Question
in c++ i have some difficulties with this program class declarement i wanted to see if anyone can help me with it. You will write
in c++ i have some difficulties with this program class declarement i wanted to see if anyone can help me with it. You will write a movie rental program that manages the movie inventory and rentals of those movies. This program will provide a simple command-line interface that allows users to interact with the application and perform actions such as creating a new movie in inventory, removing a movie from the inventory, renting a movie, and returning movie to the inventory.
The first thing the user sees is a brief welcome message and a small menu describing commands that the user can make. For example:
Welcome to Movie Rental Kiosk!
----------
am: Add Movie
dm: Discontinue Movie
rm: Rent Movie
rr: Return Rental
p: Print Movie Inventory
q: Quit Program
----------
Enter Command:
Since I am expecting us to use standard arrays and we dont have as much flexibility with the size so you will limit the maximum number of unique movies in inventory to 20 and the number of available movies to be rented to 10.
- am: Add Movie
- Adds a movie to be managed by the program
- The program will prompt the user for the movie code (i.e. HPDH2), and the movie name (i.e. Harry Potter and the Deathly Hollows Part 2).
- If the program already has the maximum number of movies (i.e. 20), then a short error is reported to the user and the menu is displayed again.
- There are no restrictions on what the valid strings for a movie code or name could be with the exception of being empty
- If either the movie code or movie name are empty a short error is reported to the user and the menu is re-displayed
- There is no specific order to maintain for the list of movies in inventory.
- The movie codes must be unique.
- If a duplicate is being added, then a brief error is reported and the menu is displayed again.
- dm: Discontinue Movie
- Removes a movie from the inventory of movies
- The program will prompt the user for the movie code. The program will then delete the movie with the movie code from memory.
- If the movie code does not match any in the inventory (note movie codes are case insensitive), then a brief error is reported and the main menu is re-displayed.
- If the inventory list is empty, then a brief error is reported and the main menu is re-displayed
- If there are copies of the movie still out for rental, then a brief error is reported with the name(s) of the renter that is currently renting them and the main menu is re-displayed again.
- rm: Rent Movie
- Adds a renter to a specific movie in the system
- The program will prompt the user for the movie code that the renter will be renting, the renter ID, and the first / last name of the renter.
- Similar to the Discontinue movie case, if the movie code does not match an existing movie code, then a brief error is reported and the main menu is re-displayed.
- There are no restrictions on what the first and last name strings can be except for empty ones. (I.e. the renter has to have at least 1 character for each first and last name)
- If either the first or last name are empty a short error is reported to the user and the main menu is re-displayed
- The renter ID must be a positive integer greater than 0 (your program can assume the renter will enter a integer, but not cannot assume it will be a positive integer).
- If the renters ID is negative a short error is reported to the user and the menu is displayed again.
- Renter IDs must be unique
- If a duplicate renter ID is found, then a brief error is reported
- If the movies are all rented (i.e. it contains10 renters), then a brief error is reported and the main menu is re-displayed again.
- Renters array should be sorted alphabetically by comparing first name first then last name second then ID number third.
- If the first and the last names are the same then you put the renter with the smaller ID number first.
- If the first name of a renter is a part of another name of another renter like (Geo and George) then the smaller first name goes first (In this case it's Geo) and same the applies for the last name in case of matching first name.
- rr: Return Rental
- Returns a rented movie from a specific movie in the system.
- The program will prompt the renter for the movie code that the renter will be returning and the renters ID.
- If the movie code does not match an existing movie code, then a brief error is reported and the main menu is re-displayed again.
- If the renter ID does not exist in the movies list of renters, a brief error is reported and the main menu is re-displayed again.
- If the movie has zero renters, then a brief error is reported and the main menu is re-displayed
- p: Print Inventory
- Prints the inventory of movies, including all of the movies and renters renting each movie.
- This includes the movie code, the movie name, the number of copies for each movie that are already rented and the list of renters including their renter ID, last name and first name.
- To print the Movie information you should overload the << operator.
- Prints the inventory of movies, including all of the movies and renters renting each movie.
- q: Quit Program
- Quits the program when this option is entered
Exceptions
Since there are several scenarios where a brief error is reported, I want you to practice Exception handling to fine-tune the error messages that are displayed to the user. The Exceptions you will need to create and use in your program are:
- DuplicateMovieException: Thrown when a movie already exists when trying to add a new movie.
