Question
IN C++ #include #include #include using namespace std; int main() { string line; string text; int number; double decimal; PART I Declare an input string
IN C++ #include#include #include using namespace std; int main() { string line; string text; int number; double decimal;
PART I
Declare an input string stream named sin.
There are a few ways to declare a string stream:
istringstream sin; // This is an empty string stream you can directly put a string into
istringstream sin("some string"); // This puts the string "some string" into the string stream
istringstream sin(line); // This puts the contents of the string text into the string stream
PART II
Using getline() and a while loop, read in line by line from cin and store into the string line already declared for you. You will be done when you type your opening brace '{'. Try writing both forms of getline() described below.
getline() can take two parameters getline(cin, line)
getline() can take three parameters getline(cin, line, ' ')
The second form of getline() allows you to specify when getline() will stop reading. A line is ended by (the newline character), so this is the default, however say you are reading from a CSV (comma-separated values) file where the fields look like: some,text,goes,here. You can read this in using getline(cin, line, ',') so that you can grab some, text, goes, here.
getline() returns either true or false. If getline() is able to get a line and put it in the string, it returns true, otherwise it returns false
CAUTION: Even though getline() may return true, you still need to check to make sure you have what you expect put into the string. getline() returns true as long as ANYTHING gets put into the string
PART III
Now put the value of the string line into the string stream sin
Remember, a string stream can only have one string at a time, so you can only do .str() once unless you clear the string inside of the string stream. Use .clear() to first clear the string, then use .str() to give it the string line
PART IV
As stated above, getline() grabs whatever it can and puts it into the string. You need to check to make sure you get all of the fields you expect to get. You need to do this with the string stream
The string stream is exactly like fin or cin. If you use the extractors '>>', it will return true if it is able to put good values in every variable you extract, or return false if it cannot.
For example: if (sin >> a >> b >> c) will only return true if a, b, and c get good and correct data in ALL three a, b, and c. If even one of them fails, the entire statement returns false.
You can use this to make sure you get good data
In the box below, extract number, decimal, and text in that order using the variables already declared for you with the same names
If you cannot get good data in all three, output "Bad Data: XXXXXX " and replace XXXXXX with the actual line you got from getline()
else { cout << setprecision(2) << fixed; cout << "Got: " << number << " " << decimal << " " << text << endl; } } // End of while() loop return 0; } // end of int main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
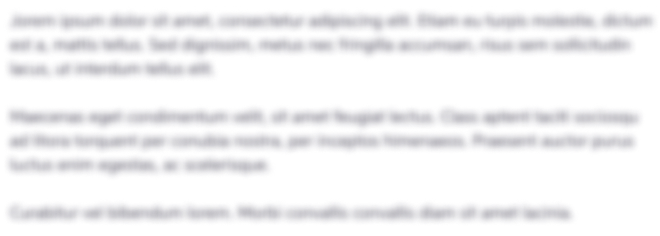
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started