Question
import org.junit.Assert; import static org.junit.Assert.*; import org.junit.Before; import org.junit.Test; import java.util.Random; public class UserAccountTest { UserAccount acct1; String name1= Horst; double bal1 =250.00; Random rand;
import org.junit.Assert; import static org.junit.Assert.*; import org.junit.Before; import org.junit.Test; import java.util.Random;
public class UserAccountTest { UserAccount acct1; String name1= "Horst"; double bal1 =250.00; Random rand;
/** Fixture initialization (common initialization * for all tests). **/ @Before public void setUp() { acct1 = new UserAccount(name1, bal1); rand = new Random(); }
/** Test the UserAccount constructor. **/ @Test public void createAccountNameTest() { String name = acct1.getUserName(); assertEquals("Test 1: user name set in constructor.", name1, name); } @Test public void createAccountBalanceTest() { double bal = acct1.getBalance(); assertEquals("Test 2: initial balance set in constructor.", bal1, bal, 0.001); } @Test public void createAccountBooksOrderedTest() { int books = acct1.getNumBooksOrdered(); assertEquals("Test 3: booksOrdered initialized correctly.", 0, books); } @Test public void createAccountBooksOrderedTitleTest() { String title = acct1.getCurrentBookOrdered(); assertEquals("Test 4: current book ordered title initialized correctly.", "none", title); } @Test public void createAccountBooksOrderedPriceTest() { double price = acct1.getCurrentBookPrice(); assertEquals("Test 5: current book ordered price initialized correctly.", 0.0, price, 0.001); } /* Test addToBalance */ @Test public void addToBalanceTest() { double amountToAdd = 3.45; acct1.addToBalance(amountToAdd); double bal = acct1.getBalance(); assertEquals("Test 6: test addToBalance method.", (bal1+amountToAdd), bal, 0.001); }
/* Test orderBook */ @Test public void orderBookTitleTest() { String orderTitle = "The Lean Startup"; double orderPrice = 33.98; acct1.orderBook(orderTitle, orderPrice); String title = acct1.getCurrentBookOrdered(); assertEquals("Test 7: current book ordered title set correctly after order.", orderTitle, title); } @Test public void orderBookPriceTest() { String orderTitle = "The Lean Startup"; double orderPrice = 33.98; acct1.orderBook(orderTitle, orderPrice); double price = acct1.getCurrentBookPrice(); assertEquals("Test 8: current book ordered price set correctly after order.", orderPrice, price, 0.001); } @Test public void orderBookBalanceTest() { String orderTitle = "The Lean Startup"; double orderPrice = 33.98; acct1.orderBook(orderTitle, orderPrice); double bal = acct1.getBalance(); assertEquals("Test 9: current balance correctly updated after order.", (bal1-orderPrice), bal, 0.001); } @Test public void orderBookNumBooksTest() { String orderTitle = "The Lean Startup"; double orderPrice = 33.98; acct1.orderBook(orderTitle, orderPrice); int num = acct1.getNumBooksOrdered(); assertEquals("Test 10: number of books ordered correctly updated after order.", 1, num); } /* Test addToBalance rand */ @Test public void addToBalanceRandomTestGS() { double amountToAdd = getRandAmt(100); acct1.addToBalance(amountToAdd); double bal = acct1.getBalance(); assertEquals("Test 11: test addToBalance method.", (bal1+amountToAdd), bal, 0.001); } /* Test constructor with random values */ @Test public void createAccountRandNameTestGS() { String randName = getRandName(); UserAccount acct2 = new UserAccount(randName, 50.00); String name = acct2.getUserName(); assertEquals("Test 12: user name set in constructor.", randName, name); } @Test public void createAccountRandBalanceTestGS() { String randName = getRandName(); double randBal = getRandAmt(100); UserAccount acct2 = new UserAccount(randName, randBal); double bal = acct2.getBalance(); assertEquals("Test 13: initial balance set in constructor.", randBal, bal, 0.001); } /* Test orderBook two books */ @Test public void orderBookTitleTestGS() { acct1.orderBook("The Lean Startup", 33.98); String orderTitle = "I, Robot"; double orderPrice = 22.68; acct1.orderBook(orderTitle, orderPrice); String title = acct1.getCurrentBookOrdered(); assertEquals("Test 14: current book ordered title set correctly after another order.", orderTitle, title); } @Test public void orderBookPriceTestGS() { acct1.orderBook("The Lean Startup", 33.98); String orderTitle = "I, Robot"; double orderPrice = 22.68; acct1.orderBook(orderTitle, orderPrice); double price = acct1.getCurrentBookPrice(); assertEquals("Test 15: current book ordered price set correctly after another order.", orderPrice, price, 0.001); } @Test public void orderBookBalanceTestGS() { acct1.orderBook("The Lean Startup", 33.98); String