Question
In C++ This is what I have to start, it works bug free. It's a linked list and needs to be modified to be a
In C++
This is what I have to start, it works bug free. It's a linked list and needs to be modified to be a binary search tree type:
Question:
Your assignment is to implement a Binary Search Tree using the employee class you developed in Week 2. The employee class should contain the employee information listed in the input section below. The program should contain all functions listed in the functionality section below
Data about current employees should be on the file "Employee.txt". You will need to create your own employee file and submit it with the final project
Each employee should have the following attributes:
Employee_Number Integer
Employee_Last_Name String
Employee_First_Name String
Employee_Years_of_Service Integer
3 employees must be loaded from the Employee.txt file on program start up
This program should utilize a binary search tree
#include
#include
#include
using namespace std;
struct node
{ //relevant information I need to store
int EmployeeNumber_;
string LastName_;
string FirstName_;
int YearsWorked_;
node *next;
};
class list
{
private:
node * head, *tail; //store heads and tails
public:
list()
{
head = NULL;
tail = NULL;
}
void createnode(int EmployeeNumber, string LastName, string FirstName, int YearsWorked)
{
node *temp = new node; //write these to file
temp->EmployeeNumber_ = EmployeeNumber;
temp->LastName_ = LastName;
temp->FirstName_ = FirstName;
temp->YearsWorked_ = YearsWorked;
temp->next = NULL;
if (head == NULL)
{
head = temp;
tail = temp;
temp = NULL;
}
else
{
tail->next = temp;
tail = temp;
}
}
void display()
{
node *temp = new node;
temp = head;
cout << "EMPLOYEE DATABASE Employee Number\tLast Name\tFirst Name\tYears Worked ";
while (temp != NULL)
{
cout << temp->EmployeeNumber_ << "\t\t" << temp->LastName_ << "\t\t" << temp->FirstName_ << "\t\t" << temp->YearsWorked_ << ' ';
temp = temp->next;
}
cout << " ";
}
void delete_first()
{
node *temp = new node;
temp = head;
head = head->next;
delete temp;
}
void delete_position(int pos)
{
if (pos == 1)
{
delete_first(); //delete first position
return;
}
node *current = new node;
node *previous = new node;
current = head;
for (int i = 1; i { if (current == NULL) return; previous = current; current = current->next; } previous->next = current->next; delete current; } int EmployeeNumber() { int count = 0; node *temp = new node; temp = head; while (temp != NULL) { ++count; temp = temp->next; } return count; } void Start() { int EmployeeNumber; //generic number that user gives Employee, not based on list number string LastName; //of employee string FirstName; int YearsWorked; ifstream FileInput("Employee.txt"); if (FileInput.fail()) { cout << " Cannot Open Employee.txt " << endl; exit(1); } while (true) { FileInput >> EmployeeNumber >> LastName >> FirstName >> YearsWorked; if (FileInput.eof()) break; //if the last of the file is found, break before writing to file createnode(EmployeeNumber, LastName, FirstName, YearsWorked); } FileInput.close(); } void Quit() { ofstream FileOutput; FileOutput.open ("Employee.txt"); node *temp = new node; temp = head; while (temp != NULL) { //saves to employee file FileOutput << temp->EmployeeNumber_ << ' ' << temp->LastName_ << ' ' << temp->FirstName_ << ' ' << temp->YearsWorked_ << ' '; temp = temp->next; } FileOutput.close(); } }; int main() { list obj; obj.Start(); int input = 0; int EmployeeNumber = 0, YearsWorked = 0; string LastName, FirstName; // user menu below cout << "Welcome to the Employee Directory "; while (input != 5) { cout << " Add a user to database enter 1 Remove an employee enter 2 Get employee count enter 3 "; cout << "Print employees enter 4 Quit program enter 5 "; cout << " Enter here: "; int check1 = 1; while (check1 == 1) { cin >> input; if (input > 0 && input < 6) check1 = 0; else cout << "invalid entry, try again :"; } cout << ' '; switch (input) { case 1: { // input and sends info int check = 1; while (check == 1) { cout << "To add an employee to the database enter Employee Number, Last Name, First Name, and years worked "; cin >> EmployeeNumber >> LastName >> FirstName >> YearsWorked; if ((EmployeeNumber > 0 && EmployeeNumber < 9999) && (YearsWorked > 0 && YearsWorked < 99)) { //error check check = 0; } } obj.createnode(EmployeeNumber, LastName, FirstName, YearsWorked); break; } case 2: { int ToDelete; //EmployeeClass.Remove(); //removes user cout << "Enter which employee you'd like to delete from the list "; cin >> ToDelete; obj.delete_position(ToDelete); //delete particular break; } case 3: { cout << "Employees in database: "; cout << obj.EmployeeNumber() << ' '; break; } case 4: { obj.display(); //prints out entire employee list break; } case 5: { obj.Quit(); //saves struct to file and quits break; } } } return 0;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
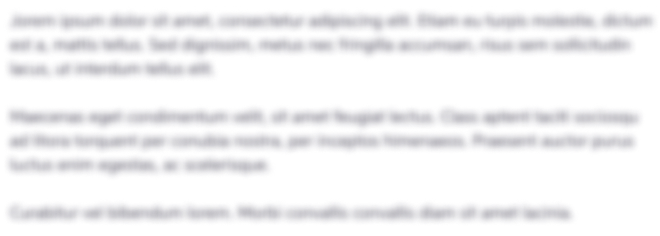
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started