Answered step by step
Verified Expert Solution
Question
1 Approved Answer
import javafx.beans.value.ChangeListener; import javafx.beans.value.ObservableValue; import javafx.geometry.Insets; import javafx.scene.Node; import javafx.scene.control.*; import javafx.scene.layout.GridPane; import javafx.stage.Stage; import javafx.util.Callback; public class AddDVDDialog extends Dialog { public AddDVDDialog(Stage owner,
import javafx.beans.value.ChangeListener; import javafx.beans.value.ObservableValue; import javafx.geometry.Insets; import javafx.scene.Node; import javafx.scene.control.*; import javafx.scene.layout.GridPane; import javafx.stage.Stage; import javafx.util.Callback; public class AddDVDDialog extends Dialog { public AddDVDDialog(Stage owner, String title, DVD d) { setTitle(title); // Set the button types ButtonType okButtonType = new ButtonType("OK", ButtonBar.ButtonData.OK_DONE); getDialogPane().getButtonTypes().addAll(okButtonType, ButtonType.CANCEL); // Create the labels and fields. GridPane grid = new GridPane(); grid.setHgap(10); grid.setVgap(10); grid.setPadding(new Insets(10, 10, 10, 10)); TextField titleField = new TextField(d.getTitle()); titleField.setPromptText("Name of movie/documentary/event/etc."); titleField.setMinWidth(300); TextField yearField = new TextField(); if (d.getYear() > 0) yearField.setText("" + d.getYear()); yearField.setPromptText("Year DVD was released"); yearField.setMinWidth(300); TextField lengthField = new TextField(); if (d.getDuration() > 0) lengthField.setText("" + d.getDuration()); lengthField.setPromptText("Length (in minutes) of movie/documentary/event/etc."); lengthField.setMinWidth(300); Label l1 = new Label("Title:"); Label l2 = new Label("Year:"); Label l3 = new Label("Length:"); grid.add(l1, 0, 0); grid.add(titleField, 1, 0); grid.add(l2, 0, 1); grid.add(yearField, 1, 1); grid.add(l3, 0, 2); grid.add(lengthField, 1, 2); getDialogPane().setContent(grid); // Puts the stuff on the window // Enable/Disable OK button depending on whether DVD data was entered. Node okButton = getDialogPane().lookupButton(okButtonType); okButton.setDisable(true); // Disable upon start titleField.textProperty().addListener(new ChangeListener() { public void changed(ObservableValue observable, Object oldValue, Object newValue) { okButton.setDisable(titleField.getText().trim().isEmpty() || yearField.getText().trim().isEmpty() || lengthField.getText().trim().isEmpty()); } }); yearField.textProperty().addListener(new ChangeListener() { public void changed(ObservableValue observable, Object oldValue, Object newValue) { okButton.setDisable(titleField.getText().trim().isEmpty() || yearField.getText().trim().isEmpty() || lengthField.getText().trim().isEmpty()); } }); lengthField.textProperty().addListener(new ChangeListener() { public void changed(ObservableValue observable, Object oldValue, Object newValue) { okButton.setDisable(titleField.getText().trim().isEmpty() || yearField.getText().trim().isEmpty() || lengthField.getText().trim().isEmpty()); } }); // Convert the result to a DVD setResultConverter(new Callback() { public DVD call(ButtonType b) { if (b == okButtonType) { d.setTitle(titleField.getText()); d.setYear(Integer.parseInt(yearField.getText())); d.setDuration(Integer.parseInt(lengthField.getText())); return d; } return null; } }); } }
import java.util.ArrayList; public class ArrayListTestProgram { public static void main(String args[]) { int total = 0; ArrayListnumbers; System.out.println("The ArrayList looks like this: " + numbers); System.out.println("It has " + numbers.size() + " elements in it"); System.out.println("The 5th element in it is: " + numbers.get(4)); } }
