Question
I'm having trouble putting my C programming code into the correct function format specified by the question. The questions asks use the code skeleton posted
I'm having trouble putting my C programming code into the correct function format specified by the question. The questions asks use the code skeleton posted below, but I do not know how to. I have the code and it does exactly what its intended to do but it should work through a function and follow the format, the goal of the code is to make an n x n 2d array/matrix, allow the user to set the array/matrix size and input its values, print the array/matrix in tabular format, check if its a magic square matrix/array, check if all the values in the array are distinct, sort and print it using row-wise approach and transpose it and print the transposed array. Please help so it works through that format:
Here is what the code skeleton looks like, it should use these functions:
#include #define MAX 100
void setMatrixData(int numOfRows, int mat[][numOfRows]){ // provide the implementation }
void printMatrixData(int numOfRows, int mat[][numOfRows]){ // provide the implementation }
int isMagicSquare(int numOfRows, int mat[][numOfRows]){ // provide the implementation return 1; // if the matrix is a magic square }
int isDistinctSquare(int numOfRows, int mat[][numOfRows]){ // provide the implementation return 1; // if the matrix has no duplicates all values are unique }
void SortRowWise(int numOfRows, int mat[][numOfRows]){ // provide the implementation } void GetTranspose(int numOfRows, int mat[][numOfRows], int tran[][numOfRows]){ // provide the implementation }
int main() { int matrix[MAX][MAX]; int transpose[MAX][MAX];
int numOfRows;
printf("Done..."); }
Here is my code, which works perfectly as intended but does not follow the above function format:
#include #define MAX 100
int main() { int mat[MAX][MAX]; int i, j, m, n; int rowsum, columnsum, diagonalsum; int k; int magic = 0; int transpose[MAX][MAX]; printf("Enter the # of rows and columns of the square matrix (must be n x n): "); scanf("%d %d", &m, &n); if (m == n) { printf("Enter the elements of the matrix:"); for (i = 0; i < m; i++) { for (j = 0; j < n; j++) { scanf("%d", & mat[i][j]); } } printf("The matrix is: "); for (i = 0; i < m; i++) { for (j = 0; j < n; j++) { printf("%d ", mat[i][j]); } printf(" "); } for (i = 0; i < m; i++) for (j = 0; j < n; j++) transpose[j][i] = mat[i][j]; printf("Transpose of the matrix: "); for (i = 0; i < n; i++) { for (j = 0; j < m; j++) printf("%d ", transpose[i][j]); printf(" "); } diagonalsum = 0; for (i = 0; i < m; i++) { for (j = 0; j < n; j++) { if (i == j) { diagonalsum = diagonalsum + mat[i][j]; } } } for (i = 0; i < m; i++) { rowsum = 0; for (j = 0; j < n; j++) { rowsum = rowsum + mat[i][j]; } if (rowsum != diagonalsum) { printf("The matrix is not magic square "); } } for (i = 0; i < m; i++) { columnsum = 0; for (j = 0; j < n; j++) { columnsum = columnsum + mat[j][i]; } if (columnsum != diagonalsum) { printf("The matrix is not magic square "); } } printf("The matrix is a magic square "); } else { printf("Please enter a square matrix, where m = n"); } int all_distinct = 1; int count, l; for (i = 0; i < m; i++) { for (j = 0; j < n; j++) { count = 0; for (k = 0; k < m; k++) { for (l = 0; l < n; l++) { if (mat[k][l] == mat[i][j]) { count++; } } } if (count != 1) { all_distinct = 0; } } } if (all_distinct) { printf("The square matrix is distinct "); } else { printf("The square matrix is not distinct "); } for (int i = 0; i < m; i++) { for (int j = 0; j < n; j++) { for (int k = j + 1; k < n; k++) { if (mat[i][j] > mat[i][k]) { int t = mat[i][j]; mat[i][j] = mat[i][k]; mat[i][k] = t; } } } } printf("The matrix after sorting row wise is: "); for (i = 0; i < m; i++) { for (j = 0; j < n; j++) { printf("%d ", mat[i][j]); } printf(" "); } return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
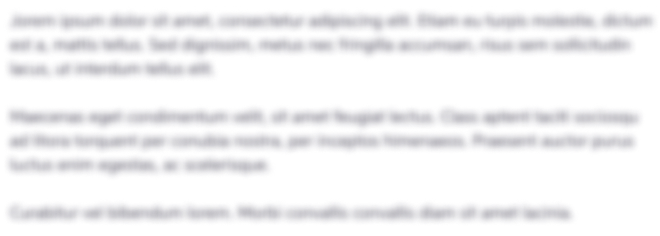
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started