Question
import java.awt.*; import java.awt.event.*; import javax.swing.*; public class Sort1 extends JFrame implements ActionListener { private TextField[] items = new TextField[6]; private JButton btnSort, btnClear, btnReset;
import java.awt.*; import java.awt.event.*; import javax.swing.*;
public class Sort1 extends JFrame implements ActionListener {
private TextField[] items = new TextField[6]; private JButton btnSort, btnClear, btnReset; private TextField tmp; private Label status;
private int pauseInterval = 100; // ms
public static void main(String[] args) { new Sort1().setVisible(true); }
public Sort1() { init(); }
public void init() { setTitle("Sorting Algorithms"); setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); setSize(440, 200); JPanel jp = new JPanel(new BorderLayout()); jp.setPreferredSize(new Dimension(440, 200)); jp.setBackground(Color.white);
JPanel itemPanel = new JPanel(); itemPanel.setBackground(Color.white); for (int i = 0; i
JPanel buttonPanel = new JPanel(); buttonPanel.setBackground(Color.white); btnSort = new JButton("Sort"); btnReset = new JButton("Reset"); btnClear = new JButton("Clear"); btnSort.addActionListener(this); btnClear.addActionListener(this); btnReset.addActionListener(this); buttonPanel.add(btnSort); buttonPanel.add(btnClear); buttonPanel.add(btnReset);
status = new Label("Wating ... "); status.setPreferredSize(new Dimension(380, 40)); JPanel statusPanel = new JPanel(); statusPanel.setBackground(Color.white); statusPanel.add(status, BorderLayout.SOUTH);
jp.add(itemPanel, BorderLayout.NORTH); jp.add(buttonPanel, BorderLayout.CENTER); jp.add(statusPanel, BorderLayout.SOUTH);
getContentPane().add(jp); }
private void initItems() { for (int i = 0; i
private void pause(int ms) { try { Thread.sleep(ms); } catch (InterruptedException e) { showStatus(e.toString()); } }
private void assign(TextField to, TextField from) { Color tobg = to.getBackground(); to.setBackground(Color.green); pause(pauseInterval); to.setText(from.getText()); pause(pauseInterval); to.setBackground(tobg); }
private void swapItems(TextField t1, TextField t2) { assign(tmp, t1); assign(t1, t2); assign(t2, tmp); }
private boolean greaterThan(TextField t1, TextField t2) { boolean greater; Color t1bg = t1.getBackground(); Color t2bg = t2.getBackground(); t1.setBackground(Color.cyan); t2.setBackground(Color.cyan); pause(pauseInterval); greater = Integer.parseInt(t1.getText()) > Integer.parseInt(t2.getText()); pause(pauseInterval); t1.setBackground(t1bg); t2.setBackground(t2bg); return greater; }
private void bubbleSort() { showStatus("Sorting ..."); boolean swap = true; while (swap) { swap = false; for (int i = 0; i
public void actionPerformed(ActionEvent e) { String command = e.getActionCommand(); switch (command) { case "Clear": for (int i = 0; i
private void showStatus(String s) { status.setText(s); }
}
Practical COMP1102/8702/1102 DE Semester 2, 2018 Task 2 1. Make sure you have saved the file you were working o for Task 1. 2. Change all occurrences of Sortl to Sort2 -There are three places where you have to do this 3. Save the file as Sort2.java 4. Modify the method bubbleSort so that it calculates and outputs, in the application's status label which appears at the bottom of the application's window, the number of swaps performed during sorting To count the number of swaps you will need to define an integer variable and initialise it to 0 It should be increased by one each time a swap is performed The output should be generated by modifying the following line which appears at the end of the method bubbleSort shoxStatus ("Sort complete"); Compile and run the application. After clicking the Sort button and once sorting is complete line of the following form should appear at the bottom of the application's window: Sorting complete, number of swaps7 To enter your own list to sort, click Clear and then type integers into each empty box. The following list should cause the above status line output to be produced: 3 4 9 17 1 2 Checkpoint 47 Have the program source code and output marked by a demonstratorStep by Step Solution
There are 3 Steps involved in it
Step: 1
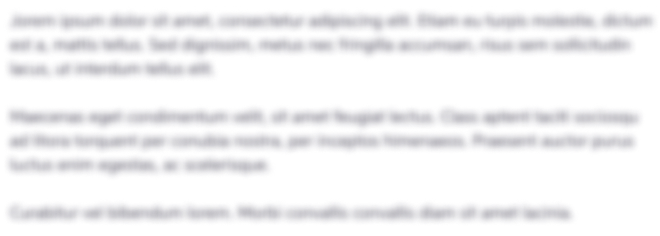
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started