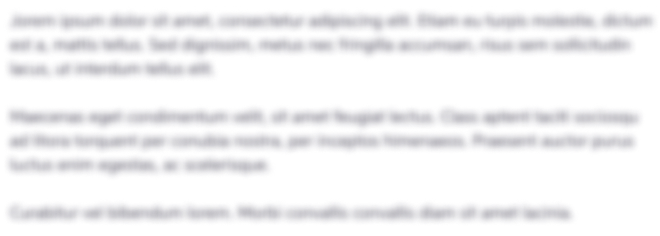
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started