public class Customer { private int id; private String name; private int age; private char gender; private float money; // A simple constructor public Customer(String n, int a, char g, float m) { name = n; age = a; gender = g; money = m; id = -1; } // Return a String representation of the object public String toString() { String result; result = "Customer " + name + ": a " + age + " year old "; if (gender == 'F') result += "fe"; return result + "male with $" + money; } public boolean hasMoreMoneyThan(Customer c) { return money > c.money; } // Get methods public String getName() { return name; } public int getAge() { return age; } public char getGender() { return gender; } public int getId() { return id; } // Set Methods public void setId(int newID) { id = newID; } }
public class DVD implements Comparable { private String title; private int year; private int duration; public DVD () { this ("",0,0); } public DVD (String newTitle, int y, int minutes) { title = newTitle; year = y; duration = minutes; } public int compareTo(Object obj) { if (obj instanceof DVD) { DVD aDVD = (DVD)obj; return title.compareTo(aDVD.title); } return 0; } public String getTitle() { return title; } public int getDuration() { return duration; } public int getYear() { return year; } public void setTitle(String t) { title = t; } public void setDuration(int d) { duration = d; } public void setYear(int y) { year = y; } public String toString() { return ("DVD (" + year + "): \"" + title + "\" with length: " + duration + " minutes"); } }
import java.util.ArrayList; public class DVDCollection { private ArrayListdvds; private int selectedDVD; public DVDCollection() { dvds = new ArrayList (); selectedDVD = -1; } public void setSelectedDVD(int i) { selectedDVD = i; } public ArrayList getDvds() { return dvds; } public String toString() { return ("DVD Collection of size " + dvds.size()); } public void add(DVD aDvd) { dvds.add(aDvd); } public boolean remove(String title) { for (DVD d: dvds) { if (d.getTitle().equals(title)) { dvds.remove(d); return true; } } return false; } public void sortByTitle() { for (int p = 1; pdvds.size(); p++) { DVD key = dvds.get(p); int i = p - 1; while ((i >= 0) && (dvds.get(i).getTitle().compareTo(key.getTitle()) > 0)) { dvds.set(i+1, dvds.get(i)); i = i - 1; } dvds.set(i+1, key); } } public void sortByYear() { for (int p = 1; pdvds.size(); p++) { DVD key = dvds.get(p); int i = p - 1; while ((i >= 0) && (dvds.get(i).getYear() > key.getYear())) { dvds.set(i+1, dvds.get(i)); i = i - 1; } dvds.set(i+1, key); } } public void sortByLength() { for (int p = 1; p dvds.size(); p++) { DVD key = dvds.get(p); int i = p - 1; while ((i >= 0) && (dvds.get(i).getDuration() > key.getDuration())) { dvds.set(i+1, dvds.get(i)); i = i - 1; } dvds.set(i+1, key); } } public DVD oldestDVD() { DVD min = dvds.get(0); for (DVD d: dvds) { if (d.getYear() return min; } public DVD newestDVD() { DVD max = dvds.get(0); for (DVD d: dvds) { if (d.getYear() > max.getYear()) max = d; } return max; } public DVD shortestDVD() { DVD min = dvds.get(0); for (DVD d: dvds) { if (d.getDuration() return min; } public DVD longestDVD() { DVD max = dvds.get(0); for (DVD d: dvds) { if (d.getDuration() > max.getDuration()) max = d; } return max; } public static DVDCollection example1() { DVDCollection c = new DVDCollection(); c.add(new DVD("If I Was a Student", 2007, 65)); c.add(new DVD("Don't Eat Your Pencil !", 1984, 112)); c.add(new DVD("The Exam", 2001, 180)); c.add(new DVD("Tutorial Thoughts", 2003, 128)); c.add(new DVD("Fried Monitors", 1999, 94)); return c; } public static DVDCollection example2() { DVDCollection c = new DVDCollection(); c.add(new DVD("Titanic",1997,194)); c.add(new DVD("Star Wars",1977,121)); c.add(new