Question
[In Java] Please implement the ByteCode classes according to their descriptions shown in the pictures. Skeleton code is provided. //ByteCode.java package interpreter.ByteCode; public abstract class
[In Java] Please implement the ByteCode classes according to their descriptions shown in the pictures. Skeleton code is provided.
//ByteCode.java
package interpreter.ByteCode;
public abstract class ByteCode { public abstract void init(String param); abstract void execute(); }
//ArgsCode.java, BopCode.java, CallCode.java, DumpCode.java, FalseBranchCode.java, GotoCode.java, HaltCode.java, LabelCode.java, LitCode.java, LoadCode.java, PopCode.java, ReadCode.java, ReturnCode.java, Storecode.java, WriteCode.java
//They all have exact code, except for class name, here is ArgsCode.java for example:
package interpreter.ByteCode;
public class ArgsCode extends ByteCode{ public void init(String param){ } void execute() { } }
//CodeTable.java
/** * Code table of byte codes in language X * @key name of a specific byte code * @value name of the class that the key belongs to. * returns Class name as a string. */ package interpreter;
import java.util.HashMap;
public class CodeTable { private static HashMap codeTable; private CodeTable(){} public static void init(){ codeTable = new HashMap(); codeTable.put("HALT", "HaltCode"); codeTable.put("POP", "PopCode"); codeTable.put("FALSEBRANCH", "FalseBranchCode"); codeTable.put("GOTO", "GotoCode"); codeTable.put("STORE", "StoreCode"); codeTable.put("LOAD", "LoadCode"); codeTable.put("LIT", "LitCode"); codeTable.put("ARGS", "ArgsCode"); codeTable.put("CALL", "CallCode"); codeTable.put("RETURN", "ReturnCode"); codeTable.put("BOP", "BopCode"); codeTable.put("READ", "ReadCode"); codeTable.put("WRITE", "WriteCode"); codeTable.put("LABEL", "LabelCode"); codeTable.put("DUMP", "DumpCode"); }
/** * A method to facilitate the retrieval of the names * of a specific byte code class. * @param key for byte code. * @return class name of desired byte code. */ public static String getClassName(String key){ return codeTable.get(key); } }
//Interpreter.java
package interpreter;
import java.io.*;
/**
*
* Interpreter class runs the interpreter:
* 1. Perform all initializations
* 2. Load the bytecodes from file
* 3. Run the virtual machine
*
*/
public class Interpreter {
private ByteCodeLoader bcl;
public Interpreter(String codeFile) throws InstantiationException, ClassNotFoundException, IllegalAccessException {
try {
CodeTable.init();
bcl = new ByteCodeLoader(codeFile);
} catch (IOException e) {
System.out.println("**** " + e);
}
}
void run() {
Program program = bcl.loadCodes();
VirtualMachine vm = new VirtualMachine(program);
vm.executeProgram();
}
public static void main(String args[]) throws InstantiationException, IllegalAccessException, ClassNotFoundException {
if (args.length == 0) {
System.out.println("***Incorrect usage, try: java interpreter.Interpreter ");
System.exit(1);
}
(new Interpreter(args[0])).run();
}
}
//RunTimeStack.java
package interpreter;
import java.util.ArrayList; import java.util.Stack;
public class RunTimeStack {
private ArrayList runTimeStack; private Stack framePointer;
public RunTimeStack() { runTimeStack = new ArrayList(); framePointer = new Stack(); //Add initial Frame Pointer, main is the entry // point of our language, so its frame pointer is 0. framePointer.add(0); } public void dump(){ //Dump the RunTimeStack information for debugging ArrayList stack = (ArrayList) runTimeStack.clone(); ArrayList framePtrs = (ArrayList) framePointer.clone(); ArrayList frameArray[] = new ArrayList[framePtrs.size()];
for (int i = framePtrs.size(); i > 0; i--) { frameArray[i - 1] = new ArrayList(); int startIndex = framePtrs.get(i - 1); int currentSize = stack.size(); for (int k = startIndex; k
for (int i = 0; i
System.out.print(frameArray[i].toString());
}
System.out.println(); } public int peek(){ //Returns the top item on the runtime stack return (int)runTimeStack.get(runTimeStack.size() - 1); } public int pop(){ //Pop the top item from the runtime stack //Returns that item int top = (int)runTimeStack.get(runTimeStack.size() - 1); runTimeStack.remove(runTimeStack.size() - 1); return top; }
public int push(int i){ //i - push this item on the runtime stack //Returns the item just pushed //runTimeStack.add(framePointer.push(i)); runTimeStack.add(i); return i; }
public int newFrameAt(int offset){ //Start new frame /* offset - indicates the number of slots down from the top of RunTimeStack for starting the new frame */ int size = runTimeStack.size(); framePointer.push(size - offset); return 0; } public void popframe(){ /*We pop the top frame when we return from a function; before popping, the functions return value is at the top of the stack so well save the value, pop the top frame and then push the return value*/ int top = this.pop(); int currentSize = runTimeStack.size(); int startIndex = framePointer.pop(); for (int i = startIndex; i
return top; } public int load(int offset){ /*Used to load variables onto the stack*/ return (int)runTimeStack.get(framePointer.peek() + offset); }
}
//ByteCodeLoader.java
package interpreter;
import interpreter.ByteCode.*; import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException; import java.util.ArrayList; import java.util.StringTokenizer; import java.util.logging.Level; import java.util.logging.Logger;
public class ByteCodeLoader extends Object {
private BufferedReader byteSource; private Program program;
public ByteCodeLoader(String file) throws IOException, InstantiationException, ClassNotFoundException, IllegalAccessException { this.byteSource = new BufferedReader(new FileReader(file)); //Tokenize string to break it into parts. String read = byteSource.readLine(); String[] split = read.split(" "); StringTokenizer token = new StringTokenizer(read); //Create instance from CodeTable String code; ByteCode bytecode; code = interpreter.CodeTable.getClassName(split[0]); bytecode = (ByteCode)(Class.forName("Interpreter."+code).newInstance()); String temp = ""; for(int i = 1; i
//Sample input file Directory is outside of the interpreter package:
//File name: factorial.x.cod
GOTO start> LABEL Read READ RETURN LABEL Write LOAD 0 dummyFormal WRITE RETURN LABEL start> GOTO continue> LABEL factorial> LOAD 0 n LIT 2 BOP > LIT 1 RETURN factorial> POP 0 GOTO continue> LABEL else> LOAD 0 n LOAD 0 n LIT 1 BOP - ARGS 1 CALL factorial> BOP * RETURN factorial> POP 0 LABEL continue> POP 0 LIT 0 GRATIS-RETURN-VALUE RETURN factorial> LABEL continue> ARGS 0 CALL Read ARGS 1 CALL factorial> ARGS 1 CALL Write POP 2 HALT
Bytecode Description HALT POP Examples HALT POP 5 POP 0 FALSEBRANCH xyz3 halt execution POP n: Pop top n levels of runtime stack FALSEBRANCH FALSEBRANCH
Step by Step Solution
There are 3 Steps involved in it
Step: 1
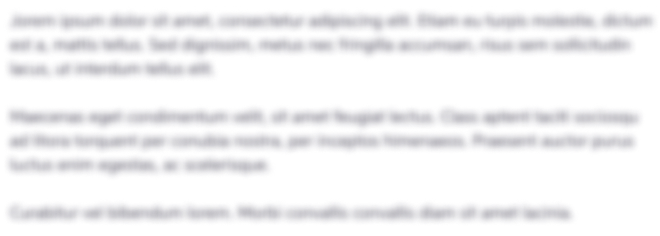
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started