Question
ICS 108 Object-Oriented Programming Lab # 10 Polymorphism Objectives: In this lab, the following topic will be covered: Polymorphism Task Given below the classes Lab10,
ICS 108 Object-Oriented Programming
Lab # 10 Polymorphism
Objectives:
In this lab, the following topic will be covered:
Polymorphism
Task
Given below the classes Lab10, Person, Employee, and Student, complete the following program to produce the output provided.
Note:
Write the missing methods only.
Do not modify the classes Person, Employee, Student.
Do not change the main method.
Put each class in a separate file
Here is a sample run of the program:
List of Employees
Name Phone ID Salary
Saad 0563428255 200003 16000
Salem 0501331845 200001 9000
avg salary of = 12500.0
List of Students
Name Phone ID GPA
Reem 0564448202 20000 3.6
Hasan 0594448202 20002 2.8
avg GPA of students = 3.2
// file Lab10.java
import java.util.ArrayList; public class Lab10 { public static void main(String[] args) { ArrayList
printEmployees(personList); //2. This method finds the average salary of employees
double avgSalary = avgSalary(personList); System.out.println("avg salary of = " + avgSalary); //3. This method prints the students only
printStudents(personList); //4. This method finds the average GPA of students
double avgGpa = avgGpa(personList); System.out.println("avg GPA of students = " + avgGpa); } private static ArrayList
// file Person.java
public class Person { private String name, phone; public Person(String name, String phone) { this.name = name; this.phone = phone; } @Override public String toString() { return name + "\t" + phone; } }
// file Employee.java
public class Employee extends Person{ private int sid; private int salary; public Employee(String name, String phone, int sid, int salary) { super(name, phone); this.sid = sid; this.salary = salary; } public int getSalary() { return salary; } @Override public String toString() { return super.toString() + "\t" + sid + "\t" + salary; } }
// file Student.java
public class Student extends Person{ private int sid; private double gpa; public Student(String name, String phone, int sid, double gpa) { super(name, phone); this.sid = sid; this.gpa = gpa; } public double getGpa() { return gpa; } @Override public String toString() { return super.toString() + "\t" + sid + "\t" + gpa; } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
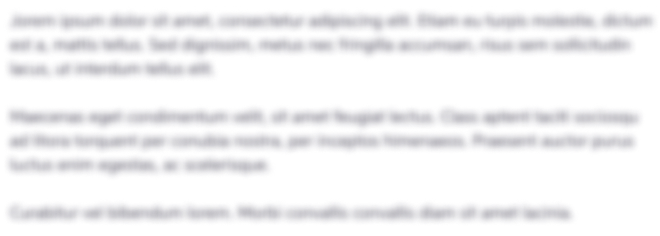
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started