Question
In C++ Implement template stack class that is capable of storing integer and string values. Write both header and implementation in stack.h. Private data fields:
In C++ Implement template stack class that is capable of storing integer and string values. Write both header and implementation in stack.h.
Private data fields:
data: An array with maximum size of 20. (Declare a constant in stack.h called MAX_SIZE and set it to 20.)
size: stores the current number of elements in the stack.
Public interface:
stack(): constructs an empty stack.
push(T val): inserts a new element (val) of type T (T could be integer or string) into the data. If the data array is full, this function should throw an overflow_error exception with error message "Called push on full stack.".
pop(): removes the last element from data. If the data array is empty, this function should throw an outofrange exception with error message "Called pop on empty stack.".
top(): returns the top element of stack (last inserted element). If stack is empty, this function should throw an underflow_error exception with error message "Called top on empty stack.".
empty(): returns true if the stack is empty otherwise it returns false.
main.cpp test harness / Use the given main.cpp file for testing your stack.
#include
#include
#include "stack.h"
#include
using namespace std;
int main()
{
cout << "Enter a test number(1-5): ";
int test;
cin >> test;
cout << endl;
//tests constructor, push, pop, top and empty
if (test == 1) {
try{
cout << " stack1 constructor called";
stack
if(stack1.empty()){
cout<<" stack1 is empty.";
}
else{
cout<<" stack1 is not empty";
}
cout << " push 10";
stack1.push( 10 );
cout << " push 20";
stack1.push( 20 );
cout << " push 30";
stack1.push( 30 );
cout << " stack1 top: ";
cout< cout << " pop"; stack1.pop(); cout << " stack1 top: "; cout< cout << " pop"; stack1.pop(); cout << " stack1 top: "; cout< cout << " pop"; stack1.pop(); if(stack1.empty()){ cout<<" stack1 is empty."; } else{ cout<<" stack1 is not empty"; } cout << endl; } catch(underflow_error & e){ cout< } catch(overflow_error & e){ cout< } catch(out_of_range & e){ cout< } } //tests top on empty stack if (test == 2) { try{ cout << " stack2 constructor called"; stack cout << " stack2 top: "; cout< cout << endl; } catch(underflow_error & e){ cout< } catch(overflow_error & e){ cout< } catch(out_of_range & e){ cout< } } //tests pop from an empty stack if (test == 3) { try{ cout << " stack3 constructor called"; stack cout<<" pop from empty stack "; stack3.pop(); cout << endl; } catch(underflow_error & e){ cout< } catch(overflow_error & e){ cout< } catch(out_of_range & e){ cout< } } //tests push to a full stack if (test == 4) { try{ cout << " stack4 constructor called"; stack cout << " push 20 elements"; for(int i = 1; i <=20; ++i){ stack4.push(i); } cout<<" stack4 top: "; cout< cout<<" push 21 "; stack4.push(21); cout << endl; } catch(underflow_error & e){ cout< } catch(overflow_error & e){ cout< } catch(out_of_range & e){ cout< } } //tests stack of strings if (test == 5) { try{ cout << " stack5 constructor called"; stack cout << " push A"; stack5.push("A"); cout << " push B"; stack5.push("B"); cout << " push C"; stack5.push("C"); cout << " stack5 top: "; cout< cout << " pop"; stack5.pop(); cout << " stack5 top: "; cout< cout << " pop"; stack5.pop(); cout << " stack5 top: "; cout< cout << " pop"; stack5.pop(); if(stack5.empty()){ cout<<" stack5 is empty."; } else{ cout<<" stack5 is not empty"; } cout << " stack5 top: "; stack5.top(); cout << endl; } catch(underflow_error & e){ cout< } catch(overflow_error & e){ cout< } catch(out_of_range & e){ cout< } } return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
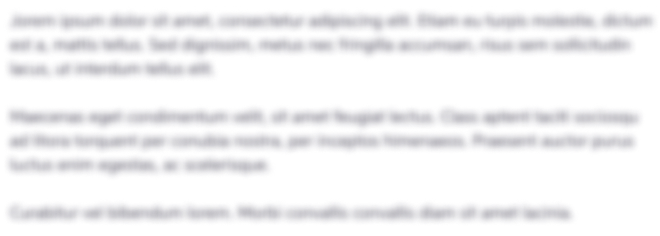
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started