Question
import java.util.*; import java.io.BufferedInputStream; import java.io.BufferedOutputStream; import java.io.DataInputStream; import java.io.DataOutputStream; import java.io.File; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.io.OutputStream; import java.io.PrintWriter; import
import java.util.*;
import java.io.BufferedInputStream; import java.io.BufferedOutputStream; import java.io.DataInputStream; import java.io.DataOutputStream; import java.io.File; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.FileOutputStream; import java.io.IOException; import java.io.OutputStream; import java.io.PrintWriter; import java.io.RandomAccessFile; import java.security.DigestInputStream; import java.security.MessageDigest; import java.security.NoSuchAlgorithmException; import java.util.Arrays; import static javafx.scene.input.KeyCode.M;
public class ExternalSort {
public static void sort(String f1, String f2) throws Exception { RandomAccessFile raf1 = new RandomAccessFile(f1, "rw"); RandomAccessFile raf2 = new RandomAccessFile(f2, "rw"); int fileByteSize = (int) (raf1.length() / 4); int size = Math.min(1000000, fileByteSize); externalSort(f1, f2, size); boolean writeToOriginal = true; DataOutputStream dos; while (size <= fileByteSize) { if (writeToOriginal) { raf1.seek(0); dos = new DataOutputStream(new BufferedOutputStream(new FileOutputStream(raf1.getFD()))); } else { raf2.seek(0); dos = new DataOutputStream(new BufferedOutputStream(new FileOutputStream(raf2.getFD()))); } for (int i = 0; i < fileByteSize; i += 2 * size) { if (writeToOriginal) { dos = merge(f2, dos, i, size); } else { dos = merge(f1, dos, i, size); } } dos.flush(); writeToOriginal = !writeToOriginal; size *= 2; } if (writeToOriginal) { raf1.seek(0); raf2.seek(0); dos = new DataOutputStream(new BufferedOutputStream(new FileOutputStream(raf1.getFD()))); int i = 0; while (i < raf2.length() / 4){ dos.writeInt(raf2.readInt()); i++; } dos.flush(); } }
public static void externalSort(String f1, String f2, int size) throws Exception{
RandomAccessFile raf1 = new RandomAccessFile(f1, "rw"); RandomAccessFile raf2 = new RandomAccessFile(f2, "rw");
int fileByteSize = (int) (raf1.length() / 4);
int[] array = new int[size]; DataInputStream dis = new DataInputStream(new BufferedInputStream( new FileInputStream(raf1.getFD()))); DataOutputStream dos = new DataOutputStream(new BufferedOutputStream( new FileOutputStream(raf2.getFD())));
int count = 0; while (count < fileByteSize){ for (int k = 0; k < size; ++k){ array[k] = dis.readInt(); } count += size; Arrays.sort(array); for (int k = 0; k < size; ++k){ dos.writeInt(array[k]); } } dos.flush(); raf1.close(); raf2.close(); dis.close(); dos.close(); }
public static DataOutputStream merge(String file, DataOutputStream dos, int start, int size) throws IOException { RandomAccessFile raf = new RandomAccessFile(file, "rw"); RandomAccessFile raf2 = new RandomAccessFile(file, "rw");
int fileByteSize = (int) (raf.length() / 4); raf.seek(4 * start); raf2.seek(4 *start); DataInputStream dis = new DataInputStream(new BufferedInputStream(new FileInputStream(raf.getFD()))); DataInputStream dis3 = new DataInputStream(new BufferedInputStream(new FileInputStream(raf2.getFD()))); int i = 0; int j = 0; int max = size * 2;
int a = dis.readInt();
int b; if (start + size < fileByteSize) { dis3.skip(4 * size); b = dis3.readInt(); } else { b = Integer.MAX_VALUE; j = size; } while (i + j < max) { if (j == size || (a <= b && i != size)) { dos.writeInt(a); i++; if (start + i == fileByteSize) { i = size; } else if (i != size) { a = dis.readInt(); } } else { dos.writeInt(b); j++; if (start + size + j == fileByteSize) { j = size; } else if (j != size) {
b = dis3.readInt(); } } } raf.close(); raf2.close(); return dos; }
private int[] minheap; private int j; private int heapsize; private int i; private String fname; private int lastwritten; private int inheap; private String[] arr;
private void twowaymerge () throws FileNotFoundException {// pseudo code
