Question
Implement all the methods that have throw new RuntimeException(Not Implemented); package week07; import java.text.DecimalFormat; import java.util.GregorianCalendar; /** * An instance of this class represents a
Implement all the methods that have throw new RuntimeException("Not Implemented"); package week07; import java.text.DecimalFormat; import java.util.GregorianCalendar; /** * An instance of this class represents a single library book. To use this class * you must * * @author Scott LaChance */ public class Book { /** * Constructor. * * @param dueDate * the due date of this book * */ public Book(GregorianCalendar dueDate) { this(dueDate, CHARGE_PER_DAY); } /** * Constructor. * * @param dueDate * the due date of this book * @param chargePerDay * charge per overdue day * */ public Book(GregorianCalendar dueDate, double chargePerDay) { this(dueDate, chargePerDay, MAX_CHARGE); } /** * Constructor. * * @param dueDate * the due date of this book * @param chargePerDay * charge per overdue day * @param maximumCharge * the maximum possible charge * @param title * the title of this book * */ public Book(GregorianCalendar dueDate, double chargePerDay, double maximumCharge) { this(dueDate, DEFAULT_TITLE, chargePerDay, maximumCharge ); } /** * Constructor. * * @param dueDate * the due date of this book * @param chargePerDay * charge per overdue day * @param maximumCharge * the maximum possible charge * @param title * the title of this book * */ public Book(GregorianCalendar dueDate, String title, double chargePerDay, double maximumCharge) { setDueDate(dueDate); setChargePerDay(chargePerDay); setMaximumCharge(maximumCharge); setTitle(title); } public Book clone() { return new Book(getDueDate(), getTitle(), getChargePerDay(), getMaxCharge()); } /** * Computes the overdue charge for the specified return date * Algorithm: * Get the due date * Get the number of days difference from the dueDate and the returnDate (current date) * If the days are > 0, meaning you have exceeded the return date, then * calculate the charge * Charges per day * number days late * Check to see if the calculated charges exceed the max charge * Set to max charge if the calculation exceeds it * return the charge * * @param returnDate * the date the book is/to be returned * * @return the amount of overdue charge */ public double computeCharge(GregorianCalendar returnDate) { throw new RuntimeException("Not Implemented"); } /** * Rounds a decimal using the DecimalFormat class * Use the DecimalFormat class to format the double * to #.## * @param d Decimal to round * @return Formatted double value */ private static double roundDecimal(double d) { throw new RuntimeException("Not Implemented"); } /** * Returns the per day charge of this book * * @return the per day charge of this book */ public double getChargePerDay() { throw new RuntimeException("Not Implemented"); } /** * Returns the due date of this book * * @return the due date of this book */ public GregorianCalendar getDueDate() { throw new RuntimeException("Not Implemented"); } /** * Returns the maximum possible charge of this book * * @return the maximum possible charge of this book */ public double getMaxCharge() { throw new RuntimeException("Not Implemented"); } /** * Returns the title of this book * * @return the title of this book */ public String getTitle() { throw new RuntimeException("Not Implemented"); } /** * Sets the per day charge of this book * * @param charge * the per day charge of this book */ public void setChargePerDay(double charge) { throw new RuntimeException("Not Implemented"); } /** * Sets the due date of this book * * @param date * the due date of this book */ public void setDueDate(GregorianCalendar date) { throw new RuntimeException("Not Implemented"); } /** * Sets the maximum possible charge for this book * * @param charge * the maximum possible charge for this book */ public void setMaximumCharge(double charge) { throw new RuntimeException("Not Implemented"); } /** * Sets the title of this book * * @param title * the title of this book */ public void setTitle(String title) { throw new RuntimeException("Not Implemented"); } /** * Returns the string representation of this book in the format * * * @return string representation of this book */ public String toString() { return String.format("%-30s $%5.2f $%7.2f %4$tm/%4$td/%4$ty", getTitle(), getChargePerDay(), getMaxCharge(), dueDate.getTime()); // or you can do the following to get a similar result /* * String tab = "\t"; * * SimpleDateFormat sdf = new SimpleDateFormat("MM/dd/yy"); * DecimalFormat df = new DecimalFormat("0.00"); * * return getTitle() + tab + "$ " + df.format(getChargePerDay()) + tab + * "$ " + df.format(getMaxCharge()) + tab + * sdf.format(dueDate.getTime()); */ } /** Default charge per day */ private static final double CHARGE_PER_DAY = 0.50; /** Default maximum charge */ private static final double MAX_CHARGE = 50.00; /** Default title of the book */ private static final String DEFAULT_TITLE = "Title unknown"; /** * Due date of the book * * @uml.property name="dueDate" */ private GregorianCalendar dueDate; /** * Title of the book (optional) * * @uml.property name="title" */ private String title; /** * Charge due per overdue day * * @uml.property name="chargePerDay" */ private double chargePerDay; /** * Maximum charge for the overdue * * @uml.property name="maximumCharge" */ private double maximumCharge; } Annotations
Step by Step Solution
There are 3 Steps involved in it
Step: 1
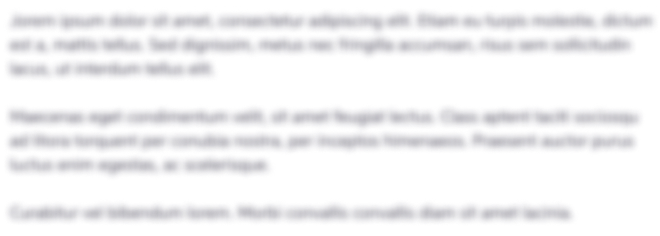
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started