DVD("E.T. - The Extraterrestrial",1982,115)); c.add(new DVD("Spider Man",2002,121)); c.add(new DVD("Jurassic Park",1993,127)); c.add(new DVD("Finding Nemo",2003,100)); c.add(new DVD("The Lion King",1994,89)); c.add(new DVD("Up",2009,96)); c.add(new DVD("The Incredibles",2004,115)); c.add(new DVD("Monsters Inc.",2001,92)); c.add(new DVD("Cars",2006,117)); c.add(new DVD("Beverly Hills Cop",1984,105)); c.add(new DVD("Home Alone",1990,103)); c.add(new DVD("Jaws",1975,124)); c.add(new DVD("Men in Black",1997,98)); c.add(new DVD("Independence Day",1996,145)); c.add(new DVD("National Treasure: Book of Secrets",2007,124)); c.add(new DVD("Aladdin",1992,90)); c.add(new DVD("Twister",1996,113)); c.add(new DVD("Iron Man",2008,126)); c.add(new DVD("Alice in Wonderland",2010,108)); c.add(new DVD("Transformers",2007,144)); return c; } public static DVDCollection example3() { DVDCollection c = new DVDCollection(); c.add(new DVD("Avatar",2009,162)); c.add(new DVD("The Avengers",2012,143)); c.add(new DVD("Titanic",1997,194)); c.add(new DVD("The Dark Knight",2008,152)); c.add(new DVD("Star Wars",1977,121)); c.add(new DVD("Shrek 2",2004,93)); c.add(new DVD("E.T. - The Extraterrestrial",1982,115)); c.add(new DVD("Star Wars - Episode I",1999,136)); c.add(new DVD("Pirates of the Caribbean: Dead Man's Chest",2006,151)); c.add(new DVD("Toy Story 3",2010,103)); c.add(new DVD("Spider Man",2002,121)); c.add(new DVD("Transformers: Revenge of the Fallen",2009,150)); c.add(new DVD("Star Wars - Episode III",2005,140)); c.add(new DVD("Spider Man 2",2004,127)); c.add(new DVD("Jurassic Park",1993,127)); c.add(new DVD("Transformers: Dark of the Moon",2011,154)); c.add(new DVD("Finding Nemo",2003,100)); c.add(new DVD("Spider Man 3",2007,139)); c.add(new DVD("Forrest Gump",1994,142)); c.add(new DVD("The Lion King",1994,89)); c.add(new DVD("Shrek the Third",2007,93)); c.add(new DVD("The Sixth Sense",1999,107)); c.add(new DVD("Up",2009,96)); c.add(new DVD("I am Legend",2007,101)); c.add(new DVD("Shrek",2001,90)); c.add(new DVD("The Incredibles",2004,115)); c.add(new DVD("Monsters Inc.",2001,92)); c.add(new DVD("Brave",2012,100)); c.add(new DVD("Toy Story 2",1999,92)); c.add(new DVD("Cars",2006,117)); c.add(new DVD("Signs",2002,106)); c.add(new DVD("Hancock",2008,92)); c.add(new DVD("Rush Hour 2",2001,90)); c.add(new DVD("Meet the Fockers",2004,115)); c.add(new DVD("The Hangover",2009,100)); c.add(new DVD("My Big Fat Greek Wedding",2002,95)); c.add(new DVD("Beverly Hills Cop",1984,105)); c.add(new DVD("Mrs. Doubtfire",1993,125)); c.add(new DVD("The Hangover Part II",2011,102)); c.add(new DVD("The Matrix Reloaded",2003,138)); c.add(new DVD("Home Alone",1990,103)); c.add(new DVD("Jaws",1975,124)); c.add(new DVD("The Blind Side",2009,129)); c.add(new DVD("Men in Black",1997,98)); c.add(new DVD("X-Men: The Last Stand",2006,104)); c.add(new DVD("The Bourne Ultimatum",2007,115)); c.add(new DVD("WALL-E",2008,98)); c.add(new DVD("Inception",2010,148)); c.add(new DVD("Independence Day",1996,145)); c.add(new DVD("Pirates of the Caribbean: The Curse of the Black Pearl",2003,143)); c.add(new DVD("The Chronicles of Narnia",2005,143)); c.add(new DVD("War of the Worlds",2005,116)); c.add(new DVD("National Treasure: Book of Secrets",2007,124)); c.add(new DVD("Aladdin",1992,90)); c.add(new DVD("How to Train Your Dragon",2010,98)); c.add(new DVD("Shrek Forever After",2010,93)); c.add(new DVD("Twister",1996,113)); c.add(new DVD("Star Wars - Episode VI",1983,134)); c.add(new DVD("Iron Man",2008,126)); c.add(new DVD("Alice in Wonderland",2010,108)); c.add(new DVD("Transformers",2007,144)); c.add(new DVD("Star Wars - Episode II",2002,142)); return c; } }
