Question
I'm currently writing a program based on stub poker and trying to deal cards to the players, show their hands, and determine the winner, and
I'm currently writing a program based on stub poker and trying to deal cards to the players, show their hands, and determine the winner, and lastly ask them to if they want to play another hand. I'm probably going to have to write a method to compare hands but i really need to just deal the cards and store in their hands Any help or suggestions? Here are my current classes.
public class PlayingCard { private final Suit suit; private final Rank rank; public PlayingCard(Rank newRank, Suit newSuit) { this.rank = newRank; this.suit = newSuit; }// Ending bracket of constructor public Rank getRank() { return this.rank; }// Ending bracket of method getRank public Suit getSuit() { return this.suit; }// Ending bracket of method getSuit public String toString() { return this.rank.getRank() + " of " + this.suit.getSuit(); }// Ending bracket of method toString public String toStringSymbol() { return this.rank.getSymbol() + "" + this.suit.getSymbol(); }// Ending bracket of method toString public int getRankValue() { return this.rank.ordinal() + 2; }// Ending bracket of method getRankVaule //So deuce will return a rank value of 2 seeing how deuce is in the position of 0. public boolean isSameSuit(PlayingCard otherCard) { return(this.getSuit() == otherCard.getSuit()); }// Ending bracket of method isSameSuit public boolean isSameRank(PlayingCard otherCard) { return(this.getRank() == otherCard.getRank()); }// Ending bracket of method isSameRank
}// Ending bracket of class PlayingCard
--------------------------------------------------------------
public enum Rank { DEUCE("Deuce", '2'), TREY("Trey", '3'), FOUR("Four", '4'), FIVE("Five", '5'), SIX("Six", '6'), SEVEN("Seven", '7'), EIGHT("Eight", '8'), NINE("Nine", '9'), TEN("Ten", 'T'), JACK("Jack", 'J'), QUEEN("Queen", 'Q'), KING("King", 'K'), ACE("Ace", 'A'); private String rank; private char symbol; private Rank(String newRank, char newSymbol) { this.rank = newRank; this.symbol = newSymbol; }// Enidng bracket of constructor public String getRank() { return this.rank; }// Ending bracket of method getRank; public char getSymbol() { return this.symbol; }
}// Ending bracket of class Rank
-------------------------------------------
public enum Suit { HEARTS("Hearts", '\u2665'), DIAMONDS("Diamonds", '\u2666'), CLUBS("Clubs", '\u2663'), SPADES("Spades", '\u2660'); private String suit; private char symbol; private Suit(String newSuit, char newSymbol) { this.suit = newSuit; this.symbol = newSymbol; }// Ending bracket of constructor public String getSuit() { return this.suit; }// Ending bracket of method public char getSymbol() { return this.symbol; }// Ending bracket of getSymbol
}// Ending bracket of enum Suit ----------------------------------------------------
import java.util.Random;
public class Deck { private PlayingCard[] cards; private static final int DECK_SIZE = 52; private Random randomizer; public Deck() { int arrayIndex = 0; this.cards = new PlayingCard[DECK_SIZE]; this.randomizer = new Random(); for(Suit suit : Suit.values()) { for(Rank rank : Rank.values()) { cards[arrayIndex++] = new PlayingCard(rank, suit); }//Ending bracket of inner for each loop }// Ending bracket of outer for each loop }// Ending bracket of Deck constructor public PlayingCard deal() { PlayingCard rv = null; int index = 0; do { index = randomizer.nextInt(DECK_SIZE); rv = cards[index]; } while (rv == null); cards[index] = null; return rv; }// Ending bracket of PlayingCard deal method public void displayDeck() { for(PlayingCard card : cards) { System.out.println(card.toString()); }// Ending bracket of for each loop }// Ending bracket of displayDeck method public void displayDeckSymbol() { for(PlayingCard card : cards) { System.out.println(card.toStringSymbol()); }// Ending bracket of for each loop }// Ending bracket of displayDeck method
}// Ending bracket of class Deck
--------------------------------------------
public class Hand {
private PlayingCard[] cards; private int currentNumberOfCards; private static final String COMMA_SPACE = ", "; public Hand(int numberOfCardsToHold) { this.cards = new PlayingCard[numberOfCardsToHold]; this.currentNumberOfCards = 0; }// Ending bracket of constructor public void sort() { boolean isNotSorted = true;//sentinal value - PlayingCard tempCard = null;// use null if you do not have an object while(isNotSorted) { isNotSorted = false; for(int i = 0; i < this.cards.length - 1; ++i) { if(this.cards[i].getRankValue() < this.cards[i + 1].getRankValue()) { tempCard = this.cards[i]; this.cards[i] = this.cards[i + 1]; this.cards[i + 1] = tempCard; isNotSorted = true; }// Ending bracket of if }// Ending bracket of for }// Ending bracket of while }// Ending bracket of method sort public void addCard(PlayingCard newCard) { this.cards[this.currentNumberOfCards] = newCard;// the assigning a card the the array cards this.currentNumberOfCards++; }// Ending bracket of addCard public String toString() { StringBuffer sb = new StringBuffer();// to add string without extra memory for (PlayingCard card : this.cards) { sb.append(card.toString());// append means to add to the end sb.append(COMMA_SPACE); }// Ending bracket of for each loop - Cards return sb.toString();// }// Ending bracket of method toString public String toStringSymbol() { StringBuffer sb = new StringBuffer();// to add string without extra memory for (PlayingCard card : this.cards) { sb.append(card.toString()); sb.append(COMMA_SPACE); }// Ending bracket of for loop return sb.toString();// }// Ending bracket of method toString
}// Ending bracket of class Hand
------------------------------------------------------
public class PokerPlayer { private String name; private Hand hand; public PokerPlayer(String newName, int numberOfCards) { this.setName(newName); this.setHand(new Hand(numberOfCards)); }// Ending bracket of constructor public void addCard(PlayingCard newCard) { this.hand.addCard(newCard);; }// Ending bracket of method addCard public String getName() { return this.name; }// Ending bracket of method getNasme public void setName(String newName) { this.name = newName; }// Ending bracket of method setName public Hand getHand() {// We are breaking encapsulation doing this. return this.hand; }// Ending bracket of method getHand public void setHand(Hand newHand) { this.hand = newHand; }// Ending bracket of setHand
Step by Step Solution
There are 3 Steps involved in it
Step: 1
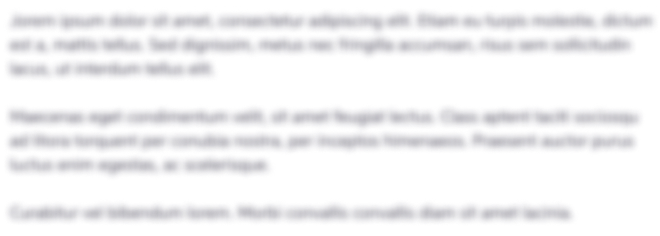
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started