Question
In java Introduction This program will require you to create a program that will allow faculty members to post their course and office hour schedules.
In java
Introduction
This program will require you to create a program that will allow faculty members to post their course and office hour schedules.
Enumeration
First of all, this project utilizes enumerated types which help your programs readability. Here is an example:
public enum DaysOfWeek { SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY; }
The code above would appear in a separate file named DaysOfWeek.java and would serve as the data type for variables that could only have the values: SUNDAY, MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY. Notice, these are not Strings! Internally, SUNDAY=0, MONDAY=1, TUESDAY=2, WEDNESDAY=3, THURSDAY=4, FRIDAY=5, SATURDAY=6. However, the enumerated type is more elegant way of representing these values. The following examples should also prove helpful to you:
DaysOfWeek firstDayOfWeek = DaysOfWeek.SUNDAY;
You can also retrieve the String value of an enumeration. The following example would give the String value the value "SUNDAY":
String value = firstDayOfWeek.name();
UML Class Diagrams
Here are the UML Class Diagrams for the classes you must implement in Java. You are free to add additional private methods if needed.
The method getFormatedTimeBlock() should return a string in the following format:
startTime - endTime comments location (e.g. 1200 - 1300 COMP167 ACB 207 )
The method getCalendar() should return all course items, office hour items and appointment items. Each item (i.e. formatted TimeBlock) should be listed under a heading with the day of the week. Within a particular day, the items should be listed in sorted order by time (Hint: Sorting is not necessary -- use a loop that goes from 5 to 2400 by 5's.).
The toString() method
The toString method should return a String formatted as in the input file. Notice that TimeBlock.toString() will not include the location or comment properties. Most classes will have each property on a new line, TimeBlock properties will be separated by a comma.
Hanlding ArrayLists
Each ArrayList should have five associated methods to perform: getNum, add, get, set and remove. So if you have an ArrayList named widgets that stored items of type Widget, then the associated UML would be:
+getNumWidgets() : int //Return the number of items in the ArrayList widgets. +getWidget(index:int) : Widget //get the Widget at location index in ArrayList widgets +setWidget(index:int, item:Widget):void //store item at location index in the ArrayList widgets. +addWidget(item:Widget):void //Append the Widget to the ArrayList. +removeWidget(index:int):Widget //remove the Widget stored at the given index and return it
Input File
The input file will be supplied to your main() method using command-line arguments. Your labs have covered how to use command-line arguments to pass information to your program. Your main() method should check to see if a file is passed to it. If there is no supplied input file, your program should present the user with the JFileChooser to allow them to select one. The format for the input file is shown below:
Department Name College Name University Name Faculty0 First Name Faculty0 Last Name Faculty0 Office Location Faculty0 Number of Courses Course0 Name Course0 Location Course0 Number of meeting days Course0 Day of the week, Start_Time, End_Time * repeat for other days * repeat for other courses Faculty0 Number of office hour sessions Office Hours0 Day of the week, Start_Time, End_Time * Repeat for other office hours. Faculty0 Number of Appointments Appointments0 Description Appointments0 Day of the week, Start_Time, End_Time * Repeat for other appointments. * Repeat for other faculty The file will end when there are no more faculty.
You should also reference the input file provided in this repository.
You must create a driver program (main() method) for each level that demonstrates that the all the functionality of that level works correctly.
Complete the DaysOfWeek enumerated type, the TimeBlock class, the Course class and the Appointment class. Write a simple driver program that will instantiate an object of each class type, populate the data fields and test the other methods of the classes. Display your output in a JavaFX Dialog.
Complete all of the Faculty class except for the getCalendar() and atAGlance() methods.
Complete all of the Department class except for the loadDepartmentData() and saveDepartmentData() classes.
Implement the loadDepartmentData() and saveDepartmentData() methods. Your main() method should obtain the input file name from the command-line arguments. Also, your main() should prompt the user for an input file name if no command-line argument is passed.
