Question
I'm really confused about this and don't understand what I am supposed to do or how to do it. I need some help starting this.
I'm really confused about this and don't understand what I am supposed to do or how to do it. I need some help starting this.
.
.
File RouteCipher.java contains the shell for the RouteCipher class. The RouteCipher class encrypts (puts into a coded form) a message by changing the order of the characters in the message. The route cipher fills a two-dimensional array with single-character substrings of the original message in row-major order, encrypting the message by retrieving the single-character substrings in column-major order. For example, the word Surprise can be encrypted using a 2-row, 4-column array as follows.
(original message) "Surprise"
Contents of Array S u r p r I s e
(encrypted message) "Sruirspe"
[Partial] Software Design: 1. Write the method fillBlock that fills the two-dimensional array letterBlock with one-character Strings taken from the String passed as parameter str. The array must be filled in row-major order the first row is filled from left to right, then the second row is filled from left to right, and so on, until all rows are filled. If the length of the parameter str is smaller than the number of elements of the array, the String A is placed in each of the unfilled cells. If the length of str is larger than the number of elements in the array, the trailing characters are ignored (by this method). 2. Write the method encryptBlock that encrypts the contents of letterBlock. The method builds an encrypted version of the contents of letterBlock by concatenating the single-character Strings in column major order. 3. Write the method encryptMessage that encrypts its String parameter message. Repeatedly call fillBlock with consecutive, nonoverlapping substrings of message until the entire message has been encrypted. After each call to fillBlock, call encryptBlock and continue to concatenate the returned Strings When all of message has been processed, return the concatenated String. Note that if message is the empty String, encryptMessage returns an empty String. Use EncryptionDriver.java to test your code. [Sample] Test Data: Using 2 rows and 4 columns: "Surprise" encrypts as "Sruirspe" "My cat" encrypts as "Mayt AcA" "It was the best of times" encrypts as "Iats wthees tb oifm ets" where the first space is actually a pair of spaces and the others are just single spaces Description points Comments (name and date) on all submitted files 1 variable names: descriptive. Not single letters. 2 comments: methods are commented internally 2 fillBlock 3 encryptBlock 3 encryptMessage 6
.
.
.
// 2D array processing to encrypt a string
public class RouteCipher { //instance data: //A 2D array of single-character Strings private String[][] letterBlock;
// The number of rows of letterBlock, set by constructor*/ private int numRows;
// The number of columns of letterBlock, set by constructor*/ private int numCols;
/****************************** / 2-arg construtor / @param int r: # of rows in array / @param int c: # of columns in array /******************************/ public RouteCipher(int r, int c) { numRows = r; numCols = c; letterBlock = new String[numRows][numCols]; }
/**************************************** / fillBlock method / Places a String into letterBlock in row-major order. / @param String str: the String to be processed / Postcondition: / if str.length() < numRows * numCols, "A" is placed in each unfilled cell / if str.length() > numsRows * numCols, trailing chracters are ignored] *****************************************************/ private void fillBlock(String str) { }
/****************************************** / encryptBlock method / Creates an encrypted String by extracting (single character) / Strings from letterBlock in column-major order. / Precondition: letterBlock has been filled / @return String the encrypted String from letterBlock ***********************************************/ private String encryptBlock() { } /******************************************* / encryptMessage method / Encrypts a message / @param String message: the String to be encrypted / @return String the encrypted message; / if the message is the empty String, returns the empty String / otherwise encrypts the message by calling the private / helper methods fillBlock and encryptBlock **********************************************/ public String encryptMessage(String message) { }
}
.
.
.
// Driver for the RouteCipher program
import java.util.Scanner; public class EncryptionDriver { public static void main(String[] args) { Scanner scanNums = new Scanner(System.in); Scanner scan = new Scanner(System.in); String ans = ""; do{ System.out.println("Enter desired number of rows:"); int r = scanNums.nextInt(); System.out.println("Enter desired number of columns:"); int c = scanNums.nextInt(); RouteCipher rc = new RouteCipher(r,c); System.out.println("Enter message to be encrypted: "); String enc = scan.nextLine(); System.out.println(rc.encryptMessage(enc)); System.out.println("Would you like to encrypt again (yes or no)?"); ans = scan.nextLine(); } while(ans.equals("yes")); }
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
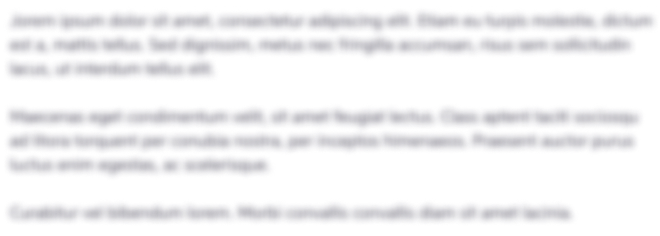
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started