Question
Implement a function in ARM assembler (factorial.s) to calculate the factorial of any number up to 100. Provide a main function driver written in C
Implement a function in ARM assembler (factorial.s) to calculate the factorial of any number up to 100. Provide a main function driver written in C to call your assembler function.
Example function call:
printf("The factorial = ", factorial(100));
Since the factorial of a number larger than 15 is too large to be stored as an int in C, you will need to implement a linked list in assembler. Use the example C code we wrote in class on Monday to calculate a large factorial as a guide if you need to.
Here is the C code as well.
#include
#include
struct Node* factorial(int n);
struct Node
{
int data;
struct Node *next;
};
int main(int argc, char const *argv[])
{
struct Node product;
product = factorial(30);
printf("factorial is: %d",product->data);
free();
return 0;
}
struct Node* factorial(int n){
struct Node *lead, *lag, product;
product ->data = n;
lag->data = n;
lead = lag;
while(n != 1){
lead->next = malloc(sizeof(struct Node));
product->data *= lead->data;
lead = lead->next;
lead->data = --n;
}
lead->next = NULL;
return product;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
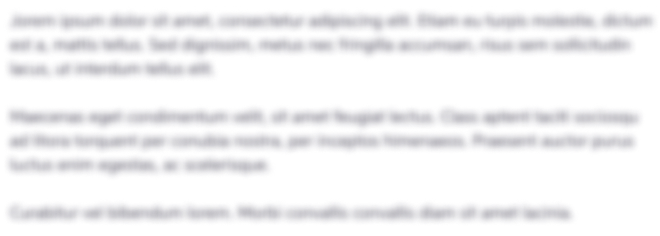
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started