- MovieLimitException: Thrown when a movie is trying to be added and the number of movies is at the at the max (I.e. 10).
- EmptyMovieInfoException: Thrown when the movie code and/or movie name is empty when trying to add one to the movie array.
- MovieNotFoundException: Thrown when a movie that does not exist.
- EmptyMovieListException: Thrown when trying to return a movie when the inventory list is empty.
- EmptyRenterListException: Thrown when trying to return a movie that does not have any copies currently being rented.
- RenterLimitException: Thrown when a renter is renting to a movie without any available copies.
- RenterNotFoundException: Thrown when trying to remove a renter for a movie that doesnt exist.
- DuplicateRenterException: Thrown when trying to add a renter to a movie and a duplicate renter ID exists.
- InvalidRenterIDException: Thrown when the user enters an invalid renter ID.
- EmptyRenterNameException: Thrown when the user enters an empty first and/or last name for the renter.
- RentedMovieException: Thrown when the user attempts to discontinue a movie that has copies currently rented out.
Organization
I will provide some requirements that you will need to follow:
- main.cpp
- Contains the main
- All it does is construct a MovieManager and calls run().
- MovieManager.cpp
- A class representing the highest layer of managing the movie inventory containing:
- An array of Movies
- Total number of current Movies
- Public Functions
- run()
- Runs the main loop of the program. This displays the menu, accepts user input, and handles each of the user commands.
- Handles the custom Exceptions and displays the messages to the user
- addMovie(Movie m)
- throws MovieLimitException, EmptyMovieInfoException, DuplicateMovieException
- Adds a movie to the array of movies
- discontinueMovie(String movieCode)
- throws MovieNotFoundException, EmptyMovieListException, RentedMovieException
- Removes a movie from the array of Movies
- rentMovie(String movieCode, Renter s)
- throws MovieNotFoundException
- Adds a renter to an already existing movie
- returnRental (int renterId, String movieCode)
- throws MovieNotFoundException
- Removes a renter from an already existing movie
- printInventory()
- Prints the information for all movies and their renters
- run()
- A class representing the highest layer of managing the movie inventory containing:
- Movies.cpp
- Class that represents a movie containing:
- A String representing the movie code.
- A String representing the movie name.
- An int representing the count of rented copies of the movie
- An array of Renters renting the movie.
- Public Functions
- rentMovie(Renters)
- throws RenterLimitException, DuplicateRenterException, InvalidRenterIDException, EmptyRenterNameException
- Adds a renter to the The renters array.
- returnRental(int renterId)
- throws EmptyRenterListException, RenterNotFoundException, InvalidRenterIDException
- Removes a renter from the movie based on their renters ID
- Any setters / getters you need to use in your program
- Overloaded << operator.
- rentMovie(Renters)
- Class that represents a movie containing:
- Renter.cpp
- Class representing a renter containing:
- An int representing the renter ID
- A String representing the renters first name.
- A String representing the renters last name.
- Public Methods
- Any setters / getters you need to use in your program
- Class representing a renter containing:
- MovieManagerUI.cpp
- Class representing the I/O between the program and user
- There really isnt a state to keep when using this object, so all of its methods can be static
- Public Functions
- void printMenu()
- Prints the menu options to the
- String getCommand()
- Gets the command from the console
- Loops until the user enters a valid command and returns the valid command.
- Any method to help obtain information from the user including:
- Getting the movie information when adding a new movie.
- Getting the movie code when adding a renter to a movie or trying to remove a movie
- Getting the renter information when adding a renter to a movie.
- Getting the renter ID when trying to remove a renter from a movie.
- void printMenu()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
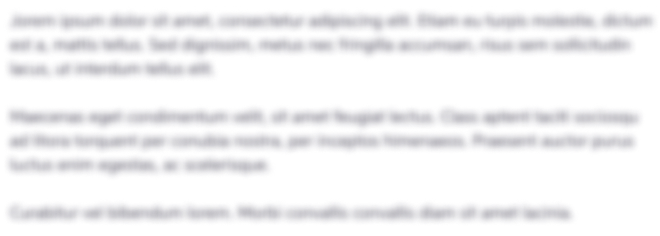
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started