Question
in C++ Linked List with a Student class There are four files: Student.h StudentList.h StudentList.cpp main.cpp These files contain a program that displays the name
in C++ Linked List with a Student class
There are four files:
- Student.h
- StudentList.h
- StudentList.cpp
- main.cpp
These files contain a program that displays the name and GPA for students with n-letter names. Example:
Enter n: 4 List of students with 4 letter names 3.2 Andy 2.5 Paul 3.9 Tina
Note: This program demonstrates the insertNode, deleteNode, getCount(), and displayList member functions. It builds and displays a sorted list. The list is sorted by name.
The information of the students is store in an array type Student s[7], but now it is required to read a file in order to get that information.
Mission: This program is already working, but the mission is to create a function that reads the file students.txt for this program to work as is working right now.
********************students.txt*********************
2.5 Paul 3.9 Katie 3.6 Bill 2.7 Ann 3.9 Tina 3.2 Andy
**********************main.cpp****************************
#include
//Create te prototype function here
int main() { // Define a StudentList object. StudentList list;
//Replace for the function to read the file*****Apply the changes here. You have to comment this Student s[] = {{2.5, "Paul"}, {3.9, "Katie"}, {3.6, "Bill"}, {2.7, "Ann"}, {3.9, "Tina"}, {3.2, "Andy"}, {0, ""}}; //****************************** cout << "TESTING INSERT "; // Build List from array (for demonstration purposes only)
//Replace for the function to read the file*****Apply the changes here You have to comment this for (int i = 0; i < 6; i++) { cout << "\tInsert " << s[i].getGpa() << " " << s[i].getName() << endl; list.insertNode(s[i]); // Display the values in the list. // list.displayList(); // cout << "\t\tThis list has " << list.getCount() << " student[s] "; } //****** Call the function you will create the file here //****************************** cout << "TESTING DISPLAY "; list.displayList(); int n; // number of letters in a studen's name cout << "Enter n: "; cin >> n; cout << "List of students with " << n << " letter names" << endl;
list.displayListName(n); //****************************** cout << "TESTING DELETE "; string target[] = { "Andy", "Tina", "Katie", "Tim", ""}; // names to test deleteNode with for (int i = 0; target[i] != ""; i++) { if( list.deleteNode(target[i]) ) cout << target[i] << " - Deleted! "; else cout << target[i] << " - Not found "; } list.displayList(); cout << "\t\tThis list has " << list.getCount() << " student[s] "; return 0; }
********************Student.h********************
#ifndef STUDENT_H #define STUDENT_H
//using namespace std; //<==== This statement // in a header file of a complex project could create // namespace management problems for the entire project // (such as name collisions). // Do not write namespace using statements at the top level in a header file! #include
class Student { private: double gpa; string name; public: Student() {name = ""; gpa = -1;} // Constructor Student(double g, string n) {name = n; gpa = g;} // Overloaded Constructor // Setters and getters void setName(string n) {name = n;} void setGpa(double g) {gpa = g;} string getName() const {return name;} double getGpa() const {return gpa;} }; #endif
********************StudentList.h********************
#ifndef STUDENTLIST_H #define STUDENTLIST_H #include "Student.h" struct ListNode { Student stu; ListNode *next; };
class StudentList { private: ListNode *head; // List head pointer int count; // To keep track of the number of nodes in the list
public: StudentList(); // Constructor ~StudentList(); // Destructor // Linked list operations int getCount() const {return count;} void insertNode(Student dataIn); bool deleteNode(std::string target); void displayList() const; void displayListName(double n);
}; #endif
**********************StudentList.cpp****************************
#include
//************************************************** // Constructor // This function allocates and initializes a sentinel node // A sentinel (or dummy) node is an extra node added before the first data record. // This convention simplifies and accelerates some list-manipulation algorithms, // by making sure that all links can be safely dereferenced and that every list // (even one that contains no data elements) always has a "first" node. //************************************************** StudentList::StudentList() { head = new ListNode; // head points to the sentinel node head->next = NULL; count = 0; }
//************************************************** // displayList shows the value // stored in each node of the linked list // pointed to by head. //**************************************************
void StudentList::displayList() const { ListNode *pCur; // To move through the list.
