Answered step by step
Verified Expert Solution
Question
1 Approved Answer
In Java please, thank you. Sample output is provided below as well. Write a Train class with one instance variable: an ArrayList of Passenger objects
In Java please, thank you. Sample output is provided below as well.
Write a Train class with one instance variable: an ArrayList of Passenger objects called travelers.
The client and the Passenger classes are fully implemented. You need to implement all the methods that are defined in the Train class.
Train.java // needs to be implemented
/** * Train class * * @author YOUR NAME * @version 11/3/2017 */ import java.util.*; import java.io.*; public class Train { private ArrayList travelers; /** * Default constructor: * 1. Creates this.travelers object * 2. Calls addPassengersFromFile passing * the file name as the parameter */ public Train(String fileName) throws IOException { // TODO // IMPLEMENT THE METHOD } /** * Secondary constructor: * 1. Creates this.travelers object * 2. Calls mutator method setTravelers * * @param newTravelers the ArrayList for travelers defined in the client */ public Train(ArrayList newTravelers) { // TODO // IMPLEMENT THIS METHOD } /** * addPassengers method: * Allows client to initialize the travelers list based on the list of passengers provided in the file. * You can assume that the file has the following format: * * * ... * * Please remember that the file must be placed in the same folder if you are using DrJava * or at the project level if you are using IDEA * * The method * reads from the file one data at a time: which is a String followed by * creates a Passenger object * adds the Passenger object to the travelers list * * Next it reads followed by and creates another * Passenger object and adds it to the travelers list. * * The process continues until there are no more data to be read. * * This method takes one parameter * @param fileName the the name of the passengers text file */ public void addPassengersFromFile(String fileName) throws IOException { System.out.println("*******Reading passengers data from file " + fileName + "*******"); File passengersFile = new File(fileName); Scanner scanFile = new Scanner(passengersFile); String currentName; int currentService; while (scanFile.hasNext()) { // read name of passenger currentName = scanFile.next(); // read service currentService = scanFile.nextInt(); this.travelers.add(new Passenger(currentName, currentService)); } } /** * Mutator method: * * Fills this.travelers with NEW Passenger objects that are copies * of the Passenger objects from newTravelers list: * this.travelers.add( new Passenger( p.getName( ), p.getClassOfService( ) ) ); * @param newTravelers the new ArrayList for travelers */ public void setTravelers(ArrayList newTravelers) { this.travelers.clear(); // TODO // IMPLEMENT THE METHOD } /** accessor method * accessor method that makes a copy of the travelers list and returns it to the caller * IMPORTANT also makes copy of Passenger objects (see setTravelers) * @return the copy of travelers */ public ArrayList getTravelers() { // TODO // IMPLEMENT THE METHOD return null; // THIS IS A STUB } /** * percentageFirstClassPassengers method * Computes the percentage of first class passengers on the train * @return a double, the percentage of first class passengers on the train */ public double percentageFirstClassPassengers() { // TODO // IMPLEMENT THE METHOD return 0; // THIS IS A STUB } /** * trainRevenues method * Computes the total revenues for the train based on first and second class ticket prices * @param firstClassPrice the price of a first class ticket * @param secondClassPrice the price of a second class ticket * @return a double, the total revenues for the train */ public double trainRevenues(double firstClassPrice, double secondClassPrice) { // TODO // IMPLEMENT THE METHOD return 0; // THIS IS A STUB } /** * isOnTrain method * Searches if a person is on the train * @param passengerName the name of a passenger * @return a Passenger if person is on the train, null otherwise */ public Passenger isOnTrain(String passengerName) { // TODO // IMPLEMENT THE METHOD return null; // THIS IS A STUB } /** * setAllPassengersClassOfService method * Checks if all passengers on the train have the given * value of clasOfService. If not changes the value accordingly * @param newClassOfService the value of clasOfService */ public void setAllPassengersClassOfService(int newClassOfService) { // TODO // IMPLEMENT THE METHOD } /** toString * @return elements of travelers one passenger per line * or "The train is empty" message if there are * no passengers on the train */ public String toString() { // TODO // IMPLEMENT THE METHOD return "???"; // THIS IS A STUB } /** equals * @param other Train object * @return return true if elements of ArrayList in other train are equal to * corresponding elements in this object * and ArrayLists have the same size */ public boolean equals(Train other) { // TODO // IMPLEMENT THE METHOD return false; // THIS IS A STUB } }
passengers.txt
Taiowa 2 Andres 2 Brian 2 Alec 2 Jessica 1 Josimar 2 Ferdinand 2 Stephanie 1 Jared 2 Kyle 2 Tiffany 1 Jonathan 2 Katherine 1 Cameron 2 Jaime 2 Daniel 2 Max 2 Jory 2 Samuel 2 Eryk 2 Teagan 1 Anna 1
Passenger.java
