Answered step by step
Verified Expert Solution
Question
1 Approved Answer
# import necessary packages # reading / writing image files from skimage import io from skimage import color # displaying images and plots import matplotlib
# import necessary packages
# readingwriting image files
from skimage import io
from skimage import color
# displaying images and plots
import matplotlib as mpl
import matplotlib.pyplot as plt
# array operations
import numpy as np
# mathematical calculations
import math
# data frame operations
import pandas as pd
# STEP Quantize the image with so that there are only intensity levels
# ADD YOUR CODE HERE
# display the quantized image
def myimgLuminanceimgRGB:
# make sure it is a color image
dimimg imgRGB.shape
assert dimimg
# get the luminance data
if dimimg :
imgLum color.rgbgrayimgRGB
else:
# ignore the alpha channel
imgLum color.rgbgrayimgRGB:::
imgLum nproundimgLum
imgLum npminimumimgLum
imgLum npmaximumimgLum
imgLum imgLum.astypeuint
return imgLum
def myHistogramimg nBit:
nLevel nBit
valMax
for i in rangenBit,:
valMax i
imgQuan img & valMax
hist npzerosnLevel dtypeint
valMin nBit
for n in rangenLevel:
histn npcountnonzeroimgQuannvalMin
return hist, imgQuan, nLevel
if imgRGB.ndim :
imgLum myimgLuminanceimgRGB
else:
imgLum imgRGB
nBit
histLum, imgLumQuan, numLevel myHistogramimgLum nBit
print
printLevel: nLevel
print
pltfigurefigsize
pltsubplot
plttitleQuantize Image'
pltimshowimgLumQuan cmap'gray'
pltaxisoff
pltshow
pltclose
# STEP Define a vector source by combining two consecutive pixels
# and calculate the probabilities of these vector symbols
# ADD YOUR CODE HERE
# notes:
# since each pixel has intensity levels, there will be x symbols in the vector source.
# the symbols for the vector source could be represented as aa ab ac hf fg hh
# there are a total of sample vectors in the input image to calculate the histogram
# calculate the histogram of the vector symbols
# obtain the pdf by normalizing the histogram
# plot the pdf of this vector source
Step by Step Solution
There are 3 Steps involved in it
Step: 1
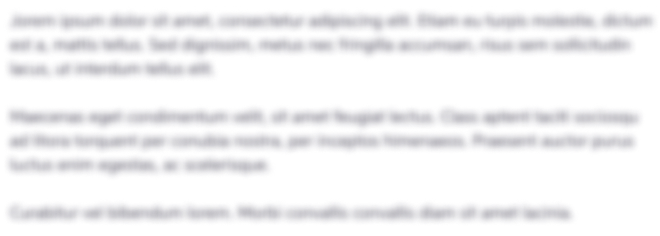
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started