import javafx.application.Application; import javafx.collections.FXCollections; import javafx.event.ActionEvent; import javafx.event.EventHandler; import javafx.geometry.HPos; import javafx.geometry.Insets; import javafx.scene.Scene; import javafx.scene.control.*; import javafx.scene.input.MouseEvent; import javafx.scene.layout.GridPane; import javafx.scene.layout.VBox; import javafx.stage.Stage; import java.util.ArrayList; import java.util.Optional; public class DVDCollectionApp extends Application { private DVDCollection model; private ListViewtList; private TextField tField, yField, lField; public DVDCollectionApp() { model = DVDCollection.example2(); } public void start(Stage primaryStage) { VBox topPane = new VBox(); GridPane aPane = new GridPane(); aPane.setPadding(new Insets(10, 10, 10, 10)); // Create the labels Label label1 = new Label("DVDs"); aPane.add(label1,0,0,1,1); aPane.setMargin(label1, new Insets(0, 0, 5, 0)); Label label2 = new Label("Title"); aPane.add(label2,0,2,1,1); Label label3 = new Label("Year"); aPane.add(label3,0,3,1,1); Label label4 = new Label("Length"); aPane.setMargin(label4, new Insets(0, 10, 0, 10)); aPane.add(label4,2,3,1,1); // Create the TextFields tField = new TextField(); aPane.add(tField,1,2,6,1); aPane.setMargin(tField, new Insets(10, 0, 10, 0)); tField.setMinSize(500,30); tField.setPrefSize(2000,30); yField = new TextField(); aPane.add(yField,1,3,1,1); yField.setPrefSize(55,30); lField = new TextField(); aPane.add(lField,3,3,1,1); lField.setPrefSize(45,30); aPane.setMargin(lField, new Insets(0, 10, 0, 0)); // Create the list tList = new ListView (); aPane.add(tList,0,1,7,1); tList.setMinSize(540,150); tList.setPrefSize(2000,2000); /// Create the buttons Button addButton = new Button("Add"); addButton.setStyle("-fx-font: 12 arial; -fx-base: rgb(0,100,0); -fx-text-fill: rgb(255,255,255);"); aPane.add(addButton,4,3,1,1); addButton.setPrefSize(90,30); aPane.setMargin(addButton, new Insets(0, 5, 0, 5)); Button deleteButton = new Button("Delete"); deleteButton.setStyle("-fx-font: 12 arial; -fx-base: rgb(200,0,0); -fx-text-fill: rgb(255,255,255);"); aPane.add(deleteButton,5,3,1,1); deleteButton.setPrefSize(90,30); aPane.setMargin(deleteButton, new Insets(0, 5, 0, 5)); Button statsButton = new Button("Stats"); statsButton.setStyle("-fx-font: 12 arial;"); aPane.add(statsButton,6,3,1,1); statsButton.setPrefSize(90,30); aPane.setMargin(statsButton, new Insets(0, 0, 0, 5)); aPane.setHalignment(statsButton, HPos.RIGHT); // Populate the lists ArrayList theList = model.getDvds(); tList.setItems(FXCollections.observableArrayList(theList)); // Add all the components to the Pane aPane.setPrefSize(548, 268); Menu fileMenu = new Menu("_File"); MenuItem exampleItem = new MenuItem("Load Example List"); fileMenu.getItems().addAll(exampleItem); Menu sortMenu = new Menu("_Sort"); RadioMenuItem titleItem = new RadioMenuItem("By Title"); RadioMenuItem yearItem = new RadioMenuItem("By Year"); RadioMenuItem lengthItem = new RadioMenuItem("By Length"); ToggleGroup settingGroup = new ToggleGroup(); titleItem.setToggleGroup(settingGroup); yearItem.setToggleGroup(settingGroup); lengthItem.setToggleGroup(settingGroup); sortMenu.getItems().addAll(titleItem, yearItem, lengthItem); // Add the menus to a menubar and then add the menubar to the pane MenuBar menuBar = new MenuBar(); menuBar.getMenus().addAll(fileMenu, sortMenu); topPane.getChildren().addAll(menuBar, aPane); exampleItem.setOnAction(new EventHandler () { public void handle(ActionEvent e) { TextInputDialog dialog = new TextInputDialog("1"); dialog.setTitle("Input Required"); dialog.setHeaderText(null); dialog.setContentText("Enter example list number to load (1, 2, or 3):"); Optional result = dialog.showAndWait(); if (result.isPresent()){ switch(Integer.parseInt(result.get())) { case 1: model = DVDCollection.example1(); break; case 2: model = DVDCollection.example2(); break; case 3: model = DVDCollection.example3(); break; } ArrayList theList = model.getDvds(); tList.setItems(FXCollections.observableArrayList(theList)); } } }); addButton.setOnAction(new EventHandler () { public void handle(ActionEvent actionEvent) { DVD d = new DVD(); // Now bring up the dialog box Dialog dialog = new AddDVDDialog(primaryStage, "New DVD Details", d); Optional result = dialog.showAndWait(); if (result.isPresent()) { model.add(d); // Add the DVD to the model update(model, model.getDvds().size() - 1); } } }); statsButton.setOnAction(new EventHandler () { public void handle(ActionEvent actionEvent) { // Now bring up the dialog box Dialog dialog = new StatsDialog(primaryStage, model); dialog.showAndWait(); } }); deleteButton.setOnAction(new EventHandler () { public void handle(ActionEvent actionEvent) { if (tList.getSelectionModel().getSelectedItem() != null) { Alert alert = new Alert(Alert.AlertType.CONFIRMATION); alert.setTitle("Please Confirm"); alert.setHeaderText(null); alert.setContentText("Are you sure that you want to remove this DVD from the collection ?"); Optional result = alert.showAndWait(); if (result.get() == ButtonType.OK){ model.remove(tList.getSelectionModel().getSelectedItem().getTitle()); update(model, -1); } // model.remove(tList.getSelectionModel().getSelectedItem().getTitle()); //update(model, tList.getSelectionModel().getSelectedIndex()); } } }); tList.setOnMousePressed(new EventHandler () { public void handle(MouseEvent mouseEvent) { model.setSelectedDVD(tList.getSelectionModel().getSelectedIndex()); update(model, tList.getSelectionModel().getSelectedIndex()); } }); titleItem.setOnAction(new EventHandler () { public void handle(ActionEvent e) { model.sortByTitle(); ArrayList theList = model.getDvds(); tList.setItems(FXCollections.observableArrayList(theList)); update(model, tList.getSelectionModel().getSelectedIndex()); } }); yearItem.setOnAction(new EventHandler () { public void handle(ActionEvent e) { model.sortByYear(); ArrayList theList = model.getDvds(); tList.setItems(FXCollections.observableArrayList(theList)); update(model, tList.getSelectionModel().getSelectedIndex()); } }); lengthItem.setOnAction(new EventHandler () { public void handle(ActionEvent e) { model.sortByLength(); ArrayList theList = model.getDvds(); tList.setItems(FXCollections.observableArrayList(theList)); update(model, tList.getSelectionModel().getSelectedIndex()); } }); primaryStage.setTitle("My DVD Collection"); primaryStage.setScene(new Scene(topPane)); primaryStage.show(); } // Update the view to reflect the model public void update(DVDCollection model, int selectedDVD) { ArrayList theList = model.getDvds(); tList.setItems(FXCollections.observableArrayList(theList)); DVD d = tList.getSelectionModel().getSelectedItem(); if (d != null) { tField.setText(d.getTitle()); yField.setText(""+d.getYear()); lField.setText(""+d.getDuration()); } else { tField.setText(""); yField.setText(""); lField.setText(""); } tList.getSelectionModel().select(selectedDVD); } public static void main(String[] args) { launch(args); } }
