Question
I'm confused on these methods, the bold are the questions: Input Validation Method : int validateInt(String prompt) The method has the following functionality It takes
I'm confused on these methods, the bold are the questions:
Input Validation Method:
int validateInt(String prompt) The method has the following functionality
It takes prompt string as a parameter, It asks user for input using the prompt sting provided,
It validates input, making sure that the value entered is actually an integer. The method uses exceptions to determine if the type of the number entered was correct. The input prompt is repeated in the loop until the user provides a numeric value of the correct type.
It returns the integer value to the calling code. Add Doc comments before the method header.
Numeric Pattern Method just use its stub: public static String numericPattern(int size, boolean direction) throws IllegalArgumentException { return "";} The full description of the method is below.
public static String numericPattern(int size, boolean direction) throws IllegalArgumentException
that returns a string with a pattern of numbers.
Parameter size defines the size of pattern.
Parameter direction defines the direction of the pattern.
Menu-Driven main()
Finally make a menu-driven user-interaction part that displays the following menu
Numeric Pattern Display
1. Print Type I pattern (like 12321)
2. Print Type II pattern (like 32123)
3. Quit Enter your choice (1 - 3):
If the user entered a number that is not a valid choice, he/she must be informed that the choice was invalid, and the menu should appear again.
When user enters any integer, it must be validated with the help of validateInt() method.
When the user choses ether 1 or 2 out of the menu, he/she gets another question - "Please enter the size of the pattern. The size must be a positive integer."
Your program must continue asking for a positive integer until the user provides one and reject all input that is negative or 0. All the user input must go through type validation too. Any input that is not an integer must be rejected as well. Continue using validateInt() method for that.
Use numericPattern () method to generate the pattern string of correct make and size. Print the pattern sting into the screen.
After the pattern is printed the main menu must appear again. The program quits only when the user chose option 3 from the menu.
Point Class
make a definition of a class named Point that might be used to store and manipulate the location of a point on the plane. The point is stored as two coordinates: x and y. Both fields must be of double type & private. You will need to declare and implement the following methods:
- Two constructors:
- no-argument constructor that sets the point coordinates to (1, 1), and
- a constructor that takes x and y coordinate of the point and sets class fields.
- two accessor methods to retrieve the coordinates of the point called getX and getY
- Methods setX and setY that set the private data fields .
- A method to move the point by an amount along the vertical and horizontal directions specified by the first and second arguments: move(double dx, double dy)
- The method to rotate the point by 90 degrees clockwise around the origin called rotate()
RightTriangle Class
make a RightTriangle class that has the following fields:
- legA: a double
- legB: a double
The class should have the following methods:
- No-argument constructor: Sets fields to 1
- Constructor: Accepts legs of the right triangle as arguments. Constructor throws IllegalArgumentException when one or both legs are set to 0 or negative number(s)
- setLegA(), setLegB()- mutator methods. Both methods throw Illegal Argument Exception when leg is set to 0 or negative number.
- getLegA(),getLegB()-accessor methods.
- getHypotenuse()- calculates and returns hypotenuse of the triangle.
- getArea() - calculates and returns area of the right triangle
- getPerimeter()- calculates and returns perimeter of the triangle
- toString()method that represents object as a string in the following format: "legA = 3.0, legB = 4.0"
MyInteger Class
Implement a class named MyInteger. The class contains:
- A data field of type int
- A constructor that takes parameter of type integer andcreates MyInteger object for the specified int value.
- toInteger() method that returns the value of the field.
- The instance methods isEven(), isOdd(), and isPrime() that return true if the value in the object is even, odd, or prime respectively.
- The static methods isEven(int), isOdd(int), and isPrime(int) that return true if the specified value is even, odd, or prime respectively.
- The methods equals(int) and equals(MyInteger) that return true if the value in this object is equal to the specified value.
- A static method parseInt(String) that converts a string into an int value, positive or negative. Method throws IllegalArgumentException when
- A string contains non-digit characters (other than '-' as the very first character of the sting).
- A string has only '-' and no digits.
- A string represents a number that is too large to be stored as integer and produces overflow
- A string represents a number that is too small to be stored as integer and produces underflow
isSorted() method
make a method that takes an array of integers as a parameter and returns true if the array is sorted in ascending order and false if it is not.
The method throws IllegalArgumentException when the array is empty.
isSorted({1}) returns true isSorted({1, 1}) returns true isSorted({1, 3, 2}) returns false isSorted ({3, 18, 20, 21, 21, 56}) returns true
mergeSortedArrays() method
Write a method that takes two SORTED arrays of integers as parameters, merges them into the third array that is also SORTED and returns the new array to the calling code.
The assumption is that both arrays are sorted. Below are the sample method calls.
int[] emptyArray = new int[0]; mergeSortedArrays({1, 4, 9}, {2, 3, 5, 8, 12}) returns {1, 2, 3, 4, 5, 8, 9, 12} mergeSortedArrays(emptyArray, {1}) returns {1} mergeSortedArrays ({1, 2}, {1, 2, 3}) returns {1, 1, 2, 2, 3}
allUniqueElements()
make a method that takes an array of integers and returns true if every integer is appearing in the array only once. It returns false if there are any duplicates in the array. You are not allowed to change the array in any way. If array is empty the method returns false.
Below are some sample method calls.
allUniqueElements({1}) returns true allUniqueElements({1, 2, 3, 9, 1}) returns false allUniqueElements({15, 3, 2}) returns true
twoDConvert()
This method takes a two-dimensional array and converts it into a regular one-dimensional array. The new one-dimensional array is returned from the method.
