Answered step by step
Verified Expert Solution
Question
1 Approved Answer
import java.util.ArrayList; / * * * A class to track a student's name and a list of numeric grades for * that student. * *
import java.util.ArrayList;
A class to track a student's name and a list of numeric grades for
that student.
@author Jim Teresco
@version Fall
public class StudentGrades
we have a student's name and a list of that student's grades
as the fields for this class
private String name;
private ArrayList grades;
Construct a new student, who has no grades when starting out.
@param name The student's name.
public StudentGradesString name
this.name name;
grades new ArrayList;
Add a grade to a for the student.
@param The grade to add to this student's grade list.
public void addGradedouble grade
grades.addgrade;
Get this student's name.
@return The student's name.
public String getName
return name;
Determine equality of StudentGrades objects, defined such that two StudentGrades objects
are equal if they have the same name field
@param obj The other object.
@return true If this and obj have the same name field.
public boolean equalsObject obj
StudentGrades other StudentGrades obj;
return other.name.equalsname;
Returns a string representing the StudentGrade object.
@return Returns a string representing the StudentGrade object.
@Override
public String toString
StringBuilder s new StringBuildername :;
for double score : grades
sappend score;
return stoString;
import java.util.ArrayList;
import java.util.Scanner;
Example CourseGrades: a program to keep track of course
grades for a group of students. Uses ArrayLists in a few
ways.
@author Jim Teresco
@version Fall
public class CourseGrades
We will have some instance variables to track the
contents of the list of students and their grades
and a set of methods to interact with the list, which
is done from main, below.
private ArrayList studentList;
Construct an empty CourseGrades.
public CourseGrades
studentList new ArrayList;
Method to add a grade for a possibly new student.
@param name The student's name.
@param grade The grade to add for this student.
public void addString name, double grade
StudentGrades sg findStudentGradesByNamename;
if sg null
sgaddGradegrade;
else
sg new StudentGradesname;
sgaddGradegrade;
studentList.addsg;
Print out all of the students and their grades.
public void printAll
for StudentGrades sg : studentList
System.out.printlnsg;
Private helper method to look up the StudentGrades object
from the list that matches the name. Returns null if none.
private StudentGrades findStudentGradesByNameString name
for StudentGrades sg : studentList
if sggetNameequalsname return sg;
return null;
This is the main method for your program.
@param args No command line arguments are required.
public static void mainString args
CourseGrades cg new CourseGrades;
cgaddLinus;
cgaddSnoopy;
cgaddLinus;
make memory diagram for main method of the CourseGrades class is executed and the program is currently executing
the last line in the main method:
cgaddLinus;
right before the call it makes to
sgaddGradegrade;
returns.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
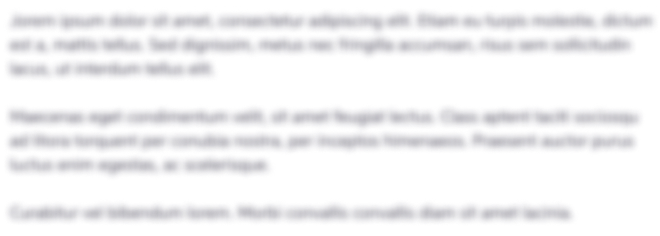
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started