public class Mall { public static final int MAX_STORES = 100; private String name; private Store[] stores; private int storeCount; public Mall(String n) { name = n; stores = new Store[MAX_STORES]; storeCount = 0; } public void addStore(Store s) { if (storeCount MAX_STORES) { stores[storeCount++] = s; } } public boolean shoppedAtSameStore(Customer c1, Customer c2) { // Go through each store for (int i=0; istoreCount; i++) { Customer[] customersInStore = stores[i].getCustomers(); int numInStore = stores[i].getCustomerCount(); boolean foundC1 = false, foundC2 = false; for (int j=0; jif (customersInStore[j] == c1) foundC1 = true; if (customersInStore[j] == c2) foundC2 = true; } if (foundC1 && foundC2) return true; } return false; } // Determine the total number of unique customers that have visited the mall public int uniqueCustomers() { // First determine how many customers are in the stores int total = 0; for (int i=0; istoreCount; i++) { total += stores[i].getCustomerCount(); } // Now go through and add all the customers to an array, as long as they are not already in there // Keep track of unique ones int unique = 0; Customer[] uniqueCustomers = new Customer[total]; for (int i=0; istoreCount; i++) { Customer[] customersInStore = stores[i].getCustomers(); int numInStore = stores[i].getCustomerCount(); for (int j=0; j boolean found = false; for(int k=0; k if (customersInStore[j] == uniqueCustomers[k]) found = true; } if (!found) { uniqueCustomers[unique] = customersInStore[j]; unique++; } } } return unique; } }
public class MallTestProgram { public static void main(String args[]) { // Make the mall Mall trainyards = new Mall("Trainyards"); // Make some stores Store walmart, dollarama, michaels, farmBoy; trainyards.addStore(walmart = new Store("Walmart")); trainyards.addStore(dollarama = new Store("Dollarama")); trainyards.addStore(michaels = new Store("Michaels")); trainyards.addStore(farmBoy = new Store("Farm Boy")); // Create the customers Customer c1, c2, c3, c4, c5, c6, c7, c8, c9, c10, c11, c12, c13, c14; Customer c15, c16, c17, c18, c19, c20, c21, c22, c23, c24, c25, c26; c1 = new Customer("Amie", 14, 'F', 100); c2 = new Customer("Brad", 15, 'M', 0); c3 = new Customer("Cory", 10, 'M', 100); c4 = new Customer("Dave", 5, 'M', 48); c5 = new Customer("Earl", 21, 'M', 500); c6 = new Customer("Flem", 18, 'M', 1); c7 = new Customer("Gary", 8, 'M', 20); c8 = new Customer("Hugh", 65, 'M', 30); c9 = new Customer("Iggy", 43, 'M', 74); c10 = new Customer("Joan", 55, 'F', 32); c11 = new Customer("Kyle", 16, 'M', 88); c12 = new Customer("Lore", 12, 'F', 1000); c13 = new Customer("Mary", 17, 'F', 6); c14 = new Customer("Nick", 13, 'M', 2); c15 = new Customer("Omar", 18, 'M', 24); c16 = new Customer("Patt", 24, 'F', 45); c17 = new Customer("Quin", 42, 'M', 355); c18 = new Customer("Ruth", 45, 'F', 119); c19 = new Customer("Snow", 74, 'F', 20); c20 = new Customer("Tamy", 88, 'F', 25); c21 = new Customer("Ulsa", 2, 'F', 75); c22 = new Customer("Vern", 9, 'M', 90); c23 = new Customer("Will", 11, 'M', 220); c24 = new Customer("Xeon", 17, 'F', 453); c25 = new Customer("Ying", 19, 'F', 76); c26 = new Customer("Zack", 22, 'M', 35); // Add the customers to the stores walmart.addCustomer(c1); walmart.addCustomer(c3); walmart.addCustomer(c4); walmart.addCustomer(c5); walmart.addCustomer(c8); walmart.addCustomer(c12); walmart.addCustomer(c13); walmart.addCustomer(c14); walmart.addCustomer(c17); walmart.addCustomer(c19); walmart.addCustomer(c25); dollarama.addCustomer(c2); dollarama.addCustomer(c3); dollarama.addCustomer(c5); dollarama.addCustomer(c6); dollarama.addCustomer(c13); dollarama.addCustomer(c16); dollarama.addCustomer(c18); dollarama.addCustomer(c19); dollarama.addCustomer(c20); michaels.addCustomer(c1); michaels.addCustomer(c2); michaels.addCustomer(c7); michaels.addCustomer(c9); michaels.addCustomer(c15); michaels.addCustomer(c18); michaels.addCustomer(c22); michaels.addCustomer(c23); michaels.addCustomer(c24); michaels.addCustomer(c26); farmBoy.addCustomer(c1); farmBoy.addCustomer(c2); farmBoy.addCustomer(c5); farmBoy.addCustomer(c10); farmBoy.addCustomer(c11); farmBoy.addCustomer(c19); farmBoy.addCustomer(c21); farmBoy.addCustomer(c24); farmBoy.addCustomer(c25); // Determine whether or not certain customers shopped at the same store System.out.println("Did Amie and Xeon shop at the same store: " + trainyards.shoppedAtSameStore(c1, c24)); System.out.println("Did Brad and Nick shop at the same store: " + trainyards.shoppedAtSameStore(c2, c14)); // Determine the number of unique Customers in the mall System.out.println("The number of unique customers in the mall is: " + trainyards.uniqueCustomers()); } }
