Question
#ifndef TEST_H #define TEST_H /************************************************************/ // System includes #include #include #include #include /************************************************************/ // Local includes using std::copy; using std::copy_backward; using std::distance; using std::fill; using
#ifndef TEST_H
#define TEST_H
/************************************************************/
// System includes
#include
#include
#include
#include
/************************************************************/
// Local includes
using std::copy;
using std::copy_backward;
using std::distance;
using std::fill;
using std::ostream;
using std::ptrdiff_t;
/************************************************************/
template
class Array
{
public:
//*****************************************************
// DO NOT MODIFY THIS SECTION!
// Some standard Container type aliases
using value_type = T;
// Iterators are just pointers to objects of type T
using iterator = value_type*;
using const_iterator = const value_type*;
using reference = value_type&;
using const_reference = const value_type&;
using size_type = size_t;
using difference_type = ptrdiff_t;
//*****************************************************
// Default ctor.
// Initialize an empty Array.
// This method is complete, and does NOT need modification.
// Remember to use a _member initialization list_ for each ctor,
// like I have below for the default ctor.
Array ()
: m_size (0),
m_capacity (0),
m_array (nullptr)
{
}
// Size ctor.
// Initialize an Array of size "pSize", with each element
// set to "value".
explicit Array (size_t pSize, const T& value = T ())
: m_size (pSize),
m_capacity (pSize),
m_array (new T[m_capacity])
{
fill (begin (), end (), value);
}
// Range ctor.
// Initialize an Array from the range [first, last).
// "first" and "last" must be Array iterators or pointers
// into a primitive array.
Array (const_iterator first, const_iterator last)
:m_size(distance(first,last)),m_capacity(m_size),m_array(new T[m_capacity])
{
}
// Copy ctor.
// Initialize this object from "a".
Array (const Array& a)
:m_size(a.m_size),m_capacity(a.m_capacity),m_array(new T[m_capacity])
{
}
// Destructor.
// Release allocated memory.
~Array ()
{
delete[] m_array;
}
// Assignment operator.
// Assign "a" to this object.
// Be careful to check for self-assignment.
Array&
operator= (const Array& a)
{
if (this != &a)
{
delete[] m_array;
m_size = a.m_size;
m_capacity = a.m_capacity;
m_array = new T[m_capacity];
}
return *this;
}
void
push_back (const T& item)
{
insert(end(),item);
}
private:
// Stores the number of elements in the Array.
size_t m_size;
// Stores the capacity of the Array, which must be at least "m_size".
size_t m_capacity;
// Stores a pointer to the first element in the Array.
T* m_array;
};
#endif
Why push_back function does not work?
Step by Step Solution
There are 3 Steps involved in it
Step: 1
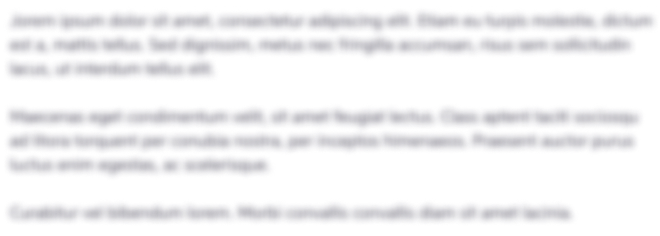
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started