Scanner F1 = new Scanner(new File("File01.txt")); Scanner F2 = new Scanner(new File("File02.txt")); PrintWriter F3 =new PrintWriter(new File("File03.txt")); // read an input from file F1 int x = F1.nextInt(); // read an input from file F2 int y = F2. nextInt(); while (F1.hasNext() && F2.hasNext()) { if (x < y ) { F3.print(x + " "); //read an input from file F1 x = F1.nextInt(); } else {
F3.print(y + " "); //read an input from file F2 y = F2.nextInt(); } } // endwhile while (F1.hasNext()) { F3.print(x + " "); //read an input from file F1 x = F1.nextInt(); } // endwhile while (F2.hasNext()) { F3.print(y + " "); //read an input from file F2 y= F2.nextInt(); } // endwhile // close all files F1.close(); F2.close(); F2.close(); } // end two way-merge
private int replacementselectionsort () throws FileNotFoundException {// // open input file inf Scanner inf = new Scanner(System.in); // read array size M for min heap array int M = inf.nextInt(); // allocate array space for min heap // Note min heap starts from position 1 minheap = new int[M+1];// // fill intial minheap from infile for(j=1; j<=M&&inf.hasNext();j++)// read next input from infile minheap[j]=inf.nextInt(); // endfor // no. of elements in heap heapsize = --j; // No. of data in minheap // output file no. i = 1; // outer while while (heapsize > 0) { // while more data exist // create output file i fname = "File0" + i +".txt"; // open output file[i] PrintWriter opf = new PrintWriter(new File(fname)); //convert to minheap for (j = heapsize/2; j > 0; j--) minheapdn (j, heapsize); // Initialize last written lastwritten= Integer.MIN_VALUE; //Integer.MIN_VALUE in java inheap = 0; // unprocessed elements // write minheap[1] to output file[i] and read next data // if not end of file read next input // else shift minheap element to left and reduce heap size // inner while while ( heapsize > 0) { // root can be written to current output file[i] if (minheap[1] < lastwritten) { swap (minheap[1], minheap[heapsize]); // heapsize --; // reduce heap size //increase unprocessed elements in heap inheap ++; } else { lastwritten = minheap[1]; // write min to output file[i] opf.print(arr[j] + " "); // if end of file shift elements to left in minheap if (!inf.hasNext()){ // for ( j = 1; j < heapsize+inheap; j ++) minheap[j] = minheap[j+1]; // end for heapsize --; // reduce heap size }// end if else { // read next input from infile minheap [1] = inf.nextInt(); }// end inner else
}//end outer else // heap down from root; minheapdn(1, heapsize); } // end inner while opf.close(); // close this file since it no more can be added if (inheap == 0) break; // all data are processed heapsize = inheap; // i ++; // start another output file } // end outer while inf.close (); // close input file return i; // No. of files } // end replacementselectionsort
private void minheapdn(int j, int heapsize) { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
private void swap(int i, int i0) { throw new UnsupportedOperationException("Not supported yet."); //To change body of generated methods, choose Tools | Templates. }
public static void main(String args[]) throws Exception{
System.out.print(" Program to:"); System.out.print(" Prepared by Last: , First: and Student ID: ."); System.out.println(" Date: "); xxxxxp2 extsort = new xxxxxp2 ();
try{
k = extsort extsort.gensortedfiles(); // k sorted files will be generated
for(i=1; i<=k;i++) extsort.printfile(F + i+ .txt); // Print content of file i
// end for
extsort.mergefiles(k); // merge k sorted files F1, F2,Fk, using 2way merge
extsort.printfile(F.txt); // Print final sorted file
}catch (Exception e) {prt(" Exception " + e + " ");} }
}
the program is external sort class and i used 2 way merge sort and replacement selection but i need help to write the main method for the class
if i input these integers 5 63 60 16 47 8 12 50 21 67 7 35 27 20 24 18 32 32 18 24 15
by using sorting phase (using replacement selection) of java program (xxxxxp2.java) should generate 3 sorted files as follows:
F1: 8 12 16 21 47 50 60 63 67
F2: 7 18 20 24 27 32 32 35
F3:15 18 24
Step by Step Solution
There are 3 Steps involved in it
Step: 1
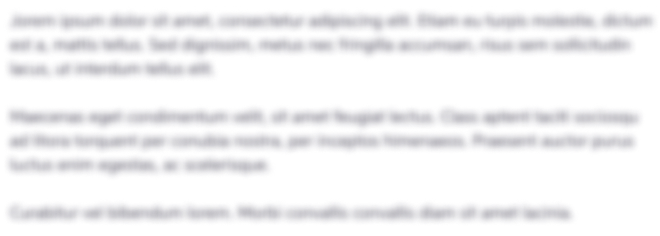
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started