Question
import java.awt.*; import java.awt.event.*; import java.util.ArrayList; import javax.swing.*; /** * * ========================================================================== * CODING INSTRUCTIONS! * ========================================================================== * * * * Task 0: * compile
import java.awt.*;
import java.awt.event.*;
import java.util.ArrayList;
import javax.swing.*;
/**
*
* ==========================================================================
* CODING INSTRUCTIONS!
* ==========================================================================
*
*
*
* Task 0:
* compile and run SnakeGame as a Java Application.
*
* Task 1: (in class Snake)
* create a class called Snake in a separate Java source file.
* import java.awt.*;
*
* required fields (or instance variables):
* int size, direction, bodyAdd;
* Rectangle head;
* ArrayListbody;
* ArrayListdirectionQ;
* (note: look up Rectangle in the Java Documentation for more information)
* write a default constructor for the Snake Object
* size is 20 (the number of pixels for each Snake piece)
* direction is 8 (8 is up, 2 down, 4 left, and 6 right.. look at numpad)
* bodyAdd is 4 (4 body pieces will be initially added to the Snake
* head is a Rectangle that is 20x20 and
* is located in the approximate center of the window (i.e. coordinates (300,300))
* remember to initialize body and directionQ to new ArrayLists
* write method: public void draw(Graphics2D g)
* that will render the head of Snake ..use g.draw(head)
* and the body of Snake (use a for-loop)
*
* Task 1a: (in class SnakeGame)
* uncomment the snake instance variable
* in method initRound()
* initialize variable snake
* in method draw()
* render snake by invoking its draw method
* YOU SHOULD SEE snake APPEAR IN THE CENTER OF THE WINDOW!
*
*
* Task 3: (in class Snake)
* write method: public int getDirection() that returns the direction
* write method: public Rectangle getHead() that returns the head
* write method: public void grow() that adds 5 to variable bodyAdd
* write method: public void setDirection(int d)
* (note: d will be either 2,4,6, or 8)
* add d to the end of directionQ
* (note: it is ok to add an int value to an ArrayList)
* write method: public void move()
* if the directionQ is not empty,
* remove the first element of directionQ and assign it to direction
* add a copy of head to the body .. body.add(0,new Rectangle(head));
* there are 4 exclusive cases for the value of direction:
* .. direction is 4 (i.e. left)
* subtract size from head.x (this will change its x position)
* .. direction is 2 (i.e. down)
* add size to head.y
* .. etc.
*
* Task 3a: (in class SnakeGame)
* uncomment the lines that invoke setDirection and move methods on snake
* and complete method keyPressed()
* YOU SHOULD BE ABLE TO SEE snake MOVE AND CONTROL IT WITH THE ARROW KEYS
*
* Task 3a (in class Snake)
* continue in method: public void move()
*
* if bodyAdd is positive, simply decrement bodyAdd,
* otherwise remove the last Rectangle in ArrayList body
* YOU SHOULD BE ABLE TO SEE snake GROW!
*
* Task 4: (in class SnakeGame)
* uncomment nibble field and initialize it
* to a 20x20 Rectangle with random coordinates
* (note: the coordinates should be multiples of 20 .. the snake size)
* render nibble in method draw .. g.fill(nibble);
* in method actionPerformed()
* (use the intersects method of Rectangle nibble
* to invoke the grow method of snake)
* (i.e. if the snake head intersects the nibble)
* also generate new random coordinates for nibble
* YOU SHOULD BE ABLE TO PLAY THE SNAKE GAME AS AN INVINCIBLE snake!
*
* Task 5: (in class Snake)
* write method: public boolean isDead()
* returns true if head intersects any Rectangle in body or
* head has passed any window boundaries
* (example: head.x < 0 , head.y > 580, etc)
* otherwise, returns false
*
* Task 5a: (in class SnakeGame)
* in method actionPerformed()
* write an if statement that stops the Timer clock if the snake isDead
Step by Step Solution
There are 3 Steps involved in it
Step: 1
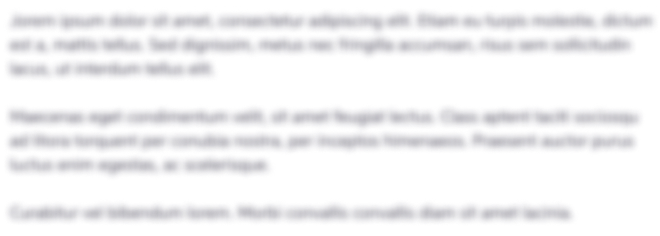
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started