/** * Passenger class * * @author Anna Bieszczad * @version 11/3/2017 */ public class Passenger { private String name; private int classOfService; public static final int FIRST_CLASS = 1; public static final int SECOND_CLASS = 2; // the default /** * Overloaded constructor: * Allows client to set beginning values for name and service * This constructor takes two parameters * Calls mutator method to validate new value * * @param name the name of the passenger * @param classOfService the class of travel */ public Passenger(String name, int classOfService) { setName(name); setClassOfService(classOfService); } /** * getName method * * @return the name of the passenger */ public String getName() { return this.name; } /** * getService method * * @return the class of service travel for the passenger */ public int getClassOfService() { return this.classOfService; } /** * Mutator method: * Allows client to set value of name * setName sets the value * in name to newName * * @param newName the new name */ public void setName(String newName) { this.name = newName; } /** * Mutator method: * Allows client to set value of class of service travel * setClassOfService sets the value * in classOfService to newClassOfServiceService * if newClassOfService is not 1 or 2, classOfService is assigned 2 * * @param classOfService the new class of travel */ public void setClassOfService(int classOfService) { // default is SECOND_CLASS if (classOfService == FIRST_CLASS) this.classOfService = classOfService; else this.classOfService = SECOND_CLASS; } /** * toString * * @return a String representation of the passenger */ public String toString() { return ("name: " + this.name + "; class of service: " + this.classOfService); } /** * equals * * @param other Passenger object * @return return true if name and class of travel in other are equal to * corresponding elements in this object */ public boolean equals(Passenger other) { return (this.name.equals(other.name) && this.classOfService == other.classOfService); } }
TrainClient.java
/** * Train client * * @author Anna Bieszczad * @version 11/3/2017 */ import java.util.*; import java.io.*; import java.text.DecimalFormat; public class TrainClient { public static void main(String[] args) throws IOException { System.out.println("******Creating train1*******"); Train train1 = new Train("passengers.txt"); System.out.println(train1.getTravelers().size() + " passengers boarded the train."); System.out.println(" The following passengers are on train1: " + train1); System.out.println("******Creating train2*******"); Train train2 = new Train(new ArrayList()); System.out.println(" The following passengers are on train2: " + train2); System.out.println(" *******Testing the equals method*******"); if (train1.equals(train2)) System.out.println("The trains are equal - INCORRECT"); else System.out.println("The trains are not equal - CORRECT"); ArrayList temp = train1.getTravelers(); train2.setTravelers(temp); if (train1.equals(train2)) System.out.println("After changing train2, the trains are equal - CORRECT"); else System.out.println("After changing train1, the trains are not equal - INCORRECT"); DecimalFormat percentFormat = new DecimalFormat("#0.00%"); System.out.println(percentFormat.format(train1.percentageFirstClassPassengers()) + " of the passengers travel in first class on train1"); System.out.println(" ******Setting all passengers on train2 to ECONOMY_CLASS*******"); train2.setAllPassengersClassOfService(Passenger.SECOND_CLASS); System.out.println(percentFormat.format(train2.percentageFirstClassPassengers()) + " of the passengers travel in first class on train2"); System.out.println(" *******Calculating the revenue*********"); Scanner scanInput = new Scanner(System.in); System.out.print("Enter the ticket price for first class > "); double priceFirstClass = scanInput.nextDouble(); System.out.print("Enter the ticket price for second class > "); double priceSecondClass = scanInput.nextDouble(); DecimalFormat dollarFormat = new DecimalFormat("$##0.00"); System.out.println("Total train1 revenues: " + dollarFormat.format(train1.trainRevenues(priceFirstClass, priceSecondClass))); System.out.println("Total train2 revenues: " + dollarFormat.format(train2.trainRevenues(priceFirstClass, priceSecondClass))); System.out.println(" *******Checking if a passenger is on train1*******"); String person = "Marco"; do { Passenger passenger = train1.isOnTrain(person); if (passenger != null) System.out.println(passenger + " is on the train"); else System.out.println(person + " is not on the train"); System.out.print("Enter the name of a passenger or exit > "); person = scanInput.next(); } while (!person.equalsIgnoreCase("exit")); System.out.println("Good-bye!"); } }
SampleRun.txt
pasaeng data fren ttle paang.txt 22 paeaangs boarded the train. Tbe tolioming paoaangeaaoa trainl: nama: Andrea clasa of srica: 2 ame:Stepbania claas of sarvica:1 riffany nama: Eatherina: clans of 2arica: 1 name:Jaina: a1aaa of aervice: 2 nane: Daniel: clasa of srica: 2 nana: Max: casa of sericR: 2 name: Jory claas of 9arvice: 2 ame: Teagan class ot sevica:2 Tbe tolioming paoaangeaaoa train2 The train ig mptr Tbe traina are not eal- CoRRECT Attez changing train2, the taina ar mal-CORRECT 27% of the paasanger taral n first elaaa aa train! atting all paasangara on train2 to Ecoroes cASS 0ot of tbe paaengaza travel in f1rst laas on train2 calculating tha Enter the ticket peis for second claa 0 Total train2 r:51100.00 Chacking if pa22enge an train. TRA9 12 net an the train Entar the aane of a paasengaz or axit Kyle
Step by Step Solution
There are 3 Steps involved in it
Step: 1
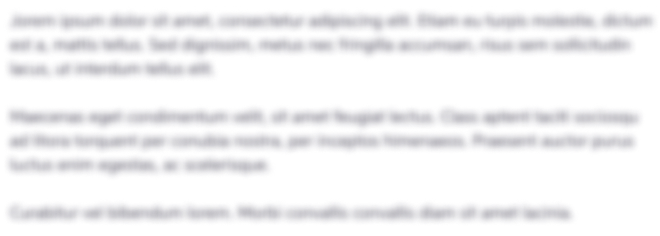
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started