orderTitle = "I, Robot"; double orderPrice = 22.68; acct1.orderBook(orderTitle, orderPrice); double bal = acct1.getBalance(); assertEquals("Test 16: current balance correctly updated after another order.", (bal1-(orderPrice+33.98)), bal, 0.001); } @Test public void orderBookNumBooksTestGS() { acct1.orderBook("The Lean Startup", 33.98); String orderTitle = "I, Robot"; double orderPrice = 22.68; acct1.orderBook(orderTitle, orderPrice); int num = acct1.getNumBooksOrdered(); assertEquals("Test 17: number of books ordered correctly updated after another order.", 2, num); } /* Test random number of book orders */ @Test public void createAccountRandBooksOrderedTestGS() { UserAccount acct = new UserAccount("", 2000.00); int numOrders = getRandInt(3, 10); for(int i=0;i //helpers private double getRandAmt(int scale){ return Math.floor((rand.nextDouble()*scale) * 100) / 100; } private String getRandName(){ String[] names = {"Ali", "Mikayla","Helia","Pengshan","Neena","Wentzle"}; int index = rand.nextInt(names.length); return names[index]; } private String getRandtitle(){ String[] names = {"Hear of Darkness", "Holes","Catch 22","Gingerman","2001: A Space Odyssey","Happy Cooking"}; int index = rand.nextInt(names.length); return names[index]; } private int getRandInt(int min, int upper){ int res = rand.nextInt(upper); if(res /* This class models an Amazone user's account. A user account has these attributes: user name- the user's name. balance- the amount of money for the account. number of books ordered- a count of how many books were ordered. current book ordered- the title of the book the user has ordered. current book price- the price of the book the user has ordered. The class keeps track of the current book ordered as well as its price. It also keep a tally of the total number of books ordered. The user can add to their balance, order a book, and see their balance, the number of books they have ordered, and what their current order is. Note: each new book order overwrites the current book title and price. Each order adds to the tally of books ordered and deducts the order price from the user's balance. The balance can go negative. Amazone is generous with credit:-) */ public class UserAccount { /* Declare the variables you will need here: */ /* Constructor that initializes this account with a user name and an initial balance that is passed in. It also initializes the number of books ordered to 0, the current book ordered to "none", and the current order price to 0.0. */ public UserAccount(String userName, double initBal) { /* write this method body with your code */ } /* Returns the user name. */ public String getUserName() { /* re-write this method body with your code */ return null; } /* Returns the user's balance. */ public double getBalance() { /* re-write this method body with your code */ return -5.0; } /* Returns the number of books ordered. */ public int getNumBooksOrdered() { /* re-write this method body with your code */ return -5; } /* Returns the current book ordered. */ public String getCurrentBookOrdered() { /* re-write this method body with your code */ return null; } /* Returns the current book price. */ public double getCurrentBookPrice() { /* re-write this method body with your code */ return -5.0; } /* This method adds the amount passed in to the current balance. */ public void addToBalance(double amountToAdd) { /* write this method body with your code */ } /* This method sets the current book ordered to the book title, sets the current order price to the book price, deducts the book price from the balance, and increments the number of books ordered. */ public void orderBook(String bookTitle, double bookPrice) { /* write this method body with your code */ } } ------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------------- import java.util.Scanner; /* This class runs a console interface between a user and * an Amazone account. */ public class AmazoneMain { public static void main(String[] args){ System.out.println("MyAmazone Account"); /* Declare and initialize any variables you need here. At * You will need at least a Scanner and a UserAccount variable. */ /* Begin the while loop. */ //print the menu // if user eneters N // if user eneters A // if user eneters O // if user eneters S // if user eneters X // if none of the above were chosen, add an else and print "Unrecognized input." /* The end of the while loop. */ //Print the message "Bye for now." and close the Scanner object. } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
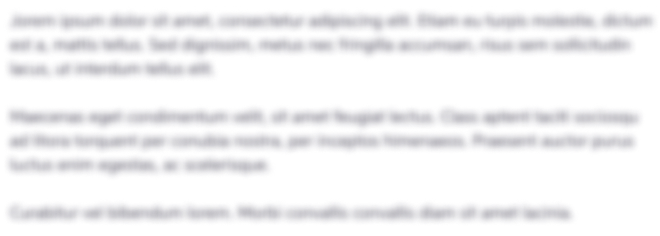
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started