Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In Java Language To begin, visit https://docs.oracle.com/javase/7/docs/api/javax/crypto/package-summary.html for a full documentation of the javax.crypto API classes. The classes that you will need to complete this
In Java Language To begin, visit https://docs.oracle.com/javase/7/docs/api/javax/crypto/package-summary.html for a full documentation of the javax.crypto API classes. The classes that you will need to complete this project are: KeyGenerator and Cipher classes. Provided with this project description a Crypto Java Class Template that has all the methods you need to implement predefined and well documented. You need to complete the implementation of the four methods, run and test your program. Extra Credit (Up to 10 points) - Generate an array of 100 different random strings (50 characters each) - Time how long it takes to encrypt all 100 strings using DES - Time how long it takes to encrypt all 100 strings using AES - Output the time difference between each crypto system import java.util.logging.Level; import java.util.logging.Logger; import javax.crypto.Cipher; import javax.crypto.KeyGenerator; import javax.crypto.SecretKey; import javax.xml.bind.DatatypeConverter; public class Crypto { public static void main(String[] args) { //1. Read input plaintext from user as String //2. Create SecretKey object try { //3. Generate the secret shared key using the getSecretEncryptionKey method //4. Encrypt the plaintext using aesEncryptText method //5. Decrypt the generated cipher using the aesDecryptText method //6. Print the cipher data in Hex Form using the bytexToHex method //7. Print the generated key in Hex Form using the bytesToHex method //8. Print the recovered plaintext in Hex Form using the bytesToHex method } catch (Exception ex) { System.out.println("Exception!"); } } /** * gets the AES encryption key. In real applications, this key should be * safely stored. * @return * @throws Exception */ public static SecretKey getSecretEncryptionKey() throws Exception { //1. Instantiate an object of KeyGenerator class for AES keys //2. Initialize the key to generate 128 bit key //3. Generate the key and stopre it in a SecretKey object //4. return the SecretKey object } /** * Encrypts plainText in AES using the secret key * @param plainText * @param secKey * @return * @throws Exception */ public static byte[] aesEncryptText(String plainText, SecretKey secKey) throws Exception { // AES defaults to AES/ECB/PKCS5Padding in Java 7 //1. Instantiate and object of Class Cipher for AES encryption //2. Initializes the object to ENCYRPT_MODE and the secret key //3. Use the doFinal method of the cipher object to encyprt the plaintext //4. Return the encrypted byte array. } /** * Decrypts encrypted byte array using the key used for encryption. * @param byteCipherText * @param secKey * @return * @throws Exception */ public static String aesDecryptText(byte[] byteCipherText, SecretKey secKey) throws Exception { // AES defaults to AES/ECB/PKCS5Padding in Java 7 //1. Instantiate and object of Class Cipher for AES encryption //2. Initializes the object to DECRYPT_MODE and the secret key //3. Use the doFinal method of the cipher object to Decrypt the plaintext //4. Return the decrypted byte array. } /** * Convert a binary byte array into readable hex form * @param toConvert * @return */ private static String bytesToHex(byte[] toConvert) { return DatatypeConverter.printHexBinary(toConvert); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
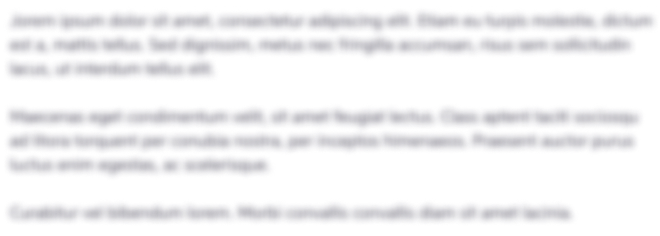
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started