Implement the getCalender() and atAGlance() methods.
departmentData.txt:
Computer Science Department College of Engineering NC A&T State University Kelvin Bryant 303 Cherry Hall 3 GEEN165 Sections 1 & 2 210 Graham Hall 3 Monday, 1100, 1150 Wednesday, 1100, 1150 Friday, 1100, 1150 COMP755 Section 1 129 McNair Hall 2 Monday, 1500, 1615 Wednesday, 1500, 1615 GEEN165 Section 3 210 Graham Hall 3 Monday, 1200, 1250 Wednesday, 1200, 1250 Friday. 1200, 1250 4 Monday, 1330, 1430 Tuesday, 1300, 1600 Wednesday, 1330, 1430 Thursday, 1300, 1600 2 Eat Lunch Monday, 1300, 1330 Eat Lunch Wednesday, 1300, 1330 Kenneth Williams 503 McNair Hall 2 GEEN163 Sections 1 & 2 210 Graham Hall 3 Monday, 900, 950 Wednesday, 900, 950 Friday, 900, 950 GEEN163 Section 3 & 4 210 Graham Hall 3 Monday, 1000, 1050 Wednesday, 1000, 1050 Friday, 1000, 1050 3 Monday, 1200, 1600 Wednesday, 1200, 1400 Friday, 1400, 1700 0
atAGlance needs two parameters: the day and the time. The UML only lists the time. Here is sample output for Monday @ 1305. It was produced by instantiating a Department object, loading the input data and executing:
public static void main(String[] args) {
Department department = new Department()
department.loadDepartmentData(args[0]);
System.out.println(department.atAGlance(DaysOfWeek.Monday, 1305));
}
Faculty At-A-Glance for Monday at 1305
Kelvin Bryant
Eat Lunch 1300 - 1330
Kenneth Williams
Office Hours 1200 - 1600
Here is output for the getCalendar method. The output was produced using:
public static void main(String[] args) {
Department department = new Department();
department.loadDepartmentData(args[0]);
System.out.println(department.getFaculty(0).getCalendar());
}
Calandar for Kelvin Bryant
Sunday
Monday
GEEN165 Sections 1 & 2 1100 - 1150
GEEN165 Section 3 1200 - 1250
Eat Lunch 1300 - 1330
Office Hours 1330 - 1430
COMP755 Section 1 1500 - 1615
Tuesday
Office Hours 1300 - 1600
Wednesday
GEEN165 Sections 1 & 2 1100 - 1150
GEEN165 Section 3 1200 - 1250
Eat Lunch 1300 - 1330
Office Hours 1330 - 1430
COMP755 Section 1 1500 - 1615
Thursday
Office Hours 1300 - 1600
Friday
GEEN165 Sections 1 & 2 1100 - 1150
GEEN165 Section 3 1200 - 1250
Saturday
Here's what I have so far:
package majorprogram1; import java.util.Scanner; import java.util.ArrayList; import javax.swing.JOptionPane; public class MajorProgram1 { /** * @param args the command line arguments */ public static void main(String[] args){ TimeBlock timeb = new TimeBlock(); Appointment ap = new Appointment(); Course course = new Course(); timeb.setDay(DaysOfWeek.SUNDAY); timeb.setStartTime(4); timeb.setEndTime(5); timeb.setLocation("Los Angeles"); timeb.setComments("Sti"); System.out.println(timeb.toString()); System.out.println(timeb.getFormTimeBlock()); ap.setDescription("tall"); ap.setTimeBlock(timeb); System.out.println(ap.toString()); ArrayList
**********************************************************************
package majorprogram1; public enum DaysOfWeek { SUNDAY,MONDAY,TUESDAY,WEDNESDAY,THURSDAY,FRIDAY,SATURDAY; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
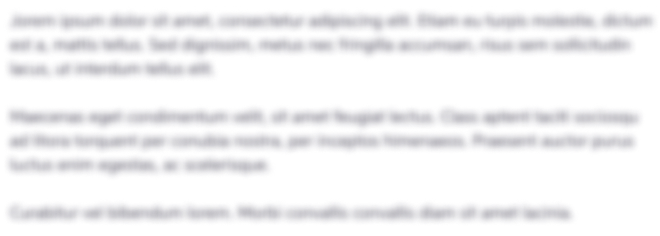
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started