Question
import static org.junit.jupiter.api.Assertions.assertArrayEquals; import static org.junit.jupiter.api.Assertions.assertEquals; import static org.junit.jupiter.api.Assertions.assertFalse; import static org.junit.jupiter.api.Assertions.assertTrue; import static org.junit.jupiter.api.Assertions.fail; import org.junit.jupiter.api.Test; /** * JUnit tests for the class Java1Review.
import static org.junit.jupiter.api.Assertions.assertArrayEquals; import static org.junit.jupiter.api.Assertions.assertEquals; import static org.junit.jupiter.api.Assertions.assertFalse; import static org.junit.jupiter.api.Assertions.assertTrue; import static org.junit.jupiter.api.Assertions.fail;
import org.junit.jupiter.api.Test;
/** * JUnit tests for the class Java1Review. * * Each method in this class tests a different method in Java1Review. Study the * tests to determine how to write the Java1Review methods. * * If you decide to write your own tests, please do so in the main method of * Java1Review. Do not modify this file. * * To run the JUnit tests in Eclipse, right-click JUnitTests.java in the * Package Explorer and select "Run As" > "JUnit Test" from the context menu. * (If a pop-up window appears with a notification about errors, click the * "Proceed" button.) A JUnit tab will open next to the Package Explorer that * shows a list of the tests. If a test is shown with a green check mark, this * indicates that the corresponding Java1Review method passed. */ class JUnitTests {
@Test void testFloatingPointDivision() { // JUnit tests use assertion methods to check the output of code. This // test uses assertEquals, which compares its first two arguments. If // the arguments are equal, the assertion passes; otherwise, the // assertion fails. To pass a JUnit test, every assertion in the method // must pass. assertEquals(4.0 / 4.0, Java1Review.divide(4.0, 4.0)); assertEquals(4.0 / 3.0, Java1Review.divide(4.0, 3.0)); assertEquals(4.0 / 2.0, Java1Review.divide(4.0, 2.0)); assertEquals(4.0 / 1.0, Java1Review.divide(4.0, 1.0)); assertEquals(Double.POSITIVE_INFINITY, Java1Review.divide(4.0, 0.0)); }
@Test void testIntegerDivision() { // In Java, different methods can have the same name. This is called // "method overloading." In order for the compiler to tell them apart, // the methods must have different parameter lists. assertEquals(1, Java1Review.divide(4, 4)); assertEquals(1, Java1Review.divide(4, 3)); assertEquals(2, Java1Review.divide(4, 2)); assertEquals(4, Java1Review.divide(4, 1));
// Unlike floating-point division, dividing by integer 0 causes an // arithmetic exception. Don't worry about the try-catch syntax right // now. We'll cover it later in the semester. try { Java1Review.divide(4, 0); fail(); // This method will cause the test to fail. } catch (ArithmeticException exception) { // If Java1Review.divide throws an arithmetic exception, the // program immediately leaves the try block (skipping the fail // method) and enters the catch block. } }
@Test void testIsDivisibleBy7() { // This test uses the assertion methods assertTrue and assertFalse. // These methods work just like assertEquals, but the second argument // is a fixed boolean value. For instance, the first assertion below // could be written like this: // assertEquals(Java1Review.isDivisibleBy7(0), true); assertTrue(Java1Review.isDivisibleBy7(0)); assertFalse(Java1Review.isDivisibleBy7(1)); assertTrue(Java1Review.isDivisibleBy7(7)); assertFalse(Java1Review.isDivisibleBy7(13)); assertTrue(Java1Review.isDivisibleBy7(-14)); }
@Test void testMain() { // In Java, even the main method can be overloaded, although this is // likely to confuse anyone reading your code. assertEquals("Overloaded main method was passed \"Hi!\".", Java1Review.main("Hi!")); assertEquals("Overloaded main method was passed \"I heart Java\".", Java1Review.main("I heart Java")); assertEquals("Overloaded main method was passed \"1337 h4x0r\".", Java1Review.main("1337 h4x0r")); }