If the original array is empty, an empty array must be returned.
The assumption is that all rows of the original array are of the same length.
doubleSquares()
In order to implement this method, you will need to add Rectangle class to your project. Please find it inAssignment06.zippackage.
The method takes an array of objects of type Rectangle. Among all the rectangle objects it finds those that are actually squares (length==width), and doubles the values of their length and width fields. The method does not return any result, it just changes the given array.
removeInRange()
This method takes an ArrayList of integers and a range defined by two integer parameters named "from" and "to". The method removes all values from ArrayList that fall in the range[from, to]. It must make no more than one pass through the ArrayList when removing the values.
If the ArrayList is empty, the method does not accept anything.
If "from"value is greater than "to"value, the method throws an IllegalArgumentException
repeat()
This method takes an ArrayList of strings and an integer k. The method modifies the ArrayList by repeating each string it originally contains k times. The method throws IllegalArgumentException when k is negative or a 0.
Dynamic Array of Integers Class
make a class named DynamicArray that will have convenient functionality similar to JavaScript's Array object and Java's ArrayList class. The class allows to store array of integers that can grow and shrink as needed, search for values, remove elements, etc.
You are not allowed to use ArrayList object as well as any methods from java.util.Arrays class.
Please see the list of required features and methods below.
- private int array[] You MUST store the data internally in a regular partially-filled array of integers. Please DO NOT USE ArrayList. The size of the allocated array is its capacity and will be discussed below.
- private int size. This variable stores the number of "occupied" elements in the array. Set to 0 in the constructor.
- Constructor with parameter. The parameter defines the capacity of initial array. Allocates array of given capacity, sets size field to 0. In case the parameter given to constructor is less than 0, IllegalArgumentException is being thrown.
- No-argument constructor. Allocates array of size 3, sets size field to 0.
- Copy constructor. The constructor takes an object of type DynamibcArray as a parameter and copies it into the object it creates. The constructor throws IllegalArgumentException if the object that was passed to copy from is null.
- int getSize() returns the number of "occupied" elements in the array.
- int getCapacity() returns the actual size (length) of the partially-filled array
- int [] getArray() accessor returns the entire partially-filled array. Make sure you DO NOT return the private array field, make a copy of it.
- int [] toArray() accessor returns an "occupied" part of the partially-filled array. Make sure you DO NOT return the private array field. Instead, allocate memory for the new array, copy the "occupied" portion of the field into that new object, and return the new array.
- public void push(int num) adds a new element to the end of the array and increments the size field. If the array is full, you need to increase the capacity of the array:
- make a new array with the size equal to double the capacity of the original one.
- Copy all the elements from the array field to the new array.
- Add the new element to the end of the new array.
- Use new array as an array field.
- Make sure your method works well when new elements are added to an empty DynamicArray.
- public int pop() throws RuntimeException removes the last element of the array and returns it. Decrements the size field. If the array is empty a RuntimeException with the message "Array is empty" must be thrown. At this point check the capacity of the array. If the capacity is 4 times larger than the number of occupied elements (size), it is time to shrink the array:
- make a new array with the size equal to half of the capacity of the original one.
- Copy all the elements from the array field to the new array.
- Use new array as an array field.
- int get(int index) throws IndexOutOfBoundsException returns element of the array with the requested index. If the index provided is too large or negative, the IndexOutOfBoundsException is thrown with the message "Illegal index".
- int indexOf(int key) returns the index of the first occurrence of the given number. Returns -1 when the number is not found.
- void add(int index, int num) throws IndexOutOfBoundsException adds a new element (passed as parameter num) to the location of the array specified by index parameter. If the index is larger than size of the array or less than 0, IndexOutOfBoundsException is thrown. When adding the element into the middle of the array, you'll have to shift all the elements to the right to make room for the new one. If the array is full and there is no room for a new element, the array must be doubled in size. Please follow the steps listed in the push() method description to double the capacity of the array.
- int remove ( int index) throws IndexOutOfBoundsException removes the element at the specified position in this array. When the element is removed from the middle of the array, all the elements must be shifted to close the gap created by removed element. If the index value passed into the method is more or equal to the size or less than 0 the IndexOutOfBoundsException must be thrown. At this point check the capacity of the array. If the capacity is 4 times larger than the number of occupied elements (size), it is time to shrink the array.
- boolean isEmpty() returns true if the size of the array is 0.
- int findMin()throws RuntimeException returns the smallest element in the array. If the array is empty throws RuntimeException
- int findMax()throws RuntimeException returns the largest element in the array. If the array is empty throws RuntimeException
- String toString() - returns an array as a string of comma-separated values. Sample: [1, 2, 3, 4]
- boolean equals(DynamicArray obj) compares two objects (this one and the one passed as parameter) element-by-element and determines if they are exactly the same. The capacity of the two objects is not being compared and can be different.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
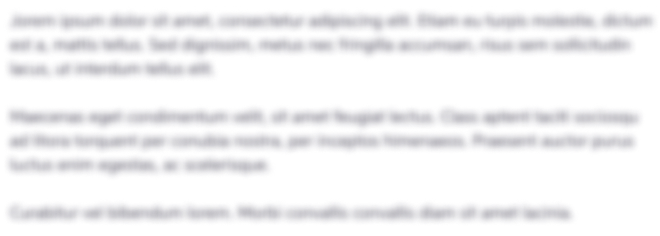
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started