Question
In C++ Objectives Implementing a class Using a header file Design a small inheritance tree Map your classes to the tree Tasks For this project
In C++
Objectives
Implementing a class
Using a header file
Design a small inheritance tree
Map your classes to the tree
Tasks
For this project you are going to create a class that represents a manufactured part on a bill of material (BOM). This part will have the following attributes (represented as a private variable):
identifier - The parts identifier as an alpha numeric string
drawing - The AutoCAD drawing file that represents the part
quantity - The number of parts that are required
In addition to the above attributes your class will provide the following methods.
Part(string id, string dwg, int qty) - Object constructor: Initializes member variables
getId() - returns the id of the part
void setId(string id) - sets the id of the part
string getDrawing() - returns this parts drawing file name
void setDrawing(string drawing) - sets this parts drawing name
int getQuantity() - returns the quantity of this part
void setQuantity(int qty) - sets the quantity of this part
string toString() - returns a string representation of the part
Your task is to design a class named Part you will declare the class in a file Part.h and implement the class in Part.cpp. The main function is already written for you below, do not modify the main function.
#include
SAMPLE OUTPUT
Id: 12-00001 drawing: 12-00001.dwg qty: 1 Id: 12-00001 drawing: 14-11111.dwg qty: 1 Id: my_new_id drawing: 14-11111.dwg qty: 1 Id: my_new_id drawing: 14-11111.dwg qty: 42 14-11111.dwg my_new_id 42
PART H
#ifndef PART_H #define PART_H #include using namespace std; class Part { private: string m_id; string m_drawing; int m_quantity; public: Part(string id, string dwg, int qty); string getId(); void setId(string id); string getDrawing(); void setDrawing(string drawing); int getQuantity(); void setQuantity(int qty); string toString(); }; #endif
Part cpp
// Part.cpp : Defines the entry point for the console application. // #include #include "Part.h" using namespace std; // // I will use my own 'main' file; therefore, you can modify // this file as you see fit. // int main(int argc, char **argv) { //Constructs a new part Part p("12-00001", "12-00001.dwg", 1); //Print the part out. cout << p.toString() << endl; //Test our set Drawing method p.setDrawing("14-11111.dwg"); cout << p.toString() << endl; //Test our set setId method p.setId("my_new_id"); cout << p.toString() << endl; //Test our set Quantity method p.setQuantity(42); cout << p.toString() << endl; //Test each of the accessor methods cout << p.getDrawing() << endl; cout << p.getId() << endl; cout << p.getQuantity() << endl; return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
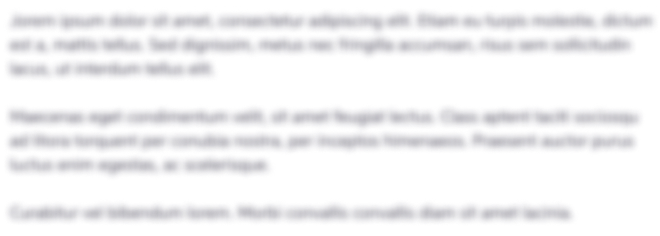
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started