@Test void testFindMin() { assertEquals(1, Java1Review.findMin(1, 2, 3)); assertEquals(1, Java1Review.findMin(3, 1, 2)); assertEquals(1, Java1Review.findMin(2, 3, 1)); assertEquals(-7, Java1Review.findMin(-7, 42, 18)); assertEquals(-7, Java1Review.findMin(42, 18, -7)); assertEquals(-7, Java1Review.findMin(18, -7, 42)); }
@Test void testFindMinElement() { int[] array = {1, 2, 3}; assertEquals(1, Java1Review.findMin(array));
array = new int[] {3, 2, 1}; assertEquals(1, Java1Review.findMin(array));
array = new int[] {7}; assertEquals(7, Java1Review.findMin(array));
array = new int[] {2, -1, 5, 1, -3, 2, 4}; assertEquals(-3, Java1Review.findMin(array));
array = new int[] {1, 1, 2, 3, 5, 8, 13}; assertEquals(1, Java1Review.findMin(array)); }
@Test void testAverage() { int[] array = {1, 2, 3}; assertEquals(6.0 / 3.0, Java1Review.average(array));
array = new int[] {3, 2, 1}; assertEquals(6.0 / 3.0, Java1Review.average(array));
array = new int[] {7}; assertEquals(7.0, Java1Review.average(array));
array = new int[] {2, -1, 5, 1, -3, 2, 4}; assertEquals(10.0 / 7.0, Java1Review.average(array));
array = new int[] {1, 1, 2, 3, 5, 8, 13}; assertEquals(33.0 / 7.0, Java1Review.average(array)); }
@Test void testToLowerCase() { // This test uses the assertion method assertArrayEquals. This method // works like assertEquals, but it takes two arrays and compares them // element by element. The assertion passes only if the corresponding // elements are equal. String[] strings = {"ABC"}; Java1Review.toLowerCase(strings); assertArrayEquals(new String[] {"abc"}, strings);
strings = new String[] {"I ", "heart ", "Java"}; Java1Review.toLowerCase(strings); assertArrayEquals(new String[] {"i ", "heart ", "java"}, strings);
strings = new String[] {"E. E.", "Cummings"}; Java1Review.toLowerCase(strings); assertArrayEquals(new String[] {"e. e.", "cummings"}, strings); }
@Test void testToLowerCaseCopy() { String[] strings = {"ABC"}; assertArrayEquals(new String[] {"abc"}, Java1Review.toLowerCaseCopy(strings)); // Java1Review.toLowerCaseCopy should leave the given array unchanged. assertArrayEquals(new String[] {"ABC"}, strings);
strings = new String[] {"I ", "heart ", "Java"}; assertArrayEquals(new String[] {"i ", "heart ", "java"}, Java1Review.toLowerCaseCopy(strings)); assertArrayEquals(new String[] {"I ", "heart ", "Java"}, strings);
strings = new String[] {"E. E.", "Cummings"}; assertArrayEquals(new String[] {"e. e.", "cummings"}, Java1Review.toLowerCaseCopy(strings)); assertArrayEquals(new String[] {"E. E.", "Cummings"}, strings); }
@Test void testRemoveDuplicates() { // Java1Review.removeDuplicates looks for integers that appear more // than once in a given array. If an integer has duplicates, the method // replaces each appearance of the integer with 0. int[] array = {451}; Java1Review.removeDuplicates(array); assertArrayEquals(new int[] {451}, array);
array = new int[] {451, 451}; Java1Review.removeDuplicates(array); assertArrayEquals(new int[] {0, 0}, array);
array = new int[] {451, 451, 451}; Java1Review.removeDuplicates(array); assertArrayEquals(new int[] {0, 0, 0}, array);
array = new int[] {451, 451, 42, 451}; Java1Review.removeDuplicates(array); assertArrayEquals(new int[] {0, 0, 42, 0}, array);
array = new int[] {451, 451, 42, 451, 101}; Java1Review.removeDuplicates(array); assertArrayEquals(new int[] {0, 0, 42, 0, 101}, array);
array = new int[] {451, 101, 451, 42, 451, 101}; Java1Review.removeDuplicates(array); assertArrayEquals(new int[] {0, 0, 0, 42, 0, 0}, array);
array = new int[] {451, 101, 451, 42, 451, 101, -1}; Java1Review.removeDuplicates(array); assertArrayEquals(new int[] {0, 0, 0, 42, 0, 0, -1}, array);
array = new int[] {38, 451, 101, 451, 42, 451, 101, -1}; Java1Review.removeDuplicates(array); assertArrayEquals(new int[] {38, 0, 0, 0, 42, 0, 0, -1}, array); } } **********************************
solution should be in this class:
/** * Implement each of the 10 methods tested in JUnitTests.java. Study the tests * to determine how the methods should work. */ public class Java1Review {
public static void main(String[] args) { // If you want to write your own tests, do so here. (Do not modify // JUnitTests.java.) To run this method in Eclipse, right-click // Java1Review.java in the Package Explorer and select "Run As" > // "Java Application" from the context menu. }
