Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Implement a class CountingArrayList in Java that extends ArrayList and that counts the number of calls to get and set. Complete the following file: import
Implement a class CountingArrayList in Java that extends ArrayList
Complete the following file:
import java.util.ArrayList;
public class CountingArrayList extends ArrayList
Here are the test files
Use the following files:
CountingArrayListTester.java
import java.util.ArrayList; import java.util.Collections; public class CountingArrayListTester { public static String smallest(ArrayListvalues) { String smallestSoFar = values.get(0); for (int i = 1; i < values.size(); i++) { String value = values.get(i); if (value.compareTo(smallestSoFar) < 0) { smallestSoFar = value; } } return smallestSoFar; } public static void main(String[] args) { CountingArrayList words = new CountingArrayList(); words.add("Mary"); words.add("had"); words.add("a"); words.add("little"); words.add("lamb"); words.add("its"); words.add("fleece"); words.add("was"); words.add("white"); words.add("as"); words.add("snow"); String smallest = smallest(words); System.out.println(words.count("get")); System.out.println("Expected: 11"); System.out.println(words.count("set")); System.out.println("Expected: 0"); } }
CountingArrayListTester2.java
import java.util.ArrayList; public class CountingArrayListTester2 { public static void reverse(ArrayListwords) { for (int i = 0; i < words.size() / 2; i++) { String oldValue = words.set(words.size() - i - 1, words.get(i)); words.set(i, oldValue); } } public static void main(String[] args) { CountingArrayList words = new CountingArrayList(); words.add("Mary"); words.add("had"); words.add("a"); words.add("little"); words.add("lamb"); words.add("its"); words.add("fleece"); words.add("was"); words.add("white"); words.add("as"); words.add("snow"); reverse(words); System.out.println(words.count("get")); System.out.println("Expected: 5"); System.out.println(words.count("set")); System.out.println("Expected: 10"); System.out.println(words); System.out.println("Expected: [snow, as, white, was, fleece, its, lamb, little, a, had, Mary]"); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
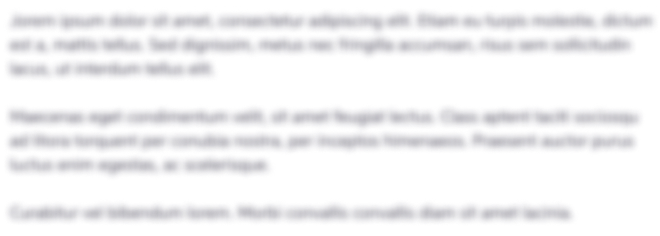
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started