Question
Im going to give you some simple code for a linked list, and youre going to add methods that do things. In the code I
Im going to give you some simple code for a linked list, and youre going to add methods that do things.
In the code I give you have a method called AddLast. Your first task is to make a different method called AddFirst
AddLast is inefficient because you have to traverse the list every time you want to add something to it. In a singly Linked List with only a head pointer its more efficient to add to the head of the list.
public void AddLast(Object data){
// make a new node using data
//point the new node.next to head (thats just node.next = head;)
//set head = new node
// obviously you cant call your node to be inserted new node
}
public void DeleteLast()
{
//this should delete the last element in the list
//so traverse the list until the last element and then delete it
}
Lastly but not trivially, I want you to uncomment out the
// private Node tail;
And then change AddFirst, AddLast and Delete last to account for it
HERE IS THE CODE:
namespace lab3 { public class Node { public Node next; // public Node previous; public Object data; } public class LinkedList { private Node head; // private Node tail; public void printAllNodes() { Node current = head; while(current != null) { Console.WriteLine(current.data); current = current.next; } } public void AddFirst(Object data) {
} public void AddLast(Object data) { if (head == null) { head = new Node(); head.data = data; head.next = null; } else { Node toAdd = new Node(); toAdd.data = data;
Node current = head; while(current.next != null) { current = current.next; } current.next = toAdd; } } public void DeleteLast() {
} } class Program { static void Main(string[] args) { Console.WriteLine("Add Last: "); LinkedList mylist2 = new LinkedList();
mylist2.AddLast("Richard"); mylist2.AddLast("Brian"); mylist2.AddLast("Bonnie"); mylist2.AddLast("Jamie"); mylist2.AddLast("Wenying"); mylist2.AddLast("Omar"); mylist2.printAllNodes(); Console.WriteLine();
// PART OF THIS LAB IS MAKING THIS CODE WORK, YOU NEED TO IMPLEMENT AddFirst Console.WriteLine("Add First"); LinkedList myList1 = new LinkedList(); myList1.AddFirst("Richard"); myList1.AddFirst("Brian"); myList1.AddFirst("Bonnie"); myList1.AddFirst("Sabine"); myList1.AddFirst("Wenying"); myList1.AddFirst("Omar"); myList1.printAllNodes(); Console.WriteLine();
Console.ReadLine(); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
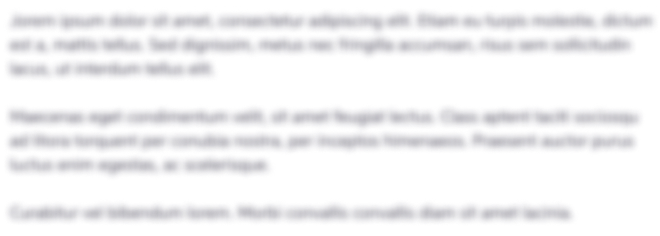
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started