import javafx.geometry.Insets; import javafx.scene.control.*; import javafx.scene.layout.GridPane; import javafx.stage.Stage; public class StatsDialog extends Dialog { public StatsDialog(Stage owner, DVDCollection c) { setTitle("DVD Statistics"); // Set the button types ButtonType okButtonType = new ButtonType("OK", ButtonBar.ButtonData.OK_DONE); getDialogPane().getButtonTypes().addAll(okButtonType); // Create the labels and fields. GridPane grid = new GridPane(); grid.setHgap(10); grid.setVgap(10); grid.setPadding(new Insets(10, 10, 10, 10)); Label l1 = new Label("Total:"); Label l2 = new Label("Oldest:"); Label l3 = new Label("Newest:"); Label l4 = new Label("Shortest Duration:"); Label l5 = new Label("Longest Duration:"); Label l6 = new Label(""+c.getDvds().size()); Label l7 = new Label(""+c.oldestDVD()); Label l8 = new Label(""+c.newestDVD()); Label l9 = new Label(""+c.shortestDVD()); Label l10 = new Label(""+c.longestDVD()); l1.setStyle("-fx-font: 12 arial; -fx-text-fill: NAVY;"); l2.setStyle("-fx-font: 12 arial; -fx-text-fill: NAVY;"); l3.setStyle("-fx-font: 12 arial; -fx-text-fill: NAVY;"); l4.setStyle("-fx-font: 12 arial; -fx-text-fill: NAVY;"); l5.setStyle("-fx-font: 12 arial; -fx-text-fill: NAVY;"); grid.add(l1, 0, 0); grid.add(l2, 0, 1); grid.add(l3, 0, 2); grid.add(l4, 0, 3); grid.add(l5, 0, 4); grid.add(l6, 1, 0); grid.add(l7, 1, 1); grid.add(l8, 1, 2); grid.add(l9, 1, 3); grid.add(l10, 1, 4); getDialogPane().setContent(grid); // Puts the stuff on the window } }
public class Store { public static final int MAX_CUSTOMERS = 500; public static int LATEST_ID = 1000000; private String name; private Customer[] customers; private int customerCount; public Store(String n) { name = n; customers = new Customer[MAX_CUSTOMERS]; customerCount = 0; } public void addCustomer(Customer c) { if (customerCount MAX_CUSTOMERS) { customers[customerCount++] = c; c.setId(LATEST_ID++); } } public Customer[] getCustomers() { return customers; } public int getCustomerCount() { return customerCount; } public void listCustomers() { for (int i=0; icustomerCount; i++) System.out.println(customers[i]); } public int averageCustomerAge() { int avg = 0; for (int i=0; icustomerCount; i++) { avg += customers[i].getAge(); } return avg / customerCount; } public char mostPopularGender() { int mCount = 0, fCount = 0; for (int i=0; icustomerCount; i++) { if (customers[i].getGender() == 'M') mCount++; else fCount++; } if (mCount > fCount) return 'M'; return 'F'; } public Customer richestCustomer() { Customer richest = customers[0]; for (int i=1; icustomerCount; i++) { if (customers[i].hasMoreMoneyThan(richest)) richest = customers[i]; } return richest; } public Customer[] getCustomersWithGender(char g) { int matchCount = 0; // Count them first for (int i=0; icustomerCount; i++) { if (customers[i].getGender() == g) matchCount++; } // Now make the array Customer[] matches = new Customer[matchCount]; matchCount = 0; for (int i=0; icustomerCount; i++) { if (customers[i].getGender() == g) matches[matchCount++] = customers[i]; } return matches; } public Customer[] friendsFor(Customer lonelyCustomer) { int friendCount = 0; // Count them first for (int i=0; icustomerCount; i++) { if ((customers[i] != lonelyCustomer) && (customers[i].getGender() == lonelyCustomer.getGender()) && (Math.abs(customers[i].getAge() - lonelyCustomer.getAge()) // Now make the array Customer[] friends = new Customer[friendCount]; friendCount = 0; for (int i=0; icustomerCount; i++) { if ((customers[i] != lonelyCustomer) && (customers[i].getGender() == lonelyCustomer.getGender()) && (Math.abs(customers[i].getAge() - lonelyCustomer.getAge()) customers[i]; } } return friends; } }