// Position pCur: skip the head of the list. pCur = head->next;
// While pCur points to a node, traverse the list. while (pCur) { // Display the value in this node. cout << pCur->stu.getGpa() << " " << pCur->stu.getName() << endl;
// Move to the next node. pCur = pCur->next; } cout << endl; } //************************************************** // displayList shows the value //of the studetns with the names with the same lenght //thata the user types //************************************************** void StudentList::displayListName(double n) // New Fucntion I added to see just the students requeried for the user
{ ListNode *pCur; // To move through the list. // Position pCur: skip the head of the list. pCur = head->next; int counter = 0;
// While pCur points to a node, traverse the list. while (pCur) { // Display the value in this node if stu.gpa >= gpa if (pCur->stu.getName().length() == n) { cout << pCur->stu.getGpa() << " " << pCur->stu.getName() << endl; } else { counter = counter + 1; } // Move to the next node. pCur = pCur->next; } if (counter == 6){ cout << "None!"; } cout<< endl; }
//************************************************** // The insertNode function inserts a node with // stu copied to its value member. //************************************************** void StudentList::insertNode(Student dataIn) { /* Write your code here */ ListNode *newNode; // A new node ListNode *pCur; // To traverse the list ListNode *pPre; // The previous node // Allocate a new node and store dataIn there. newNode = new ListNode; newNode->stu = dataIn;
// Initialize pointers pPre = head; pCur = head->next; // Find location: skip all nodes whose name is less than dataIn's gpa while (pCur != NULL && pCur->stu.getName() < dataIn.getName()) { pPre = pCur; pCur = pCur->next; }
// Insert the new node between pPre and pCur pPre->next = newNode; newNode->next = pCur; // Update the counter count++; }
//************************************************** // The deleteNode function searches for a node // with num as its value. The node, if found, is // deleted from the list and from memory. //************************************************** bool StudentList::deleteNode(string target) { ListNode *pCur; // To traverse the list ListNode *pPre; // To point to the previous node bool deleted = false; /* Write your code here */ // Initialize pointers pPre = head; pCur = head->next;
// Find node containing the target: Skip all nodes whose name is less than the target while (pCur != NULL && pCur->stu.getName() < target) { pPre = pCur; pCur = pCur->next; } // If found, delte the node if (pCur != NULL && pCur->stu.getName() == target) { pPre->next = pCur->next; delete pCur; deleted = true; count--; } return deleted; }
//************************************************** // Destructor * // This function deletes every node in the list. * //************************************************** StudentList::~StudentList() { ListNode *pCur; // To traverse the list ListNode *pNext; // To point to the next node // Position nodePtr at the head of the list. pCur = head->next; // While pCur is not at the end of the list... while (pCur != NULL) { // Save a pointer to the next node. pNext = pCur->next; // Delete the current node. cout << "DEBUG - Destructor: Now deleting " << pCur->stu.getName() << endl; delete pCur; // Position pCur at the next node. pCur = pNext; } cout << "DEBUG - Destructor: Now deleting the sentinel node gpa = " << head->stu.getGpa() << endl; delete head; // delete the sentinel node }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
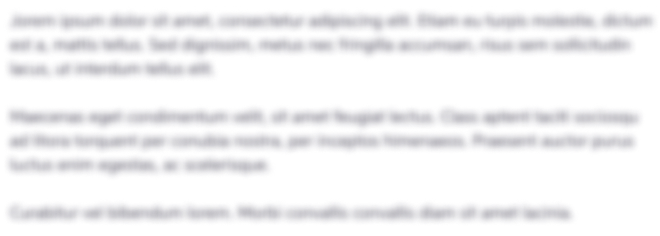
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started