}
Run the Unit Tests Take a look at the class JUnitTests. This is a JUnit test class. Notice that each method is prefixed with the annotation @Test. Right-click the file JUnit Tests.java in the Package Explorer and select "Run As" > "JUnit Test" from the context menu. You will receive a warning that errors exist in the project, but click the "Proceed" button anyway. A new tab will open next to the Package Explorer named "JUnit." The tab includes a list of all the methods with the @Test annotation. Each of these methods is a test, and they're all failing! The goal of this assignment is to add code to the class Java 1 Review so that all of the tests pass. (Don't change the code in JUnitTests.) You'll know you're making progress when some of those red X's turn into green check marks. Write the Java1Review Class Let's take a closer look at the first test in JUnit Tests, which I'll duplicate below. @Test void testFloatingPointDivision () { assertEquals(4.0 / 4.0, JavalReview.divide (4.0, 4.0)); assertEquals(4.0 / 3.0, JavalReview.divide (4.0, 3.0)); assertEquals(4.0 / 2.0, JavalReview.divide (4.0, 2.0)); assertEquals(4.0 / 1.0, JavalReview.divide (4.0, 1.0)); assertEquals(Double. POSITIVE_INFINITY, JavalReview.divide (4.0, 0.0)); } The test, which is named "testFloating Point Division," calls the assertEquals method five times. (If you look at the other tests, you'll see that they also call methods with names that begin with "assert.") This is a special JUnit method that defines the test criteria. To pass the test, assertEquals must be given two equal inputs every time it is called. If assertEquals is called anywhere in testFloatingPointDivision with unequal inputs, the test fails. Now notice that the second input to assertEquals is always a call to a method named "divide" in the class Java 1 Review. This implies that testFloatingPointDivision is testing the Java 1 Review divide method. Each time assertEquals is called, it checks the output of divide. By looking at the inputs to divide and the first input to assertEquals , we can see that divide should divide its first argument by its second and return the result. Each method in JUnit Tests tests a different method in Java1Review. By studying the tests, you can figure out how each Java 1 Review method should work. Your job is to write the Java1 Review methods so that they pass the tests. This approach to writing software, where the tests are written before the code, is known as "test-driven development." You will learn more about it later in the semester. Getting Started The Java1 Review class included in your GitHub repo is entirely empty other than the class declaration and the main method. This is why the Java 1 Review methods are underlined in red in JUnitTests. (JUnitTests can't find the methods in Java 1 Review because they don't exist yet.) A good way to start this assignment is to add method stubs to Java 1 Review. A method stub consists of a method declaration and an arbitrary return value. For instance, if you know a method returns an integer, the body of the stub could be return @; . For each test in JUnitTests, try to write a stub for the Java1 Review method being tested. Think carefully about the method declarations. In particular, ask yourself these questions: What is the data type of each input? What is the return type? Should the method be static? Should the method be public or private? You'll know a stub is correct if the method is no longer underlined in JUnitTests. After you've written all the stubs, the project will run without warning you about errors. Then you can focus on passing the tests. This assignment is intended to be a review of concepts from your previous programming class, so consider looking back at your old code for help. If you run into trouble, be sure to ask your TA some questionsStep by Step Solution
There are 3 Steps involved in it
Step: 1
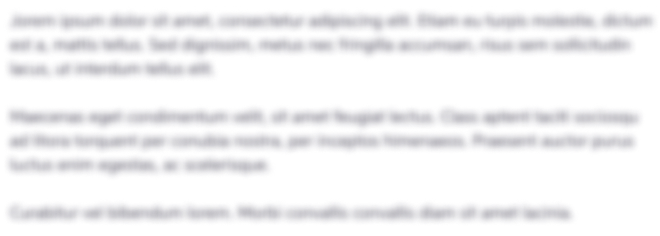
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started