Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In a guessing game, you think of something and I have to guess what it is by asking you questions that have a yes or
In a guessing game, you think of something and I have to guess what it is by asking you questions that have a yes or no answer. Write a program that asks the questions for me
This program uses a binary decision tree that grows as the game progresses. Instead of creating the tree before it is used, the program acquires facts from the user and adds them to the decision tree. Thus, the program learns by playing the game and becomes more proficient over time.
To simplify the problem, lets restrict your choice of things. For example, suppose that you think of a country. The program could begin with the simple threenode tree:
Is it in North America Yes USA
No
Brazil
With this tree, the program asks the question in the root and makes one of two guesses, depending on the answer to the question. Here is one possible exchange between the program and the user user replies are bold:
Is it in North America?
Yes Bold
My guess is USA Am I right?
yes bold
I win.
Play again?
The program has guessed correctly; the tree remains unchanged.
Now, suppose the user is thinking of something else. The exchange might go like this:
Is it in North America?
no bold
My guess is Brazil. Am I right?
no bold
I give up; what are you thinking of
England bold
Give me a question whose answer is yes for England and no for Brazil.
Is it in Europe? bold
Play again?
We replace the contents of the leaf that contained the wrong answerBrazil in this casewith the new question provided by the user. We then give the leaf two children. One child contains the guess that was in the former leaf Brazil and the other contains the users answer England as a new guess. The program now can distinguish between Brazil and England.
Finish the code in the file GuessingGame.java and submit in Canvas. You may keep the same file name. You do not need to submit the Package folders they are for your use when developing your program.
Code:
import TreePackage.;
import StackAndQueuePackage.;
import java.util.Scanner;
public class GuessingGame
public static void mainString args
String response;
DecisionTree gameTree new DecisionTreeIs it in North America?", "Brazil", USA;
do
System.out.printlnThink of a country and I will guess it;
gameTree playgameTree;
System.out.printPlay again? ;
response getUserResponse;
while responsetoLowerCaseequalsyes;
System. out printlnBye;
end main
public static String getUserResponse
TODO
return response;
end getUserResponse
public static boolean isUserResponseYes
String answer getUserResponse;
if answertoLowerCaseequalsyes
return true;
else
return false;
end isUserResponseYes
public static DecisionTree playDecisionTree gameTree
TODO Initialize current node to root
TODO while loop answer has not been reached
Ask current question
System.out printlngameTreegetCurrentData;
if isUserResponseYes
gameTree. advanceToYes;
else
TODO
end while
assert TODO Assertion: Leaf is reached
Make guess
System.out.printlnMy guess is gameTree.getCurrentData Am I right?";
TODO if user responds 'yes'
System.out.printlnI win.";
else
learn gameTree ;
return gametree;
end play
Responds to the user when this program makes a wrong guess and
extends the decision tree so that this guess is not made again.
public static void learnDecisionTree gameTree
System.out.printlnI give up; what are you thinking of;
TODO set correct answer string
TODO set current answer string
System.out.printlnGive me a question whose answer is yes for correctAnswer but no for currentAnswer;
String newQuestion getUserResponse;
TODO create a new question with current data
TODO set responses based on current answer and correct answer
end learn
end GuessingGame
Step by Step Solution
There are 3 Steps involved in it
Step: 1
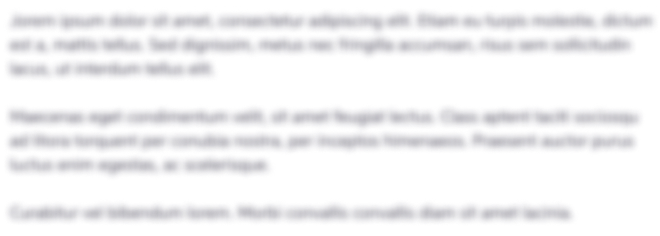
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started