Answered step by step
Verified Expert Solution
Question
1 Approved Answer
'implement the shiftRight method in the ArrayIndexedList class. List.java import java.util.Iterator; public interface List extends Iterable { /** Returns the first element in this list.
'implement the shiftRight method in the ArrayIndexedList class.
List.java
import java.util.Iterator; public interface List extends Iterable { /** Returns the first element in this list. */ T first(); /** Returns the last element in this list. */ T last(); /** Removes the first element in this list. */ T removeFirst(); /** Removes the last element in this list. */ T removeLast(); /** Removes the specified element from this list. */ T remove(T element); /** Returns true if this list has no elements, false otherwise. */ boolean isEmpty(); /** Returns the number of elements in this list. */ int size(); /** Returns an iterator over the elements in this list. */ Iterator iterator(); /** Returns a string representation of this list. */ String toString();
IndexedList.java public interface IndexedList extends List { /** Adds the given element at the specified index in this list. */ boolean add(T element, int index); /** Adds the given element at the end of this list. */ boolean add(T element); /** Replaces the current element at the specified index with the given elemeent. */ boolean set(T element, int index); /** Returns the element at the given index in this list. */ T get(int index); /** Returns the index of the specified element in this list. */ int indexOf(T element); /** Removes the element at the specified index in this list. */ T remove(int index); }
ArrayIndexedList.java
import java.util.Iterator; public class ArrayIndexedList implements IndexedList { /** stores elements of list. */ T[] elements; /** index of next insertion point; number of elements in list. */ int rear; /** Constructs an instance of this list. */ @SuppressWarnings("unchecked") public ArrayIndexedList(int capacity) { elements = (T[]) new Object[capacity]; rear = 0; } /** Returns the first element in this list. */ public T first() { return null; } /** Returns the last element in this list. */ public T last() { return null; } /** Removes the first element in this list. */ public T removeFirst() { return null; } /** Removes the last element in this list. */ public T removeLast() { return null; } /** Removes the specified element from this list. */ public T remove(T element) { return null; } /** Returns true if this list has no elements, false otherwise. */ public boolean isEmpty() { return rear == 0; } /** Returns the number of elements in this list. */ public int size() { return rear; } /** Returns an iterator over the elements in this list. */ public Iterator iterator() { return null; } /** Returns a string representation of this list. */ public String toString() { StringBuilder result = new StringBuilder(); result.append("["); for (int i = 0; i < rear; i++) { result.append(elements[i]); result.append(", "); } result.delete(result.length() - 2, result.length()); result.append("]"); return result.toString(); } /** Adds the given element at the specified index in this list. */ public boolean add(T element, int index) { if (index == rear) { return add(element); } if (!validIndex(index)) { return false; } if (isFull()) { resize(elements.length * 2); } shiftRight(index); elements[index] = element; rear++; return true; } /** Adds the given element at the end of this list. */ public boolean add(T element) { if (isFull()) { resize(elements.length * 2); } elements[rear] = element; rear++; return true; } /** Replaces the current element at the specified index with the given elemeent. */ public boolean set(T element, int index) { return false; } /** Returns the element at the given index in this list. */ public T get(int index) { return null; } /** Returns the index of the specified element in this list. */ public int indexOf(T element) { return -1; } /** Removes the element at the specified index in this list. */ public T remove(int index) { return null; } /** Returns true if the array is full, false otherwise. */ private boolean isFull() { return rear == elements.length; } /** Resizes the array to the specified size, copying corresonding elements. */ @SuppressWarnings("unchecked") void resize(int capacity) { assert (capacity >= rear); T[] newArray = (T[]) new Object[capacity]; for (int i = 0; i < rear; i++) { newArray[i] = elements[i]; } elements = newArray; } /** Moves elements from index..rear-1 one index to the right. */ private void shiftRight(int index) { assert !isFull(); // you must fill this in } /** Returns true if specified index is in the legal range, false otherwise. */ private boolean validIndex(int index) { return (index >= 0) && (index < rear); } }
ArrayIndexedListClient.java public class ArrayIndexedListClient { /** Drives execution. */ public static void main(String[] args) { ArrayIndexedList list = new ArrayIndexedList<>(5); list.add("A"); list.add("B"); list.add("C"); list.add("D"); list = new ArrayIndexedList<>(5); list.add("A", 0); list.add("B", 1); list.add("C", 2); list.add("D", 3); list.add("E", 5); list.add("E", 1); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
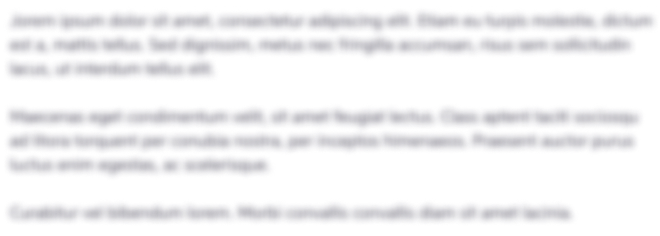
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started