Answered step by step
Verified Expert Solution
Question
1 Approved Answer
import java.util.concurrent.Semaphore; class Buffer { private int data; private Semaphore mutex; private Semaphore empty; private Semaphore full; public Buffer ( ) { / / Initialize
import java.util.concurrent.Semaphore;
class Buffer
private int data;
private Semaphore mutex;
private Semaphore empty;
private Semaphore full;
public Buffer
Initialize semaphores
mutex new Semaphore; Binary semaphore for mutual exclusion
empty new Semaphore; Semaphore to track empty slots in buffer
full new Semaphore; Semaphore to track filled slots in buffer
public void produceint newData throws InterruptedException
empty.acquire; Wait for an empty slot
mutex.acquire; Acquire mutex for critical section
Add data to buffer
data newData;
System.out.printlnProduced: newData;
mutex.release; Release mutex
full.release; Signal that buffer is full
public int consume throws InterruptedException
full.acquire; Wait for a full slot
mutex.acquire; Acquire mutex for critical section
Consume data from buffer
int consumedData data;
System.out.printlnConsumed: consumedData;
mutex.release; Release mutex
empty.release; Signal that buffer is empty
return consumedData;
class Producer implements Runnable
private Buffer buffer;
public ProducerBuffer buffer
this.buffer buffer;
public void run
try
for int i ; i ; i
buffer.producei;
Thread.sleep; Simulate production time
catch InterruptedException e
eprintStackTrace;
class Consumer implements Runnable
private Buffer buffer;
public ConsumerBuffer buffer
this.buffer buffer;
public void run
try
for int i ; i ; i
buffer.consume;
Thread.sleep; Simulate consumption time
catch InterruptedException e
eprintStackTrace;
public class Main
public static void mainString args
Buffer buffer new Buffer;
Thread producerThread new Threadnew Producerbuffer;
Thread consumerThread new Threadnew Consumerbuffer;
producerThread.start;
consumerThread.start;
can you add override to this code and add rice condition if not there
Step by Step Solution
There are 3 Steps involved in it
Step: 1
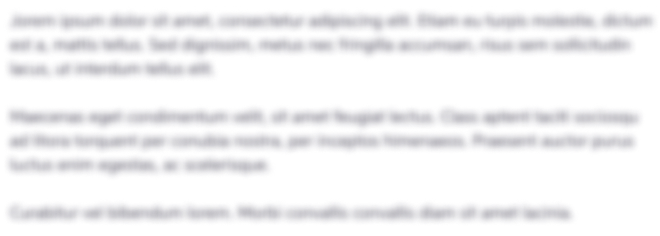
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started