public class StoreTestProgram { public static void main(String args[]) { Customer[] result; Store walmart; walmart = new Store("Walmart off Innes"); walmart.addCustomer(new Customer("Amie", 14, 'F', 100)); walmart.addCustomer(new Customer("Brad", 15, 'M', 0)); walmart.addCustomer(new Customer("Cory", 10, 'M', 100)); walmart.addCustomer(new Customer("Dave", 5, 'M', 48)); walmart.addCustomer(new Customer("Earl", 21, 'M', 500)); walmart.addCustomer(new Customer("Flem", 18, 'M', 1)); walmart.addCustomer(new Customer("Gary", 8, 'M', 20)); walmart.addCustomer(new Customer("Hugh", 65, 'M', 30)); walmart.addCustomer(new Customer("Iggy", 43, 'M', 74)); walmart.addCustomer(new Customer("Joan", 55, 'F', 32)); walmart.addCustomer(new Customer("Kyle", 16, 'M', 88)); walmart.addCustomer(new Customer("Lore", 12, 'F', 1000)); walmart.addCustomer(new Customer("Mary", 17, 'F', 6)); walmart.addCustomer(new Customer("Nick", 13, 'M', 2)); walmart.addCustomer(new Customer("Omar", 18, 'M', 24)); walmart.addCustomer(new Customer("Patt", 24, 'F', 45)); walmart.addCustomer(new Customer("Quin", 42, 'M', 355)); walmart.addCustomer(new Customer("Ruth", 45, 'F', 119)); walmart.addCustomer(new Customer("Snow", 74, 'F', 20)); walmart.addCustomer(new Customer("Tamy", 88, 'F', 25)); walmart.addCustomer(new Customer("Ulsa", 2, 'F', 75)); walmart.addCustomer(new Customer("Vern", 9, 'M', 90)); walmart.addCustomer(new Customer("Will", 11, 'M', 220)); walmart.addCustomer(new Customer("Xeon", 17, 'F', 453)); walmart.addCustomer(new Customer("Ying", 19, 'F', 76)); walmart.addCustomer(new Customer("Zack", 22, 'M', 35)); System.out.println("Here are the customers: "); walmart.listCustomers(); // Find average Customer age System.out.println(" Average age of customers: " + walmart.averageCustomerAge()); // Find most popular gender System.out.println(" Most popular gender is: " + walmart.mostPopularGender()); // Find richest customer System.out.println(" Richest customer is: " + walmart.richestCustomer()); // Find male customers System.out.println(" Here are all the male customers:"); result = walmart.getCustomersWithGender('M'); for (Customer c: result) System.out.println(c); // Find female customers System.out.println(" Here are all the female customers:"); result = walmart.getCustomersWithGender('F'); for (Customer c: result) System.out.println(c); // Find friends for Amie System.out.println(" Friends for 14 year old female Amie:"); result = walmart.friendsFor(walmart.getCustomers()[0]); for (Customer c: result) System.out.println(c); // Find friends for Brad System.out.println(" Friends for 15 year old male Brad:"); result = walmart.friendsFor(walmart.getCustomers()[1]); for (Customer c: result) System.out.println(c); } }
Let's begin by familiarizing ourselves with the use of ArrayList objects. Examine the class called ArrayListTestProgram. It does not compile. The compiler will complain, stating that Variable 'numbers' might not have been initialized. Fix this by adding the following line of code before the println statements: numbersnew ArrayList
Step by Step Solution
There are 3 Steps involved in it
Step: 1
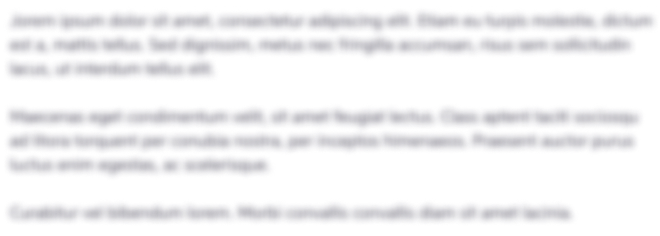
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started